在 Angular 下拉菜单中选择选项
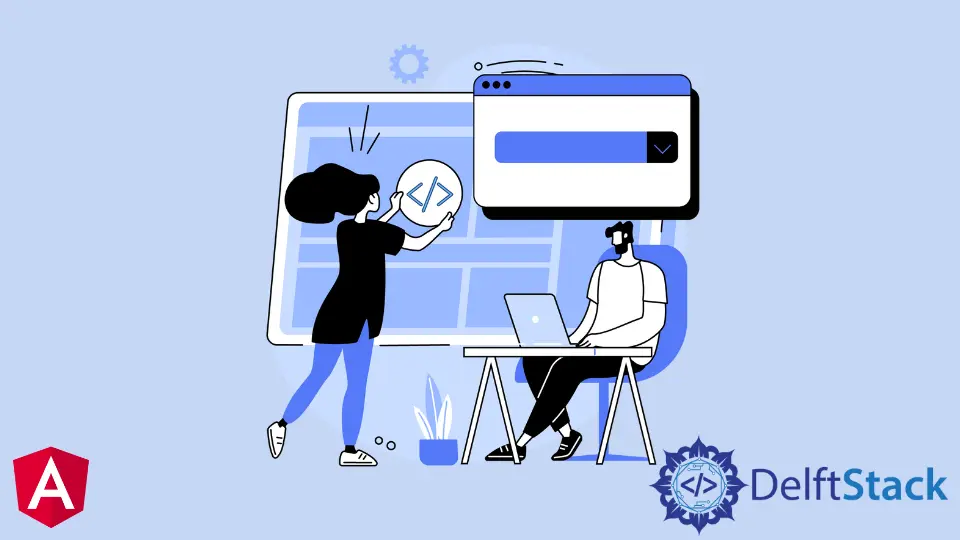
我们将介绍如何在 Angular 下拉列表中设置选定选项,从选项数组中设置选定选项,以及如果选项在 Angular 中是动态的,则设置选定选项。
在 Angular 下拉菜单中设置选定选项
本文将讨论为 Angular 下拉菜单设置一个选定的选项。创建具有多个选项的下拉列表时,不会自动选择任何选项。
因此,我们将首先创建一个下拉列表并设置选定选项,并在选项静态或动态或有一系列选项时讨论设置选定选项的最佳方法中讨论不同的方案。
在 app.component.html
中,我们将创建一个选择表单。
# angular
<select [(ngModel)]='ngDropdown' name="dropdownOption" id="dropdownOption" class='form-control'>
<option value="1">1</option>
<option value="2">2</option>
<option value="3">3</option>
</select>
正如在这段代码中看到的,我们使用了 [(ngModel)]
,它创建了一个 FormControl
实例并将其绑定到一个表单控件元素。
我们将在 app.component.css
中添加一些 CSS 来改进我们的下拉表单。
# angular
.form-control{
padding: 5px 10px;
background-color: rgb(52, 119, 179);
color: white;
border-color: black;
width: 100px;
height: 30px;
}
option:hover{
background-color: white;
color: rgb(52, 119, 179);
}
输出:
我们可以看到没有选择任何选项,但是单击它时会出现所有选项。
要将选项设置为选中,我们可以将值分配给 app.component.ts
中的变量 ngDropdown
。查看 app.component.html
中的代码,我们会看到我们已经分配了变量 [(ngModel)]='ngDropdown'
。
让我们尝试将 1
设置为默认值。
# angular
import { Component, VERSION } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
ngDropdown = 1;
}
分配选定选项后,我们注意到下拉菜单显示 1
作为选定选项。这样,我们可以选择任何选项作为 Angular 中的选定选项。
输出:
从选项数组中设置选定的选项
我们将讨论如何从一组选项中设置默认值。
首先,我们将创建两个变量:ngDropdown
,我们的下拉菜单的默认选项,第二个是 ngOptions
,它将包含我们想要在下拉选项中显示的所有选项。
所以,让我们在 app.component.ts
中添加这段代码。
# angular
import { Component, VERSION } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
ngOptions = [1,2,3,4,5,6,7,8,9,10]
ngDropdown = 1;
}
在 ngOptions
数组中,我们将值从 1
设置为 10
;在 ngDropdown
中,我们将 1
设置为默认值。
我们将在 app.component.html
中创建一个选择表单。
# angular
<select class="form-control" id="DropdownOptions">
<option
*ngFor="let opt of ngOptions"
[selected]="opt === ngDropdown"
[value]="opt"
>
{{ opt }}
</option>
</select>
在这段代码中,我们将看到我们将 [selected]
设置为 ngDropdown
,因此当任何选项与 ngDropdown
相同时,它将自动被选中。
输出:
从动态值中选择的选项
如果我们有选项的动态值,我们将讨论设置一个选定的选项。首先,我们将创建两个变量;第一个是 ngDropdown
,我们选择表单的默认选项,第二个是 ngOptions
,它将包含我们想要在下拉选项中显示的所有值。
我们知道会有动态值,所以让我们有一个随机数数组并为该数组中的第二项设置选定的选项。
# angular
import { Component, VERSION } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
ngOptions = [3,6,1,4,2,10,7,5,9,8]
ngDropdown = this.ngOptions[1];
}
app.component.html
将具有以下代码。
# angular
<select class="form-control" id="DropdownOptions">
<option
*ngFor="let opt of ngOptions"
[selected]="opt === ngDropdown"
[value]="opt"
>
{{ opt }}
</option>
</select>
输出:
因此,我们可以看到该数组的第二项设置为选定选项。我们可以通过改变数组的索引来改变它。
本教程教我们如何设置 Angular 下拉菜单的选定选项。我们还讨论了当值为静态、动态或数组时选择选项的三种不同场景。
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn