How to Run Batch Script in C#
- Method 1: Using Process.Start
- Method 2: Running Batch Files with Command Prompt
- Method 3: Handling Errors and Output
- Conclusion
- FAQ
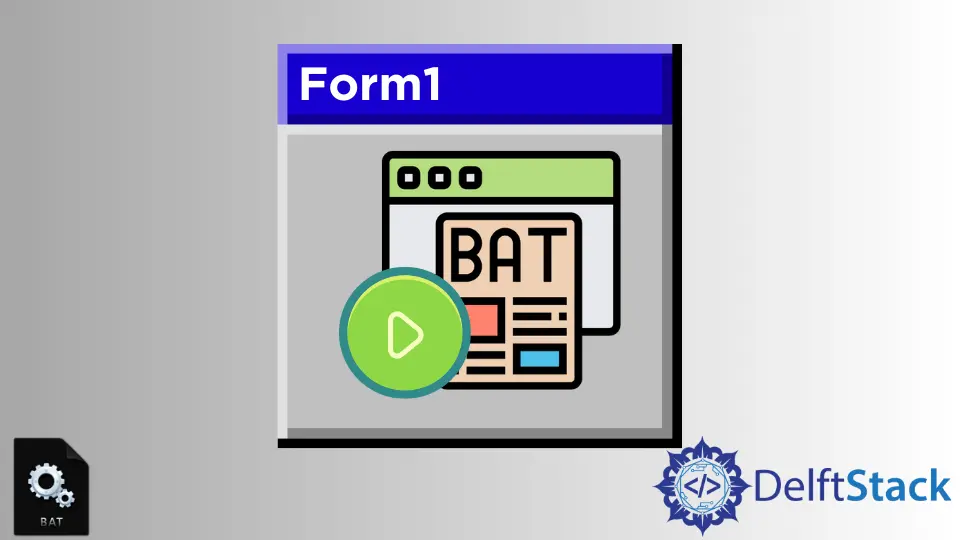
Running batch scripts can be an essential task for developers and system administrators alike. Whether you need to automate repetitive tasks or streamline your workflow, integrating batch scripts with C# can save you a significant amount of time.
In this tutorial, we’ll explore how to run batch scripts using C#. We’ll dive into the different methods available, providing clear code examples and explanations to help you understand each approach. By the end of this guide, you’ll have the knowledge you need to execute batch scripts seamlessly within your C# applications.
Method 1: Using Process.Start
One of the most straightforward ways to run a batch script in C# is by using the Process.Start
method. This method allows you to start a process, in this case, your batch script. Below is a simple example demonstrating how to implement this.
using System.Diagnostics;
class Program
{
static void Main()
{
Process process = new Process();
process.StartInfo.FileName = "your_script.bat";
process.StartInfo.UseShellExecute = false;
process.StartInfo.RedirectStandardOutput = true;
process.StartInfo.RedirectStandardError = true;
process.Start();
string output = process.StandardOutput.ReadToEnd();
string error = process.StandardError.ReadToEnd();
process.WaitForExit();
System.Console.WriteLine(output);
System.Console.WriteLine(error);
}
}
The Process.Start
method enables you to specify various start information about the process. In this example, we set the FileName
property to the name of your batch script. The UseShellExecute
property is set to false
to redirect the output and error streams, which allows us to capture any output from the batch script. After starting the process, we read the standard output and error streams, wait for the process to complete, and finally print the results to the console. This method is efficient and gives you control over the execution flow.
Method 2: Running Batch Files with Command Prompt
Another effective way to run a batch script from C# is by invoking the Command Prompt. This method is particularly useful if you need to pass arguments to your batch script. Here’s how you can do it:
using System.Diagnostics;
class Program
{
static void Main()
{
Process process = new Process();
process.StartInfo.FileName = "cmd.exe";
process.StartInfo.Arguments = "/c your_script.bat arg1 arg2";
process.StartInfo.UseShellExecute = false;
process.StartInfo.RedirectStandardOutput = true;
process.StartInfo.RedirectStandardError = true;
process.Start();
string output = process.StandardOutput.ReadToEnd();
string error = process.StandardError.ReadToEnd();
process.WaitForExit();
System.Console.WriteLine(output);
System.Console.WriteLine(error);
}
}
In this example, we use cmd.exe
to execute the batch script. The /c
argument tells Command Prompt to execute the command that follows and then terminate. You can also pass additional arguments to your batch script, making this method quite flexible. Just like the previous method, we redirect the output and error streams to capture any messages from the batch script. This approach is particularly useful when you need to execute batch scripts that require parameters.
Method 3: Handling Errors and Output
When running batch scripts, it’s crucial to handle potential errors effectively. You can enhance the previous methods by adding error handling and logging the output. Here’s an improved version of the Process.Start
method that includes error handling:
using System;
using System.Diagnostics;
class Program
{
static void Main()
{
Process process = new Process();
process.StartInfo.FileName = "your_script.bat";
process.StartInfo.UseShellExecute = false;
process.StartInfo.RedirectStandardOutput = true;
process.StartInfo.RedirectStandardError = true;
try
{
process.Start();
string output = process.StandardOutput.ReadToEnd();
string error = process.StandardError.ReadToEnd();
process.WaitForExit();
if (!string.IsNullOrEmpty(output))
{
Console.WriteLine("Output:");
Console.WriteLine(output);
}
if (!string.IsNullOrEmpty(error))
{
Console.WriteLine("Error:");
Console.WriteLine(error);
}
}
catch (Exception ex)
{
Console.WriteLine("An error occurred: " + ex.Message);
}
}
}
In this enhanced version, we wrap the process execution in a try-catch
block to catch any exceptions that may occur during execution. This is crucial for debugging and ensures that your application can handle errors gracefully. The output and error messages are printed separately, allowing you to distinguish between successful execution and any issues that arise. This method not only runs the batch script but also provides a more robust framework for error handling.
Conclusion
Running batch scripts in C# can significantly enhance your application’s functionality. By utilizing methods like Process.Start
, you can execute batch files efficiently while managing output and errors effectively. Whether you are automating tasks or integrating existing scripts into your C# applications, the techniques outlined in this tutorial will empower you to streamline your workflow. With practice, you’ll find that combining C# with batch scripting opens up a world of possibilities for automation and efficiency.
FAQ
-
How can I run a batch script from a C# console application?
You can use the Process.Start method to execute a batch script directly from your C# console application. -
Can I pass arguments to my batch script when running it from C#?
Yes, you can pass arguments by using the Command Prompt with the /c option followed by your batch script name and arguments. -
What should I do if my batch script fails to execute?
Ensure that the batch script path is correct and that you have the necessary permissions to execute it. Implement error handling in your C# code to capture any issues. -
Is it possible to capture both output and error messages from the batch script?
Yes, you can redirect both the standard output and standard error streams in your C# code to capture messages from the batch script. -
Can I run multiple batch scripts sequentially in C#?
Absolutely! You can create multiple Process instances and start them one after the other, ensuring to wait for each script to finish before starting the next.
effectively. Learn various methods, including using Process.Start and Command Prompt, to execute batch files seamlessly. Discover how to handle output and errors, making your C# applications more efficient and automated.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn