How to Convert XML to JSON in Angular
- The XML Format (Extensible Markup Language)
- The JSON Format (JavaScript Object Notation)
- Convert XML to JSON in Angular
- Properties of XML and JSON Format
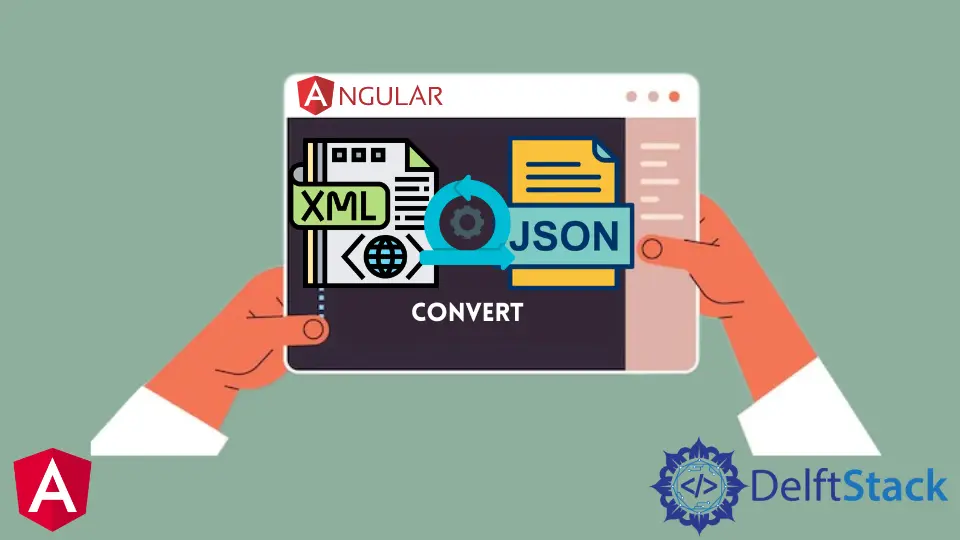
If you want to convert your Angular XML to JSON, there are two ways to do it: using the built-in Angular function or using an external library like xml2js
.
In this article, we will provide the steps to convert XML to JSON with the help of xml2js
. But before moving into the conversion section, let us discuss what XML and JSON are.
The XML Format (Extensible Markup Language)
XML stands for Extensible Markup Language. It is a markup language designed to describe data structure machine-readable way.
XML was initially developed to share data between different software and systems without losing information or formatting, relying on proprietary formats, and requiring special tools for processing. It organizes data systematically, similar to a hierarchical tree, to minimize intricacy and make it easier to comprehend.
It saves the information it receives, making it heavier and slower. When transferring data, you’ll require a fast transmission speed.
The JSON Format (JavaScript Object Notation)
JSON stands for JavaScript Object Notation. It is a lightweight data-interchange format.
JSON is an open-standard file format that employs human-readable text to communicate data objects of attribute-value pairs and arrays (or any other serializable value).
JSON is predominantly used to transfer data between a server and web application as an alternative to XML. The JSON format was initially designed to be easy for humans to read and write while fast enough to convert the data into an efficient storage system.
Convert XML to JSON in Angular
Below are the steps for converting XML to JSON with the help of xml2js
.
-
Install
xml2js
.npm install xml2js
-
Load the XML file which needs conversion into the browser console using below.
```typescript
('xml2json').parse(filePath)
```
-
Convert the loaded XML file into a string of JavaScript Object Notation (JSON) by using the following code.
var obj = require('xml2json').parse(filePath); console.log(obj);
Example:
TypeScript code:
import { Component, OnInit } from '@angular/core';
import { NgxXml2jsonService } from 'ngx-xml2json';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit {
name = 'Angular';
objs: any = {};
xml = ` <inform lastUpdated="2022-12-18T05:18:43">
<NameInfo>
<Name type="NAICS" order="1" reported="0" mnem="">Steve</Name>
<Name type="NAICS" order="2" reported="0" mnem="">Adil</Name>
</inform>`;
constructor(private ngxXml2jsonService: NgxXml2jsonService) {
}
ngOnInit() {
const parser = new DOMParser();
const xml = parser.parseFromString(this.xml, 'text/xml');
const obj = this.ngxXml2jsonService.xmlToJson(xml);
this.objs = obj
console.log(this.objs);
}
}
HTML code:
<h2>Example of XML to JSON conversion in Angular</h2>
<ul>
<li *ngFor="let data of objs?.inform?.NameInfo?.Name">{{data}}</li>
</ul>
Click here to check the working of the code above.
Properties of XML and JSON Format
XML and JSON are two different formats of data interchange, each having its pros and cons. XML offers more flexibility in structure and content, but it’s harder to work with because it’s not as human-readable as JSON.
On the other hand, JSON is lighter than XML because it doesn’t use any tags or formatting, but it can take up more bandwidth than XML because it needs to include a lot of quotes around strings or numbers.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook