How to Add Class to an Element in Angular
- Add Classes without Angular 4
-
Add Class to an Element Using the
ClassName
Property in Angular 4 -
Add Class to an Element Using the
NgClass
Directive in Angular 4
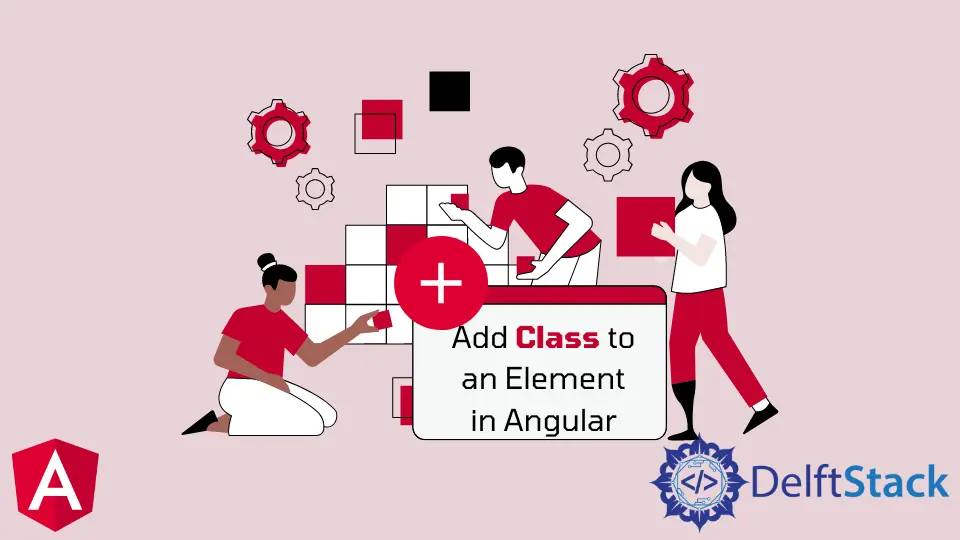
Angular 4 is a framework for building web applications. It is built on TypeScript, and it has many features that make it more powerful and flexible than Angular 2.
One of the features that make Angular 4 more powerful is the concept of adding classes to an element.
The easiest way to add a class to an element in Angular 4 is by using HTML attribute selector syntax with the NgClass
directive.
This syntax can be used on any HTML tag and allows you to apply multiple classes at once, which can then be applied as needed by other directives or stylesheet rules.
This article will thoroughly cover adding a class to an element in Angular 4.
Before we do that, we’ll examine how classes are allocated using traditional JavaScript versus how they are assigned in Angular.
Add Classes without Angular 4
The popular JavaScript library Angular provides a way to do this using the NgClass
directive. This directive allows you to add and remove classes from an element with ease dynamically.
However, if you are not using Angular, then there are a few other ways to use JavaScript to accomplish this task.
A jQuery plugin called addClass
allows you to easily add a class to any element as long as it has been initialized with jQuery. You can also use the .setAttribute()
method in JavaScript and set the className
property on the DOM node being manipulated.
Add Class to an Element Using the ClassName
Property in Angular 4
To directly bind to the className
property in Angular 4, we will use the ClassName
property. It adds classes to any element, accessed using brackets after the selector or by dot syntax ClassName
.
<div
[className]="isActive ? 'active' : 'inactive'">
</div>
If isActive
returns true, the active
class is added; otherwise, inactive
remains the same.
Add Class to an Element Using the NgClass
Directive in Angular 4
There are many use cases for the NgClass
directive. One of the most common is adding a class to an element when a user clicks on it.
The NgClass
directive allows you to add classes to an element based on certain conditions. For example, when a user clicks on a component, you can add the active class to that element.
The NgClass
directive is helpful because it allows you to dynamically add and remove classes from elements in your Angular application. Moreover, The directive itself is highly adaptable and does various things based on the input.
For instance, suppose we want to assign a static class using NgClass
. Let’s discuss its syntax.
<div [ngClass]="'Class-Name'">/div>
NgClass
can also assign numerous static class names simultaneously.
<div [ngClass]="['Class-Name', 'other-class']">/div>
Complete Example of Adding Classes to an Element in Angular 4
HTML code:
<p [ngClass]="something === 'first' ? 'blue red-border' : 'blue'">
Add some text here
</p>
<p [ngClass]="something === 'first' ? 'blue red-border' : 'blue'">
Add some text here
</p>
CSS code:
p {
font-family: Lato;
}
.blue {
background-color: blue;
}
.red-border {
border: 2px solid red;
}
*{
color: white;
}
TypeScript code:
import { Component, ViewChild, OnInit, Renderer2, ElementRef, } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
name = 'Angular 4';
something = 'first';
constructor(private renderer: Renderer2) {}
}
Click here to check the live demonstration of the examples mentioned above.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook