How to Use Google Maps in Angular
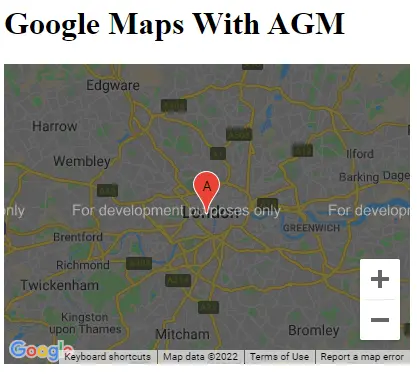
We will introduce Google Maps and use them on our Angular applications.
Google Maps in Angular
Google Maps are the easiest way to help users find your locations without leaving your website to improve user experience.
In this tutorial, a few libraries help us integrate Google Maps into our applications, discussing the Angular Google Maps
library.
Let’s understand it by implementing Google Maps in one of our applications. So to create a new Angular application, we will do the following command in the terminal.
# Terminal
ng new my-app
cd my-app
This command will create a new Angular project and take us into the my-app
project directory.
Now, we will import the Angular Google Maps
library using CLI.
# angular
npm install @agm/core
After installing Angular Google Maps
library, we need to import it in our app.module.ts
file.
# angular
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { FormsModule } from '@angular/forms';
import { AppComponent } from './app.component';
import { HelloComponent } from './hello.component';
import { AgmCoreModule } from '@agm/core';
@NgModule({
imports: [
BrowserModule,
FormsModule,
AgmCoreModule.forRoot({
apiKey: 'xxx'
})
],
declarations: [ AppComponent, HelloComponent ],
bootstrap: [ AppComponent ]
})
export class AppModule { }
Now, in the app.component.ts
file, we will define the initial position of the map that will show when the page is loaded by giving values of longitude and latitude. And we will also make a marker to a random place.
For setting a marker, we need three parameters, longitude, latitude, and label of that marker. In the end, we will define an interface so that we always place the correct type of values in these parameters. Our code in app.component.ts
will look like this.
# angular
import { Component } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
})
export class AppComponent {
latitude: number = 51.5055676;
longitude: number = -0.1326702;
markers: marker[] = [
{
latitude: 51.5055676,
longitude: -0.1326702,
label: 'A',
},
];
}
interface marker {
latitude: number;
longitude: number;
label?: string;
}
Now, let’s create a template to display our map with our settings. We can also allow zoom controls using [zoomControl]
.
# angular
<h1>Google Maps With AGM</h1>
<agm-map
[latitude]="latitude"
[longitude]="longitude"
[disableDefaultUI]="false"
[zoomControl]="true">
<agm-marker
*ngFor="let a of markers; let b = index"
[latitude]="a.latitude"
[longitude]="a.longitude"
[label]="a.label">
<agm-info-window>
<strong>Info Window! This is a random location</strong>
</agm-info-window>
</agm-marker>
</agm-map>
Output:
We can easily display any map with multiple markers on it in this easy way.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn