C#에서 배치 스크립트 실행
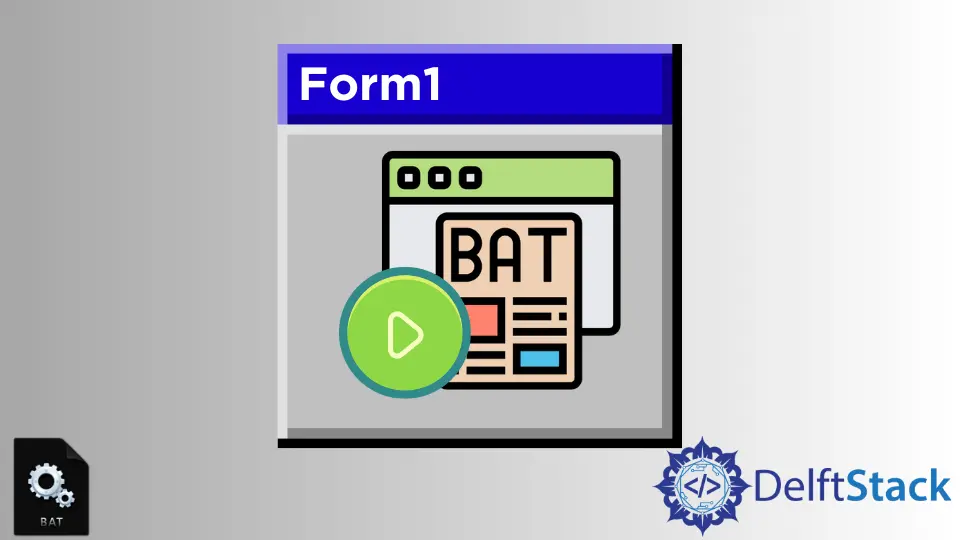
이 기사에서는 디렉토리에서 Batch 파일을 실행할 수 있는 C# 프로그램을 작성하는 방법을 살펴봅니다.
C#
에서 배치 스크립트 실행
C#에서는 Batch 파일을 실행하려고 할 때 프로세스 역할을 합니다. 아래 예제 코드에 따라 C# 프로그램을 사용하여 배치 스크립트를 실행할 수 있습니다.
System.Diagnostics.Process pros = new System.Diagnostics.Process();
pros.StartInfo.FileName = "C:\\MyDir\\simple.bat";
pros.StartInfo.WorkingDirectory = "C:\\MyWorkDir";
pros.Start();
위의 예제 코드에서는 simple.bat
라는 배치 스크립트를 실행합니다. 여기에서 프로세스를 시작하기 전에 작업 디렉토리를 설정해야 합니다.
위의 예는 지정된 디렉토리에서 배치 파일을 실행할 수 있는 코드 조각입니다. 다음 스니펫에서는 동일한 작업을 간단히 수행했습니다.
코드 - C#:
using System;
using System.Collections.Generic;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
using System.Diagnostics;
namespace BatchLoader {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void Button_Click(object sender, EventArgs e) {
// initialize empty process
Process pros = null;
try {
string BatFileDir = string.Format(@"D:\"); // directory of the file
pros = new Process();
pros.StartInfo.WorkingDirectory = BatFileDir;
pros.StartInfo.FileName = "Mybat.bat"; // batch file name to be execute
pros.StartInfo.CreateNoWindow = false;
pros.Start(); // run batch file
pros.WaitForExit();
MessageBox.Show("Batch file successfully executed !!");
} catch (Exception ex) {
Console.WriteLine(ex.StackTrace.ToString());
}
}
}
}
먼저 필요한 모든 패키지를 코드에 초기화합니다. 그런 다음 모든 그래픽 구성 요소를 초기화합니다.
버튼을 통해 배치 파일을 실행하는 액션을 제공합니다. Process pros = null;
줄을 통해 빈 프로세스를 초기화합니다.
런타임 오류를 생성할 수 있으므로 코드의 주요 부분을 예외 처리기에 보관합니다. string BatFileDir = string.Format(@"D:\");
라인을 통해 파일의 디렉토리를 포함하는 문자열을 취합니다.
그런 다음 새 프로세스를 선언하고 BatFileDir
변수를 사용하여 작업 디렉터리를 초기화했습니다. pros.StartInfo.FileName = "Mybat.bat";
줄을 통해 파일 이름을 설정합니다. pros.StartInfo.CreateNoWindow = false;
줄로 새 창 열기를 비활성화합니다.
그런 다음 pros.Start();
줄을 통해 배치 파일을 실행합니다. 라인 pros.WaitForExit();
프로그램이 배치 파일의 실행을 마칠 때까지 기다리게 합니다.
마지막으로 MessageBox.Show("Batch file successful execute !!");
라인을 통해 Batch 파일이 성공적으로 실행되었음을 사용자에게 보여주었습니다.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn