How to Round A Float Number to The Nearest Integer in Arduino
-
Understanding the
round()
Function - Rounding Positive Numbers
- Rounding Negative Numbers
- Handling Decimal Places
- Conclusion
- FAQ
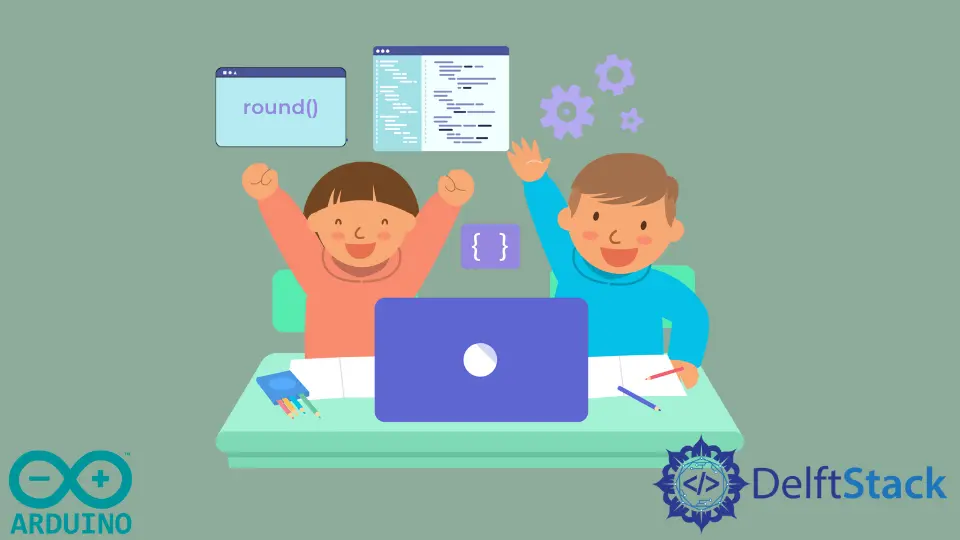
Rounding float numbers to the nearest integer is a common task in programming, especially when working with microcontrollers like Arduino. Whether you’re dealing with sensor readings or calculations, knowing how to effectively round float values can enhance your project’s accuracy and functionality.
In this article, we will explore how to round float numbers to the nearest integer using the built-in round()
function in Arduino. We will provide clear examples and explanations to help you grasp the concept easily. From rounding positive and negative numbers to managing decimal places, you will discover practical techniques to elevate your Arduino programming skills.
Understanding the round()
Function
The round()
function in Arduino is a straightforward way to round a float number to the nearest integer. It takes a single float argument and returns the nearest integer value. If the decimal part is exactly 0.5, the function rounds to the nearest even integer, which is known as “bankers’ rounding.” This behavior is important to understand, especially when handling numerical data that may frequently encounter such scenarios.
Interestingly, if you’re trying to map values that have been rounded, consider looking into the Arduino map() Function for better handling of these rounded integers.
Here’s how you can use the round()
function in your Arduino projects:
float num1 = 3.6;
float num2 = -2.3;
float num3 = 4.5;
int roundedNum1 = round(num1);
int roundedNum2 = round(num2);
int roundedNum3 = round(num3);
In this example, we declare three float variables: num1
, num2
, and num3
. We then use the round()
function to round these numbers to the nearest integers. After executing this code, the variables roundedNum1
, roundedNum2
, and roundedNum3
will hold the rounded integer values.
Output:
roundedNum1: 4
roundedNum2: -2
roundedNum3: 4
The output demonstrates how the function effectively rounds positive and negative float numbers, providing you with integer results that can be used in further calculations or displayed in your project.
Rounding Positive Numbers
When rounding positive float numbers, the round()
function behaves predictably. If the decimal part is less than 0.5, the function rounds down, while if it’s 0.5 or greater, it rounds up. This simple rule makes it easy to manage positive values in your projects.
Consider the following example:
float positiveNum1 = 5.4;
float positiveNum2 = 5.5;
float positiveNum3 = 5.6;
int roundedPositive1 = round(positiveNum1);
int roundedPositive2 = round(positiveNum2);
int roundedPositive3 = round(positiveNum3);
Here, we have three positive float numbers. After rounding, the results will be stored in roundedPositive1
, roundedPositive2
, and roundedPositive3
.
Output:
roundedPositive1: 5
roundedPositive2: 6
roundedPositive3: 6
As expected, 5.4
rounds down to 5
, while both 5.5
and 5.6
round up to 6
. This behavior ensures that you can rely on the round()
function to provide accurate results when working with positive float numbers.
Rounding Negative Numbers
Rounding negative float numbers can be slightly counterintuitive, but the round()
function handles them in the same manner as positive numbers. The key difference is the direction in which the numbers round. For instance, a negative number with a decimal part less than 0.5 will round away from zero.
Let’s look at an example:
float negativeNum1 = -3.4;
float negativeNum2 = -3.5;
float negativeNum3 = -3.6;
int roundedNegative1 = round(negativeNum1);
int roundedNegative2 = round(negativeNum2);
int roundedNegative3 = round(negativeNum3);
In this code, we are rounding three negative float numbers. The results will be stored in roundedNegative1
, roundedNegative2
, and roundedNegative3
.
Output:
roundedNegative1: -3
roundedNegative2: -4
roundedNegative3: -4
As shown, -3.4
rounds to -3
, while both -3.5
and -3.6
round to -4
. Understanding this behavior is crucial for applications where negative values are common, ensuring that your calculations remain accurate and logical.
Handling Decimal Places
Sometimes, you may want to round float numbers that contain more than one decimal place. The round()
function only rounds to the nearest integer, but you can manipulate the float value before applying the function to achieve the desired number of decimal places.
Consider this approach:
float value = 7.4567;
int decimalPlaces = 2;
float factor = pow(10, decimalPlaces);
float roundedValue = round(value * factor) / factor;
In this example, we want to round the float number 7.4567
to two decimal places. We first calculate a factor of 10
raised to the number of decimal places. Then, we multiply the original value by this factor, apply round()
, and finally divide by the factor to get the rounded result.
Output:
roundedValue: 7.46
This method allows you to control the precision of your rounded float values, making it easier to work with measurements or calculations requiring a specific number of decimal places. By adjusting the decimalPlaces
variable, you can customize the rounding behavior to suit your project’s needs.
Conclusion
Rounding float numbers to the nearest integer in Arduino is a simple yet powerful technique that can significantly improve your programming projects. By utilizing the round()
function, you can effectively manage both positive and negative float values, as well as control the precision of your results. With the examples and explanations provided in this article, you should now have a solid understanding of how to implement rounding in your Arduino code. So go ahead and apply these techniques in your projects to enhance their accuracy and functionality!
FAQ
-
How does the
round()
function work in Arduino?
theround()
function rounds a float number to the nearest integer, following standard rounding rules. -
Can I round float numbers with more than one decimal place?
Yes, you can manipulate the float value before rounding to achieve the desired precision. -
What happens if the decimal part is exactly 0.5?
theround()
function rounds to the nearest even integer, a method known as bankers’ rounding. -
Is there a way to round down or up specifically?
Yes, you can use floor() to round down and ceil() to round up in Arduino. -
Can I use the
round()
function with negative numbers?
Yes, theround()
function works with negative numbers, rounding away from zero.