How to Replace Text From File in Batch Script
-
How to Replace Text From File in Batch Script Using
findstr
andecho
- How to Replace Text From File in Batch Script Using Windows PowerShell
-
How to Replace Text From File in Batch Script Using
sed
- Conclusion
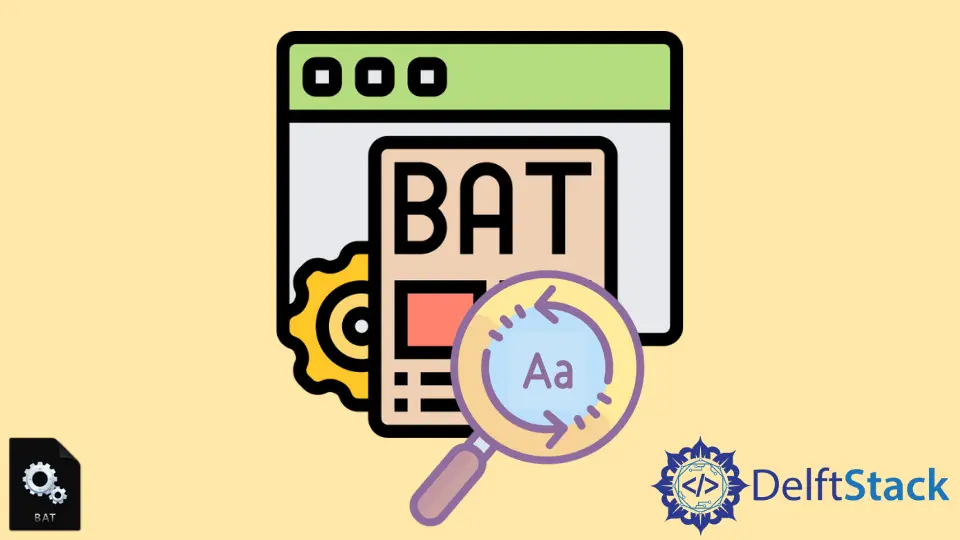
In Batch scripting and automation, the ability to efficiently manipulate text within files is a crucial skill. Whether you’re a seasoned system administrator, a curious enthusiast, or a developer seeking to streamline your workflow, text replacement in Batch files is a valuable asset.
This article delves into three methods to handle string replacement tasks on a Windows system: the findstr
and echo
commands, Windows PowerShell, and the sed
tool.
Text replacement is a common requirement in various scenarios, ranging from modifying configuration files to updating code snippets. Each method discussed here brings its unique strengths to the table, catering to different preferences and situations.
How to Replace Text From File in Batch Script Using findstr
and echo
The findstr
command is a powerful tool for searching for text patterns in files. It supports regular expressions and various search options.
In the following example script, we use findstr
to locate lines containing the text substring we want to replace within the specified file.
On the other hand, the [echo
command]({{relref “/HowTo/Batch/echo command in batch.en.md”}}) is used for displaying messages or, in our case, for outputting modified content to the original file. We leverage the for /f
loop to read each line from the temporary file, replace the desired text, and then echo
the modified line back to the original file.
This approach is particularly useful when you want to perform replacements on a line-by-line basis in a given file.
Sample File: textFile.txt
Let’s consider a sample text file named textFile.txt
with the following content:
Replace String From File Using findstr
and echo
Code Example 1
Now, let’s take a look at the Batch code that replaces the specified text:
@echo off
setlocal enabledelayedexpansion
rem delayed variable expansion, allowing variables to be expanded at execution time.
set "search=sample"
set "replace=modified"
set "inputFile=textFile.txt"
set "outputFile=output.txt"
(for /f "tokens=*" %%a in ('type "%inputFile%" ^| findstr /n "^"') do (
set "line=%%a"
set "line=!line:*:=!"
if defined line (
set "line=!line:%search%=%replace%!"
echo(!line!
) else echo.
)) > "%outputFile%"
endlocal
rem end local scope to clean up environment variable.
Here, we begin by turning off the default echoing of commands with @echo off
, ensuring that only the desired output is displayed. The setlocal enabledelayedexpansion
command is used to enable delayed variable expansion, a crucial feature for working with variables within loops.
Next, we set up variables: search
holds the text we want to find (sample
), replace
contains the replacement text (modified
), inputFile
points to path to the source file (textFile.txt
), and outputFile
designates the path to the file where the modified content will be stored (output.txt
).
The core of the script lies in the for
loop, which iterates through each line of the given file. The type "%inputFile%" ^| findstr /n "^"
command extracts each line, and the for /f "tokens=*" %%a
parses the lines.
Within the loop, the line number is stripped off with set "line=!line:*:=!"
.
Conditional checks follow to ensure that the line is not empty. If a line contains text, the set "line=!line:%search%=%replace%!"
command performs the actual text replacement using the values in the search
and replace
variables.
Finally, the modified line is echoed to the console with echo(!line!
.
The entire loop, the function which handles the replacement and echoing, is encapsulated within parentheses, and the output is redirected to the specified output file (%outputFile%
) using the >
operator.
The script concludes with the endlocal
command, ensuring that the changes in environment variable states are confined to the script’s scope.
Code Output:
Replace String From File Using findstr
and echo
Code Example 2
Let’s enhance the existing script to demonstrate how to replace two or more consecutive words in a text file. Here, we’ll replace the words sample text
with modified content
.
@echo off
setlocal enabledelayedexpansion
rem delayed variable expansion, allowing variables to be expanded at execution time.
set "search=sample text"
set "replace=modified content"
set "inputFile=textFile.txt"
set "outputFile=output.txt"
(for /f "tokens=*" %%a in ('type "%inputFile%" ^| findstr /n "^"') do (
set "line=%%a"
set "line=!line:*:=!"
if defined line (
set "line=!line:%search%=%replace%!"
echo(!line!
) else echo.
)) > "%outputFile%"
endlocal
rem end local scope to clean up environment variable.
In this modified script, the search
variable now contains the phrase sample text
, and the replace
variable holds the replacement modified content
. The script will identify and replace occurrences of sample text
with modified content
throughout the text file.
Code Output:
This demonstrates the flexibility of the script in handling replacements of multiple consecutive words. You can customize the search
and replace
variables to match the specific words or phrases you want to replace in your text file.
How to Replace Text From File in Batch Script Using Windows PowerShell
Another approach we can use to perform text replacement in Batch files is PowerShell—a versatile scripting language that seamlessly integrates with Windows environments. Specifically, we can use PowerShell’s -replace
operator.
The -replace
operator in PowerShell is designed for pattern-based string manipulation. It allows you to specify a search pattern and its replacement within a string.
In the context of Batch Scripting, this operator becomes a valuable asset to enable string substitution feature.
Replace String From File Using Windows PowerShell Code Example
Let’s use the same sample file named textFile.txt
:
@echo off
set "search=sample"
set "replace=modified"
set "inputFile=textFile.txt"
set "outputFile=output.txt"
powershell -Command "(gc %inputFile%) -replace '%search%', '%replace%' | Out-File -encoding ASCII %outputFile%"
In this example, we begin with the directive @echo off
to suppress the echoing of commands. Following that, we set up variables: search
holds the text we want to find (sample
), replace
contains the replacement text (modified
), inputFile
points to the source file (textFile.txt
), and outputFile
designates the file where the modified content or text string will be stored (output.txt
).
The core of the script lies in the powershell -Command
line. Here, we leverage PowerShell’s -replace
operator to perform and execute the text replacement.
The (gc %inputFile%)
part reads the content of the input file, and -replace '%search%', '%replace%'
specifies the search and replacement patterns. The | Out-File -encoding ASCII %outputFile%
section saves the updated content to the specified output file using the PowerShell Out-File
cmdlet.
Code Output:
How to Replace Text From File in Batch Script Using sed
The integration of powerful text processing tools adds another layer of versatility to your toolkit. One such tool is sed
, a stream editor that originated in Unix environments, but thanks to ports like GnuWin32, sed
is available for Windows, providing a command-line utility that can be seamlessly integrated into the command prompt.
sed
stands for stream editor, designed for parsing and transforming text streams. In our context, the Windows port of sed
allows us to perform sophisticated text replacements directly within a Batch file.
This provides a flexible and powerful alternative for scenarios where other native solutions might fall short.
Replace String From File Using sed
Code Example
Let’s continue using our sample file named textFile.txt
:
@echo off
set "search=sample"
set "replace=modified"
set "inputFile=textFile.txt"
set "outputFile=output.txt"
sed "s/%search%/%replace%/g" "%inputFile%" > "%outputFile%"
In this example, we also begin with @echo off
to suppress the echoing of commands. Subsequently, we set up the same variables: search
, replace
, inputFile
, and outputFile
.
The core of the script lies in the sed "s/%search%/%replace%/g" "%inputFile%" > "%outputFile%"
command line call. Here, the sed
command reads the content of the %inputFile%
, searches for occurrences of characters in the specified search
text, and replaces them with the replace
text.
The s/%search%/%replace%/g
syntax defines the search strings and replacement patterns, and the > "%outputFile%"
section directs the modified content to write the specified output file.
Code Output:
This test script effectively replaced the specified text string in the test file with another string, using the Windows port of sed
, demonstrating the versatility of Batch Scripting in handling advanced text processing tasks.
Conclusion
Throughout this article, we’ve delved into three distinct methods, each offering its unique strengths and approaches.
The use of findstr
and echo
commands provides a native and straightforward solution. Its simplicity and reliance on familiar commands make it an accessible choice for those looking to perform text replacements efficiently within a Windows Batch file.
Windows PowerShell, with its -replace
operator, introduces a powerful and dynamic way to manipulate text. Its seamless integration into Batch Scripting allows for intricate replacements and pattern-based transformations, providing a versatile tool for those comfortable with PowerShell’s capabilities.
For users seeking advanced text processing capabilities, the incorporation of sed
proves invaluable. As a Windows port of a Unix-originated stream editor, sed
brings a wealth of functionality for complex text manipulations, expanding the toolkit for seasoned scripters and administrators.
Each method caters to specific needs and preferences, offering flexibility in handling diverse text replacement tasks.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn