How to Set Selected Option in Angular Dropdown
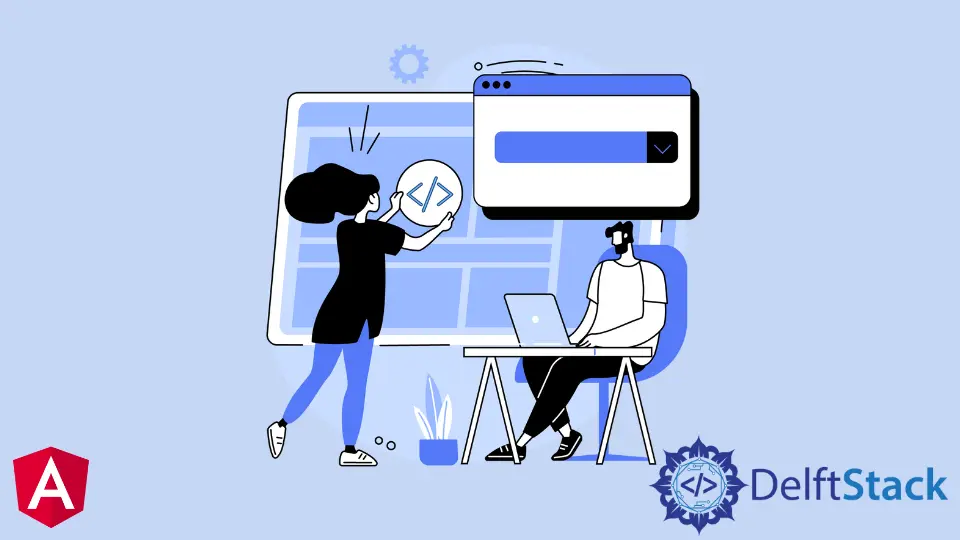
We will introduce how to set a selected option in an Angular dropdown, set a selected option from an array of options, and set a selected option if options are dynamic in Angular.
Set Selected Option in Angular Dropdown
This article will discuss setting a selected option for Angular dropdown. When creating a dropdown with multiple options, no option is selected automatically.
So, we will create a dropdown first and set a selected option, and we will discuss different scenarios on the best way to set a selected option when options are static or dynamic or when we have an array of options.
In the app.component.html
, we will create a select form.
# angular
<select [(ngModel)]='ngDropdown' name="dropdownOption" id="dropdownOption" class='form-control'>
<option value="1">1</option>
<option value="2">2</option>
<option value="3">3</option>
</select>
As seen in this code, we used[(ngModel)]
, which creates a FormControl
instance and binds it to a form control element.
We will add some CSS in app.component.css
to improve our dropdown form.
# angular
.form-control{
padding: 5px 10px;
background-color: rgb(52, 119, 179);
color: white;
border-color: black;
width: 100px;
height: 30px;
}
option:hover{
background-color: white;
color: rgb(52, 119, 179);
}
Output:
We can see no option is selected, but all the options appear when clicking on it.
To set an option as selected, we can assign the value to variable ngDropdown
in the app.component.ts
. Looking at our code from the app.component.html
, we’ll see that we already assigned the variable [(ngModel)]='ngDropdown'
.
Let’s try setting 1
as a default value.
# angular
import { Component, VERSION } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
ngDropdown = 1;
}
After assigning the selected option, we notice that the dropdown shows 1
as a selected option. In that way, we can select any option as a selected option in Angular.
Output:
Set Selected Option from an Array of Options
We will discuss how to set a default value from an array of options.
First of all, we will create two variables: ngDropdown
, the default option for our dropdown, and the second will be ngOptions
that will have all the options we want to display in the dropdown options.
So, let’s add this code in the app.component.ts
.
# angular
import { Component, VERSION } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
ngOptions = [1,2,3,4,5,6,7,8,9,10]
ngDropdown = 1;
}
In the ngOptions
array, we set values from 1
to 10
; in ngDropdown
, we set 1
as a default value.
We will create a select form in the app.component.html
.
# angular
<select class="form-control" id="DropdownOptions">
<option
*ngFor="let opt of ngOptions"
[selected]="opt === ngDropdown"
[value]="opt"
>
{{ opt }}
</option>
</select>
In this code, we’ll see that we set [selected]
to ngDropdown
, so it will automatically be selected when any option is the same as ngDropdown
.
Output:
Selected Option from Dynamic Values
We will discuss setting a selected option if we have dynamic values for options. First, we will create two variables; one will be ngDropdown
, the default option for our select form, and the second will be ngOptions
that will have all the values we want to display in the dropdown options.
We know that there will be dynamic values so let’s have random numbers array and set the selected option for the second item in that array.
# angular
import { Component, VERSION } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
ngOptions = [3,6,1,4,2,10,7,5,9,8]
ngDropdown = this.ngOptions[1];
}
The app.component.html
will have the following code.
# angular
<select class="form-control" id="DropdownOptions">
<option
*ngFor="let opt of ngOptions"
[selected]="opt === ngDropdown"
[value]="opt"
>
{{ opt }}
</option>
</select>
Output:
So, we can see that the second item of that array is set as a selected option. We can change this by changing the index of an array.
This tutorial taught us how to set the selected option for the Angular dropdown. We also discussed three different scenarios of selecting options when values are static, dynamic, or an array.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn