How to Parse JSON in Angular
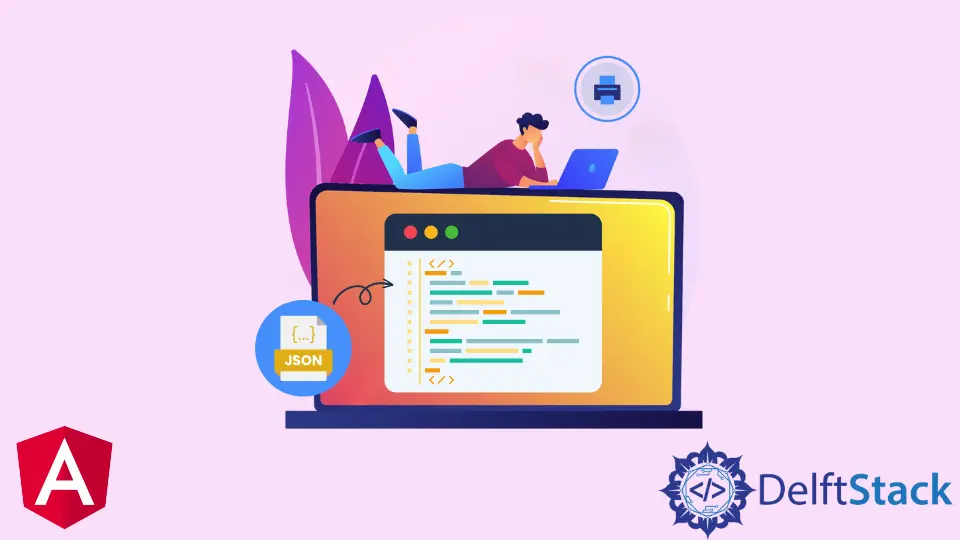
This article will tackle how to parse JSON in Angular with examples.
Parse JSON in Angular
When working with APIs in Angular applications, we come across responses in JSON. We may be getting some data from the API in JSON format and may need to display it on a table.
For this, we need to parse the JSON data. Let’s have an example and try to create JSON data using the stringify
method of JSON.
This method is used to convert anything to JSON format. Then we can use that JSON to parse with the help of another method of JSON parse()
.
The parse()
method can parse JSON without any trouble.
Example Code:
# Angular
import { Component, VERSION, OnInit } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
})
export class AppComponent implements OnInit {
strInfo: any;
parseInfo: any;
myInfo =
{
"id": 0,
"guid": "9b06a996-23f4-46ff-a007-a6bca1f9c1f3",
"balance": "$1,590.37",
"age": 33,
"name": "Chaney Harrell",
"gender": "male",
"company": "GOGOL",
"email": "chaneyharrell@gogol.com",
"phone": "+1 (836) 566-3872",
"address": "716 Grafton Street, Rodanthe, West Virginia, 6757"
}
ngOnInit() {
console.log(this.myInfo);
this.strInfo = JSON.stringify(this.myInfo);
console.log('After using Stringify :', this.strInfo);
this.parseInfo = JSON.parse(this.strInfo);
console.log('After Parsing Json Info:', this.parseInfo);
}
}
Output:
With the help of stringify()
, we can convert the data into a perfect JSON, and then we can use another function of JSON parse()
that can parse the JSON data without any trouble.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn