How to Use Angular stateParams
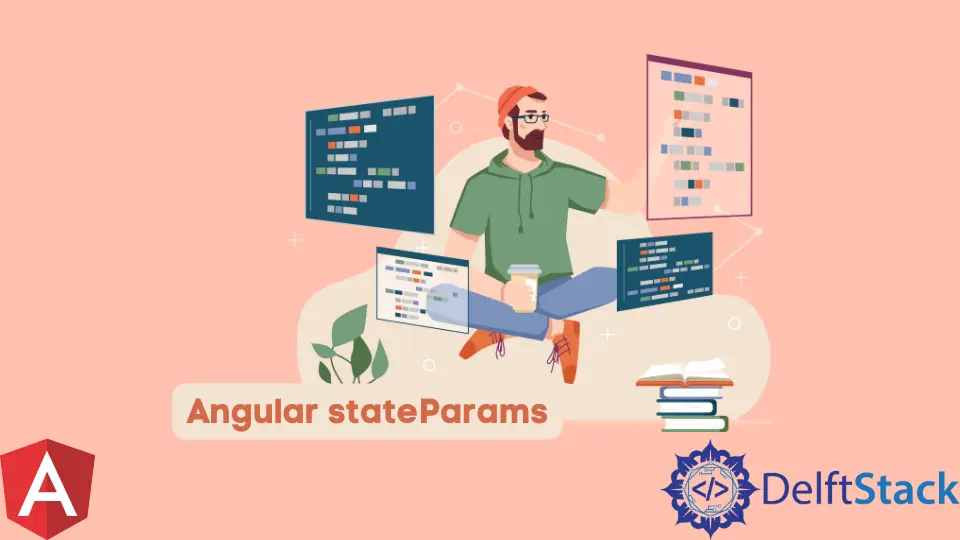
We will introduce the $stateParams
service and use it in Angular.
What Is the $stateParams
Service in Angular?
$stateParams
is a service that captures URL-based parameters, and we can use these parameters to display information according to the state.
Let’s create an example with two states, understand how states work, and use $stateparams
to store parameters.
We will use $stateProvider
to create a state. The state takes three parameters URL
, templateUrl
, and controller
.
In URL
, we add the page link where the state will go using state.go
. In templateUrl
, we will provide the name or URL of the template for that state. In a controller, we will provide the controller for the state.
Let’s create two states as LoginState
and SignUpState
. So our code will look like below.
console.clear();
var app = angular.module('app', [
'ui.router'
]);
app.config(function($stateProvider) {
$stateProvider
.state('LoginState', {
url: '',
templateUrl: 'Firststate',
controller : function ($scope, $state, $stateParams) {
$scope.params = $stateParams;
$scope.go = function () {
$state.go('SignUpState', { id : '2nd Parameter' });
};
console.log('Firststate params:', $stateParams);
}
})
.state('SignUpState', {
url: 'SignUpState/:id',
templateUrl: 'SecondState',
controller : function ($scope, $state, $stateParams) {
$scope.params = $stateParams;
$scope.go = function () {
$state.go('LoginState', { someOtherParam : '1st Parameter' });
};
console.log('SecondState params:', $stateParams);
}
});
});
Now let’s create a template for these templates and include Ui-router
script files. Our template will display the saved parameters and have a button to take us to SignUpState
and LoginState
, respectively.
<body ng-app="app">
<ui-view></ui-view>
<script type="text/ng-template" id="Firststate">
<h1>Login State</h1>
<h2>$stateParams: {{params}}</h2>
<p>(you can see it always empty)</p>
<button ng-click="go()">Sign Up</button>
</script>
<script type="text/ng-template" id="SecondState">
<h1>SignUp State</h1>
<h2>$stateParams: {{params}}</h2>
<button ng-click="go()">Login</button>
</script>
<script src="//cdnjs.cloudflare.com/ajax/libs/angular-ui-router/0.2.8/angular-ui-router.js"></script>
</body>
Output:
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn