Arduino Natural Log
-
Understanding the
log()
Function - Basic Example of Using log() in Arduino
-
Error Handling with the
log()
Function -
Advanced Applications of the
log()
Function - Conclusion
- FAQ
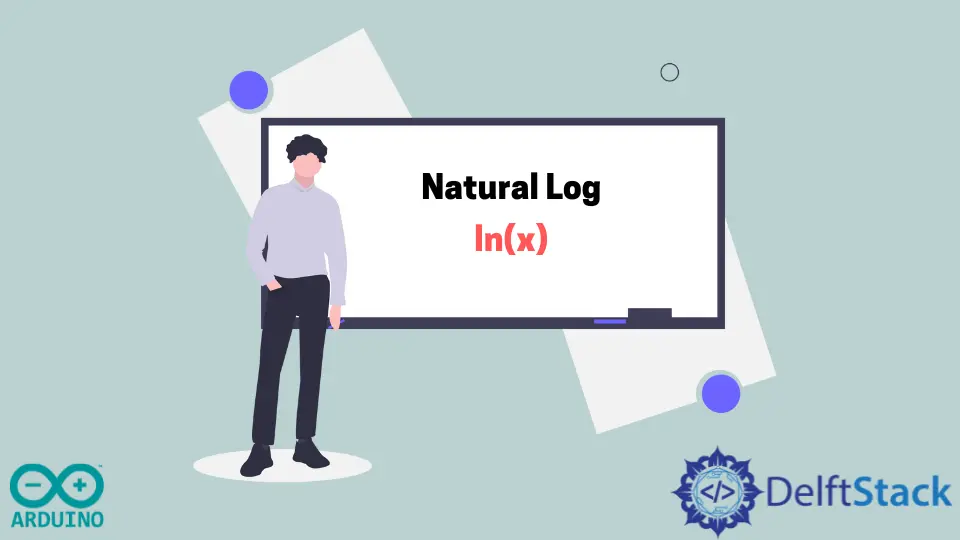
Exploring the world of Arduino programming can be both exciting and challenging, especially when diving into mathematical functions like the natural logarithm.
In this article, we will take a close look at the Arduino natural log function, specifically the log()
function. Whether you’re a beginner or an experienced programmer, understanding how to effectively use this function can enhance your projects and improve your programming skills. We’ll cover practical applications, error handling techniques, and provide real code examples to illustrate how to implement the log()
function in your Arduino projects. By the end, you’ll have a solid grasp of logarithmic calculations and how to apply them in various scenarios.
Understanding the log()
Function
the log()
function in Arduino is a built-in mathematical function that calculates the natural logarithm (base e) of a given number. This function is particularly useful in various applications, such as signal processing, data analysis, and scientific computations. The syntax for the log()
function is straightforward:
float log(float x);
The function takes a single argument, x, which must be a positive number. If x is less than or equal to zero, the function will return NaN (Not a Number). This is crucial to remember, as it can lead to errors in your calculations if not handled properly.
Practical Applications of the log()
Function
The natural logarithm has numerous applications in the field of electronics and programming. Here are a few examples:
- Signal Processing: In audio processing, logarithmic scales are often used to represent sound intensity levels, making the
log()
function essential for audio applications. - Data Analysis: Logarithmic transformations can help in normalizing data that spans several orders of magnitude, making it easier to analyze and visualize.
- Scientific Calculations: Many scientific formulas involve natural logarithms, especially in fields like physics and chemistry.
Understanding how to implement the log()
function can significantly enhance your Arduino projects, allowing you to tackle more complex calculations with ease.
Basic Example of Using log() in Arduino
Now, let’s look at a basic example of how to use the log()
function in an Arduino sketch. This example will demonstrate how to calculate the natural logarithm of a number and print the result to the Serial Monitor.
void setup() {
Serial.begin(9600);
float number = 10.0;
float result = log(number);
Serial.print("The natural log of ");
Serial.print(number);
Serial.print(" is: ");
Serial.println(result);
}
void loop() {
}
In this code snippet, we first initialize the Serial communication at a baud rate of 9600. We then define a floating-point variable named number and assign it a value of 10. the log()
function is called with this number, and the result is stored in the variable result. Finally, we print both the original number and its natural logarithm to the Serial Monitor.
Output:
The natural log of 10.0 is: 2.302585
This simple example showcases how to use the log()
function effectively. By understanding the output, you can see how the natural logarithm provides valuable information about the input value.
Error Handling with the log()
Function
When using the log()
function, it’s essential to handle potential errors, especially when dealing with user input or dynamic data. Since the log()
function only accepts positive values, we need to ensure that our input meets this requirement. Here’s a code example that demonstrates error handling:
void setup() {
Serial.begin(9600);
float number = -5.0; // Example of an invalid input
if (number > 0) {
float result = log(number);
Serial.print("The natural log of ");
Serial.print(number);
Serial.print(" is: ");
Serial.println(result);
} else {
Serial.print("Error: Input must be a positive number.");
}
}
void loop() {
}
In this example, we check if the variable number is greater than zero before calling the log()
function. If the input is invalid, an error message is printed to the Serial Monitor instead. This approach helps prevent unexpected behavior in your program and ensures that your calculations remain accurate.
Output:
Error: Input must be a positive number.
Implementing error handling is a critical aspect of programming. It not only improves the robustness of your code but also enhances the user experience by providing clear feedback on input errors.
Advanced Applications of the log()
Function
Once you have a solid understanding of the basic usage and error handling of the log()
function, you can explore more advanced applications. For instance, you might want to use the natural logarithm in a mathematical model or a sensor calibration process. Here’s an example that demonstrates how to use the log()
function to calculate the decay of a substance over time.
void setup() {
Serial.begin(9600);
float initialAmount = 100.0; // Initial quantity of the substance
float halfLife = 5.0; // Half-life in time units
float timeElapsed = 10.0; // Time elapsed
float remainingAmount = initialAmount * exp(-log(2) / halfLife * timeElapsed);
Serial.print("Remaining amount after ");
Serial.print(timeElapsed);
Serial.print(" time units is: ");
Serial.println(remainingAmount);
}
void loop() {
}
In this code, we calculate the remaining amount of a substance after a certain period using the natural logarithm. The formula used combines the log()
function with an exponential decay formula. By adjusting the initial amount, half-life, and time elapsed, you can model various decay scenarios.
Output:
Remaining amount after 10.0 time units is: 25.0
This example illustrates how the log()
function can be applied in practical scenarios, enabling you to create more sophisticated Arduino projects that incorporate mathematical modeling.
Conclusion
In this comprehensive guide, we’ve explored the Arduino natural log function and its applications. From understanding the log()
function to implementing error handling and advanced applications, you now have the tools to enhance your Arduino programming skills. Whether you’re working on signal processing, data analysis, or scientific computations, the log()
function is a valuable asset in your programming toolkit. Keep experimenting with this function and apply it to your projects to unlock new possibilities in your Arduino journey.
FAQ
-
What is the
log()
function in Arduino?
thelog()
function calculates the natural logarithm (base e) of a given positive number. -
Can I use log() with negative numbers?
No, thelog()
function only accepts positive values. If you input a negative number or zero, it will return NaN. -
How can I handle errors when using the
log()
function?
You can check if the input is positive before calling thelog()
function and provide feedback if the input is invalid. -
What are some practical applications of the
log()
function?
thelog()
function is used in signal processing, data normalization, and scientific calculations among others. -
Can I combine log() with other mathematical functions?
Yes, you can combine thelog()
function with other mathematical functions to create complex calculations in your Arduino projects.