Angular의 Google 지도
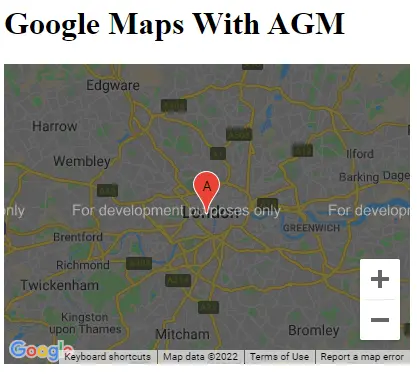
Google 지도를 소개하고 Angular 애플리케이션에서 사용할 것입니다.
Angular의 Google 지도
Google 지도는 사용자 경험을 개선하기 위해 웹사이트를 떠나지 않고도 사용자가 위치를 찾을 수 있도록 도와주는 가장 쉬운 방법입니다.
이 튜토리얼에서는 Angular Google Maps
라이브러리에 대해 논의하면서 Google Maps를 애플리케이션에 통합하는 데 도움이 되는 몇 가지 라이브러리를 제공합니다.
우리 애플리케이션 중 하나에서 Google 지도를 구현하여 이해해 봅시다. 따라서 새 Angular 응용 프로그램을 만들기 위해 터미널에서 다음 명령을 수행합니다.
# Terminal
ng new my-app
cd my-app
이 명령은 새 Angular 프로젝트를 만들고 my-app
프로젝트 디렉토리로 이동합니다.
이제 CLI를 사용하여 Angular Google Maps
라이브러리를 가져옵니다.
# angular
npm install @agm/core
Angular Google Maps
라이브러리를 설치한 후 app.module.ts
파일로 가져와야 합니다.
# angular
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { FormsModule } from '@angular/forms';
import { AppComponent } from './app.component';
import { HelloComponent } from './hello.component';
import { AgmCoreModule } from '@agm/core';
@NgModule({
imports: [
BrowserModule,
FormsModule,
AgmCoreModule.forRoot({
apiKey: 'xxx'
})
],
declarations: [ AppComponent, HelloComponent ],
bootstrap: [ AppComponent ]
})
export class AppModule { }
이제 app.component.ts
파일에서 경도와 위도 값을 제공하여 페이지가 로드될 때 표시될 지도의 초기 위치를 정의합니다. 그리고 우리는 또한 임의의 장소에 마커를 만들 것입니다.
마커를 설정하려면 해당 마커의 경도, 위도 및 레이블의 세 가지 매개변수가 필요합니다. 결국 우리는 이러한 매개변수에 항상 올바른 유형의 값을 배치하도록 인터페이스를 정의합니다. app.component.ts
의 코드는 다음과 같습니다.
# angular
import { Component } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
})
export class AppComponent {
latitude: number = 51.5055676;
longitude: number = -0.1326702;
markers: marker[] = [
{
latitude: 51.5055676,
longitude: -0.1326702,
label: 'A',
},
];
}
interface marker {
latitude: number;
longitude: number;
label?: string;
}
이제 설정으로 지도를 표시할 템플릿을 만들어 보겠습니다. [zoomControl]
을 사용하여 확대/축소 제어를 허용할 수도 있습니다.
# angular
<h1>Google Maps With AGM</h1>
<agm-map
[latitude]="latitude"
[longitude]="longitude"
[disableDefaultUI]="false"
[zoomControl]="true">
<agm-marker
*ngFor="let a of markers; let b = index"
[latitude]="a.latitude"
[longitude]="a.longitude"
[label]="a.label">
<agm-info-window>
<strong>Info Window! This is a random location</strong>
</agm-info-window>
</agm-marker>
</agm-map>
출력:
이 쉬운 방법으로 여러 마커가 있는 모든 지도를 쉽게 표시할 수 있습니다.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn