How to Find Elements by Class Name in Angular
-
Find Elements by Class Name in Angular Using
ViewChild
WithElementRef
-
Find Elements by Class Name in Angular Using the
ElementRef
andRenderer2
- Conclusion
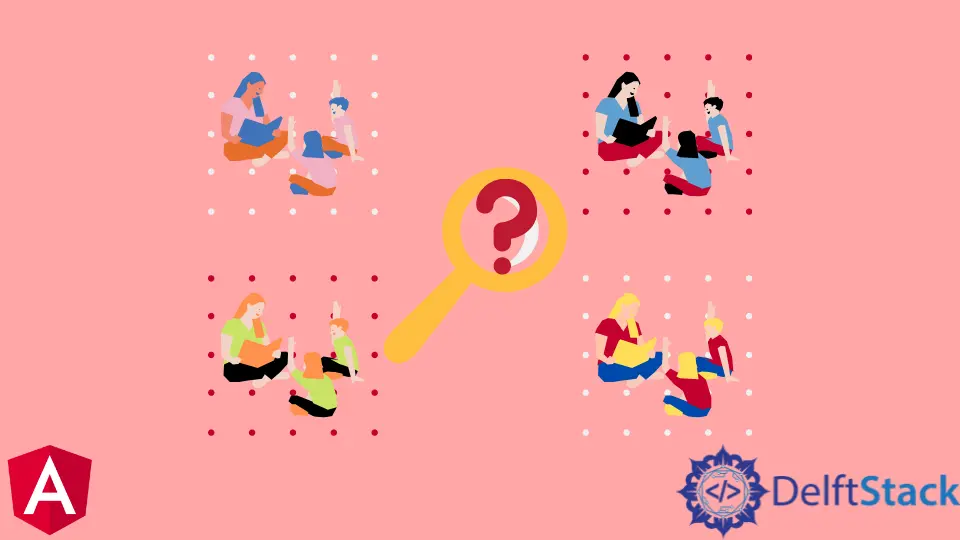
In Angular applications, dynamically interacting with elements based on their class names is crucial for implementing features such as styling changes, animations, or behavior modifications. This enables developers to create responsive and user-friendly interfaces.
In this article, we will explore various methods for finding elements by class name in Angular.
Find Elements by Class Name in Angular Using ViewChild
With ElementRef
While Angular predominantly emphasizes data binding and component interaction, there are scenarios where you might need to get elements by their class name.
Angular’s ViewChild
decorator is used to get a reference to a child component or an HTML element in the template. It allows you to access the properties and methods of the selected component or element directly from your component’s template class.
ElementRef
, on the other hand, is a class in Angular that represents a reference to a native element in the DOM. It is a powerful tool for interacting with the DOM directly when Angular’s data binding and directives are not sufficient.
To get elements by class name, you can use ViewChild
to get a element references in your template and then use ElementRef
to interact with its native element properties.
Step-By-Step Guide to Finding Elements by Class Name Using ViewChild
With ElementRef
Let’s have an example and break down the process into detailed step-by-step instructions.
Step 1: Create a New Angular Application
Open your terminal and run the following command to generate a new Angular application:
ng new my-app
Navigate to the app’s directory:
cd my-app
Launch the application to ensure all dependencies are installed correctly:
ng serve --open
Step 2: Import Necessary Modules and Create the Component
In your app.component.ts
file, import the required modules – Component
, ViewChild
, and ElementRef
.
import { Component, ElementRef, ViewChild } from '@angular/core';
Step 3: Implement the Component Logic
Within the AppComponent
class, create a private variable named ElByClassName
of type ElementRef
. This variable will hold the reference to the DOM element.
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
})
export class AppComponent {
constructor(private ElByClassName: ElementRef) {}
ngAfterViewInit() {
// Access the native DOM element from the HTML document using ElementRef object
const btnElement = (<HTMLElement>this.ElByClassName.nativeElement).querySelector('.myButton');
// Manipulate the element, for example, change its innerHTML
btnElement.innerHTML = 'This is Button';
}
}
Here, the ngAfterViewInit
lifecycle hook is used to ensure that the view is fully initialized before attempting to manipulate the DOM. Within the ngAfterViewInit
function, we obtain a reference to the button element with the class myButton
using ElementRef
.
We then manipulate the button’s content by changing its innerHTML
.
Step 4: Create a Template
In the app.component.html
file, create a button template with a specific class, for example, myButton
.
<button class="myButton">My Button</button>
Step 5: Run the Application
Save your changes and observe the output by running the application:
ng serve --open
Visit your application in the browser, and you’ll witness the button’s name transformed to This is Button
through the use of ViewChild
with ElementRef
.
Code Output:
Now that we have successfully implemented the basic functionality of finding and modifying HTML elements by class name using ViewChild
with ElementRef
, let’s explore how to enhance this functionality. One common scenario is handling user interactions, such as a button click, to trigger the element modification dynamically.
In the app.component.html
file, let’s add a button and bind a click event to it:
<button (click)="onButtonClick()">Modify Element</button>
The onButtonClick
method will be called by this button when clicked.
In your app.component.ts
file, implement the onButtonClick
method to dynamically modify the element on button click:
onButtonClick() {
const btnElement = (<HTMLElement>this.ElByClassName.nativeElement).querySelector('.myButton'); // Access and manipulate the first element with the specified class
btnElement.innerHTML = 'Button Modified!';
}
Now, when the Modify Element
button is clicked, it will dynamically change the content of the element with the class myButton
.
Code Output:
Find Elements by Class Name in Angular Using the ElementRef
and Renderer2
When it comes to manipulating the Document Object Model (DOM), Angular encourages the use of services such as ElementRef
and Renderer2
to ensure a consistent and secure approach.
As discussed above, the ElementRef
is a service in Angular that grants access to the underlying native element of a component. While it provides a direct link to the DOM, its usage comes with caution. Directly manipulating the DOM in an Angular component can lead to potential security vulnerabilities, and developers are advised to use Renderer2
for such operations.
The Renderer2
service acts as a layer of abstraction over the DOM, allowing developers to manipulate elements safely. It ensures that changes are applied consistently across various platforms, enhancing the cross-browser compatibility of your application.
Step-By-Step Guide to Finding Elements by Class Name Using the ElementRef
and Renderer2
Now, let’s walk through an example to better understand how to get elements by class name using ElementRef
and Renderer2
:
Step 1: Create a New Angular Application
Similar to the previous example, initiate a new Angular application by executing the following in your terminal:
ng new my-app
Navigate to the app’s directory:
cd my-app
Ensure all dependencies are installed correctly by serving the application:
ng serve --open
Step 2: Import Necessary Modules
In your app.component.ts
file, import the required modules – Renderer2
and ElementRef
.
import { Component, Renderer2, ElementRef } from '@angular/core';
Step 3: Implement the Logic in the Component
Class
Within the AppComponent
component class, inject Renderer2
and ElementRef
into the constructor. Create a function, for example, changeButtonName
, that utilizes these elements to locate and manipulate the desired element.
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
})
export class AppComponent {
constructor(private renderer: Renderer2, private el: ElementRef) {}
ngAfterViewInit() {
// Function called to manipulate the element
this.changeButtonName();
}
changeButtonName() {
// Access elements with the specified class name nativeElement property
const elements = this.el.nativeElement.getElementsByClassName('myButton');
// Loop through the elements and manipulate their content or attributes
for (let i = 0; i < elements.length; i++) {
// Use Renderer2 for safe HTML tag manipulations
this.renderer.setProperty(elements[i], 'innerHTML', 'This is Button');
}
}
}
The ngAfterViewInit
lifecycle hook ensures that the view is fully initialized before attempting to manipulate the DOM. Then, the changeButtonName
function uses Renderer2
and ElementRef
to access elements with the specified class name and modify their content using setProperty
.
Here, Angular’s nativeElement
property provides a direct reference to the underlying DOM node. This allows you to delegate actions directly to the DOM element using standard DOM APIs or jQuery-like methods.
Step 4: Create a Template
In the app.component.html
file, create a button with the class myButton
.
<button class="myButton">My Button</button>
Step 5: Run the Application
Save your changes and observe the output by running the application:
ng serve --open
Visit your application in the browser, and you’ll witness the button’s name dynamically changed to This is Button
through the use of Renderer2
with ElementRef
.
Code Output:
If you want to replace the entire element rather than modifying its content, you can use the outerHTML
property. Within the changeButtonName
function, replace the content manipulation with the following:
// modified changeButtonName function
changeButtonName() {
const elements = this.el.nativeElement.getElementsByClassName('myButton');
for (let i = 0; i < elements.length; i++) {
this.renderer.setProperty(elements[i], 'outerHTML', '<h1>This is Heading</h1>');
}
}
Save your changes and run the application once more. Now, instead of just changing the button’s name, each button has been replaced with an <h1>
heading.
Code Output:
Conclusion
In this article, we explored how to find an element by class name in Angular using ElementRef
and Renderer2
and ViewChild
with ElementRef
. By following the step-by-step guide and the provided examples, you should now have a clear understanding of how to leverage these Angular services for direct DOM manipulation when necessary.
Keep in mind that while these methods are available, it’s generally recommended to use Angular’s declarative and reactive approach for building scalable and maintainable applications.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn