Angular 中的谷歌地图
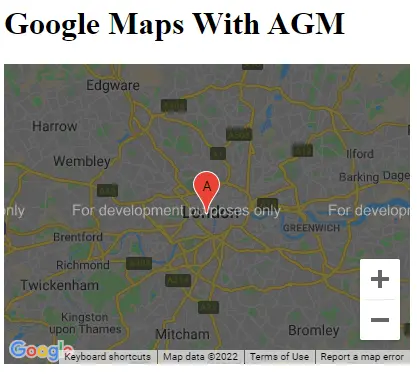
我们将介绍 Google 地图并将它们用于我们的 Angular 应用程序。
Angular 中的谷歌地图
Google 地图是帮助用户在不离开你的网站的情况下找到你的位置以改善用户体验的最简单方法。
在本教程中,一些库帮助我们将 Google 地图集成到我们的应用程序中,讨论了 Angular Google Maps
库。
让我们通过在我们的一个应用程序中实现谷歌地图来理解它。因此,要创建一个新的 Angular 应用程序,我们将在终端中执行以下命令。
# Terminal
ng new my-app
cd my-app
该命令将创建一个新的 Angular 项目并将我们带到 my-app
项目目录。
现在,我们将使用 CLI 导入 Angular Google Maps
库。
# angular
npm install @agm/core
安装 Angular Google Maps
库后,我们需要将其导入 app.module.ts
文件中。
# angular
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { FormsModule } from '@angular/forms';
import { AppComponent } from './app.component';
import { HelloComponent } from './hello.component';
import { AgmCoreModule } from '@agm/core';
@NgModule({
imports: [
BrowserModule,
FormsModule,
AgmCoreModule.forRoot({
apiKey: 'xxx'
})
],
declarations: [ AppComponent, HelloComponent ],
bootstrap: [ AppComponent ]
})
export class AppModule { }
现在,在 app.component.ts
文件中,我们将通过提供经度和纬度值来定义地图的初始位置,该位置将在页面加载时显示。我们还会在一个随机的地方做一个标记。
为了设置标记,我们需要三个参数,该标记的经度、纬度和标签。最后,我们将定义一个接口,以便我们始终在这些参数中放置正确类型的值。我们在 app.component.ts
中的代码将如下所示。
# angular
import { Component } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
})
export class AppComponent {
latitude: number = 51.5055676;
longitude: number = -0.1326702;
markers: marker[] = [
{
latitude: 51.5055676,
longitude: -0.1326702,
label: 'A',
},
];
}
interface marker {
latitude: number;
longitude: number;
label?: string;
}
现在,让我们创建一个模板来显示我们的地图和我们的设置。我们还可以使用 [zoomControl]
进行缩放控制。
# angular
<h1>Google Maps With AGM</h1>
<agm-map
[latitude]="latitude"
[longitude]="longitude"
[disableDefaultUI]="false"
[zoomControl]="true">
<agm-marker
*ngFor="let a of markers; let b = index"
[latitude]="a.latitude"
[longitude]="a.longitude"
[label]="a.label">
<agm-info-window>
<strong>Info Window! This is a random location</strong>
</agm-info-window>
</agm-marker>
</agm-map>
输出:
我们可以通过这种简单的方式轻松地显示任何带有多个标记的地图。
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn