Angular Multi-Select Dropdown
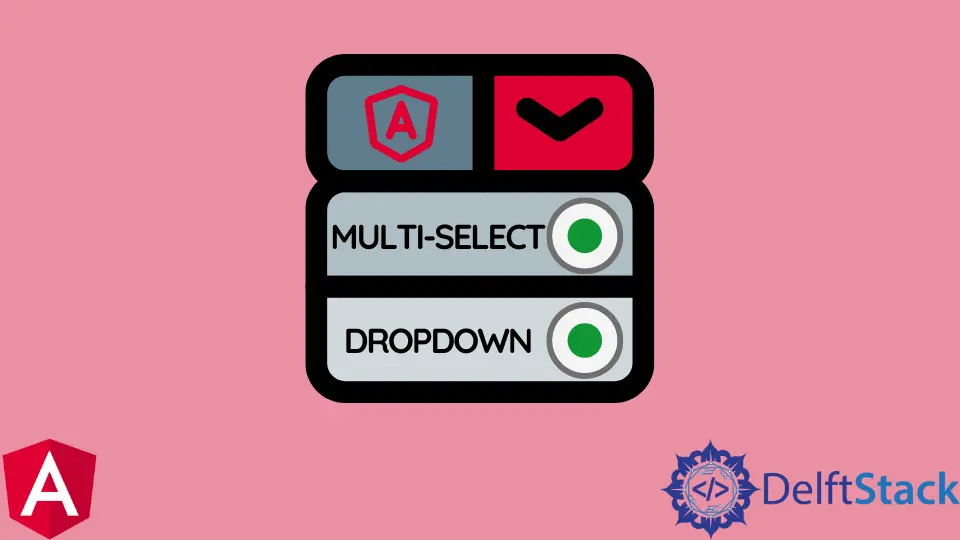
The dropdown is a ubiquitous component in any website. It’s used to filter the list of items, and it can be used for anything from displaying the list of countries to filtering the list of products.
This article is about the Angular multi-select dropdown and how to implement it in our Angular application.
Multi-Select Dropdown in Angular
The Angular Multi-Select Dropdown replaces the HTML select tag for picking several values. This textbox component allows users to write or pick multiple values from a predetermined list of possibilities.
Its built-in capabilities include data binding, sorting, grouping, labeling with custom values, and toggle mode.
The ng-multi-select
directive is useful when selecting multiple options from a list. It allows us to create a dropdown with multiple options that the user can select from.
The ng-select
directive is used as an attribute on an HTML element with the value of an expression that returns an array of items. The selected item(s) are then displayed in the dropdown list, and any items that are not selected are hidden.
This package also includes change events for obtaining the selected option value to enable the multi-select dropdown in Angular.
Create a Multi-Select Dropdown in Angular
This tutorial will teach creating a multi-select dropdown in Angular with ng-select
. We will use the Angular CLI
to generate our project.
First, open the terminal and type the following command to generate a project.
ng new angular-multi-dropdown
Next, go inside the project folder and type the command below.
ng serve
This command will start up a development server on our machine. Now, open your browser and type "localhost:4200"
in the address bar.
You should see a welcome message from our app. If you don’t see that, something went wrong with the ng serve
command, or you need to restart your computer/browser.
After that, use the following steps.
-
Add the
ng-select
directive to adiv
element. -
Add the
ng-model
directive to bind the value of an expression to a variable. -
Add the option element, an array of strings representing one option in the list.
-
Add an input element with
type = "checkbox"
for each option in the list and label attributes for each checkbox corresponding to its associated string from step 3 above, as well as a value attribute that corresponds to its index in step 3 (this will be0
unless you use numbers).
Below is a code example of the Angular multi-select dropdown.
Typescript Code:
import { Component, OnInit } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit {
dropdownList: { id: number; itemName: string; }[];
selectedItems: { id: number; itemName: string; }[];
dropdownSettings: { singleSelection: boolean; text: string; selectAllText: string; unSelectAllText: string; enableSearchFilter: boolean; classes: string; };
ngOnInit() {
this.dropdownList = [
{ id: 1, itemName: 'Netherlands' },
{ id: 2, itemName: 'Pakistan' },
{ id: 3, itemName: 'Australia' },
{ id: 4, itemName: 'USA' },
{ id: 5, itemName: 'Canada' },
];
this.selectedItems = [
{ id: 2, itemName: 'Pakistan' },
];
this.dropdownSettings = {
singleSelection: false,
text: 'Select Countries',
selectAllText: 'Select All',
unSelectAllText: 'UnSelect All',
enableSearchFilter: true,
classes: 'myclass custom-class'
};
}
onItemSelect(item: any) {
console.log(item);
console.log(this.selectedItems);
}
OnItemDeSelect(item: any) {
console.log(item);
console.log(this.selectedItems);
}
onSelectAll(items: any) {
console.log(items);
}
onDeSelectAll(items: any) {
console.log(items);
}
}
Html Code:
<h2>Example of Angular Multi Select Dropdown</h2>
<angular2-multiselect [data]="dropdownList" [(ngModel)]="selectedItems"
[settings]="dropdownSettings"
(onSelect)="onItemSelect($event)"
(onDeSelect)="OnItemDeSelect($event)"
(onSelectAll)="onSelectAll($event)"
(onDeSelectAll)="onDeSelectAll($event)">
</angular2-multiselect>
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook