Arduino map() Function
- What is the Arduino map() Function?
-
How to Use the
map()
Function in Arduino -
Practical Applications of the
map()
Function -
Common Mistakes When Using the
map()
Function - Conclusion
- FAQ
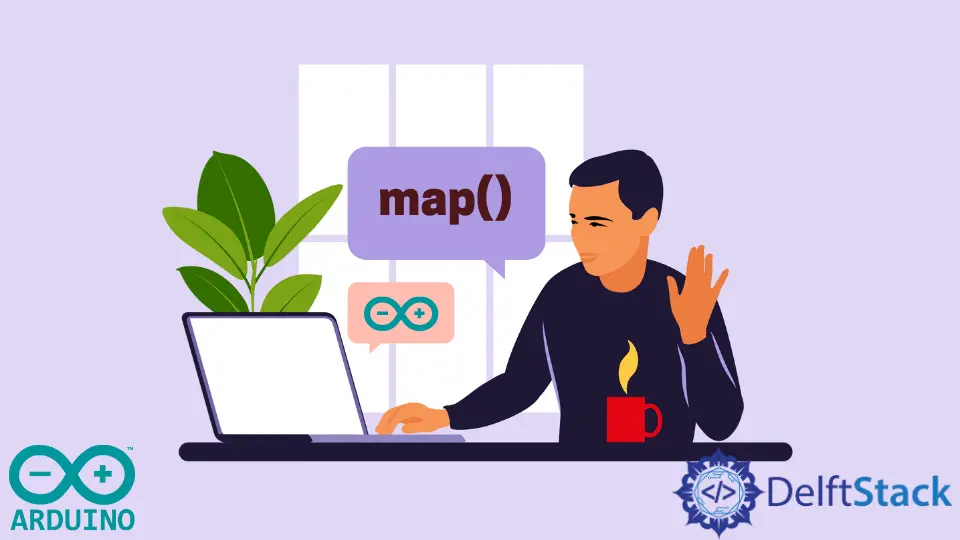
The Arduino map()
function is a powerful tool that allows developers and hobbyists to transform a number from one range to another seamlessly. Whether you’re working on a project that involves sensors, motors, or any other components requiring precise numerical adjustments, the map()
function can simplify your calculations. It takes an input value and maps it from a defined input range to a specified output range. This functionality is crucial for tasks like scaling sensor values or adjusting motor speed based on varying input conditions.
In this article, we will dive deep into the workings of the map()
function, its syntax, and practical applications, ensuring you have a solid understanding of how to leverage this feature in your Arduino projects.
What is the Arduino map() Function?
The map()
function is a built-in function in the Arduino programming language that translates a number from one range to another. Its syntax is straightforward:
long map(long x, long in_min, long in_max, long out_min, long out_max)
x
: The number you want to map.in_min
: The lower bound of the input range.in_max
: The upper bound of the input range.out_min
: The lower bound of the output range.out_max
: The upper bound of the output range.
This function is particularly useful in scenarios where you need to convert sensor readings or scale values for display or control purposes. For further understanding of related concepts, you might want to learn how to get the Arduino length of an array using the sizeof()
function.
How to Use the map()
Function in Arduino
To illustrate how the map()
function works, let’s consider a practical example. Suppose you have a temperature sensor that outputs values between 0 and 100 degrees Celsius, and you want to display these values on a scale from 0 to 255, which is commonly used in RGB LED brightness control. Here’s how you would implement this:
int sensorValue = analogRead(A0);
int mappedValue = map(sensorValue, 0, 100, 0, 255);
In this code snippet, analogRead(A0)
retrieves the sensor value, which is then mapped from the range of 0-100 to the range of 0-255. The result is stored in mappedValue
, which can then be used to control an LED or any other output device.
Output:
mappedValue will be a value between 0 and 255
This example demonstrates the versatility of the map()
function. By adjusting the input and output ranges, you can easily adapt it to different applications, making it a fundamental tool in your Arduino programming toolkit.
Practical Applications of the map()
Function
The map()
function finds its utility in various practical applications. One common use case is in robotics, where you might need to control motor speed based on sensor input. For instance, if your robot’s speed sensor outputs values from 0 to 1023, and you want to map these to a PWM signal range of 0 to 255, you would use the map()
function as follows:
int sensorSpeed = analogRead(A1);
int motorSpeed = map(sensorSpeed, 0, 1023, 0, 255);
analogWrite(motorPin, motorSpeed);
In this example, sensorSpeed
reads the current speed from a sensor. The map()
function translates this value into a suitable PWM signal for the motor, allowing for smooth control of the robot’s speed.
Output:
motorSpeed will be a value between 0 and 255
This capability is crucial for ensuring that your robot operates smoothly and responsively. The map()
function helps bridge the gap between raw sensor data and actionable control signals, making it an invaluable asset in any Arduino project.
Common Mistakes When Using the map()
Function
While the map()
function is a powerful tool, there are common pitfalls that users should be aware of. One frequent mistake is not understanding the implications of integer division in C++. If you are working with floating-point numbers, the output may not be what you expect. You might find it useful to understand how to round a float number to the nearest integer in Arduino to maintain precision.
For example, if you mistakenly use integers in your calculations, you could end up with truncated values. To avoid this, ensure your input and output ranges are defined correctly, and consider casting your values to float
if necessary.
float sensorValue = analogRead(A0);
float mappedValue = map(sensorValue, 0, 100, 0, 255);
In this case, using float
ensures that you maintain precision in your calculations.
Output:
mappedValue will now retain decimal values
By being mindful of these common mistakes, you can effectively utilize the map()
function in your projects without running into unexpected issues.
Conclusion
The Arduino map()
function is an essential tool for anyone looking to manipulate numerical data within their projects. Its ability to transform a number from one range to another makes it invaluable for applications in robotics, sensor readings, and control systems. By understanding its syntax and practical applications, you can enhance the functionality of your Arduino projects significantly. As you continue to explore the possibilities with Arduino, remember that mastering the map()
function will empower you to create more dynamic and responsive systems.
FAQ
-
What is the purpose of the Arduino map() function?
themap()
function is used to convert a number from one range to another, making it easier to work with different scales in your projects. -
Can I use the
map()
function with floating-point numbers?
Yes, you can use themap()
function with floating-point numbers, but it’s essential to ensure that you maintain precision in your calculations. -
What happens if I use incorrect input or output ranges?
Using incorrect ranges can lead to unexpected results, such as values being mapped outside the desired bounds. -
Is the
map()
function specific to Arduino?
While themap()
function is commonly associated with Arduino, similar functionality can be found in other programming environments, but the syntax may vary. -
How can I improve the accuracy of the mapped values?
To improve accuracy, ensure that your input and output ranges are well-defined and consider using floating-point arithmetic when necessary.