How to Set Default Value in Angular
- Set Default Value in Angular
- Set Default Value From an Array of Options in Angular
- Select Default Value From Dynamic Values in Angular
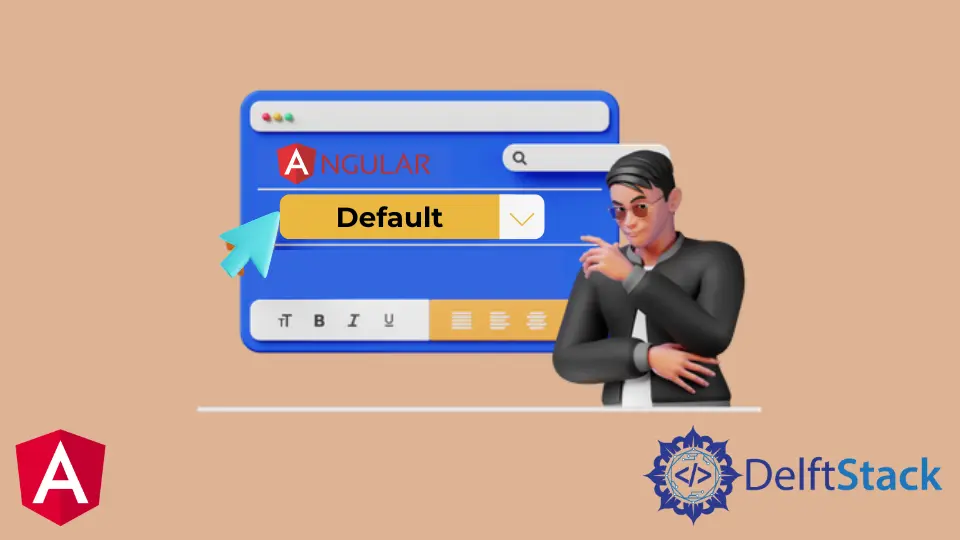
We will learn how to set a default value in a select
form, set a default value from an array of options, and set a default value if options are dynamic in Angular.
Set Default Value in Angular
This tutorial will discuss how to set a default value for a select tag in Angular. No default value is automatically selected when creating a select
form with multiple options.
Therefore, we will create a select form first and set a default value, and we will discuss different scenarios on the best way to set a default value when options are static or dynamic or when we have an array of options.
In app.component.html
, we will create a select
form.
# angular
<select [(ngModel)]='ngSelect' name="selectOption" id="selectOption" class='form-control'>
<option value="1">1</option>
<option value="2">2</option>
<option value="3">3</option>
</select>
We will add CSS in app.component.css
to make our select
form look better.
# angular
.form-control{
padding: 5px 10px;
background-color: DodgerBlue;
color: white;
border-color: black;
width: 100px;
height: 30px;
}
option:hover{
background-color: white;
color: dodgerblue;
}
Output:
You can see no default value is selected, but all the options appear when we click on it.
To set any value as a default, we can assign the value to variable ngSelect
in app.component.ts
. If you see our code from app.component.html
, you can see that we already assigned the variable [(ngModel)]='ngSelect'
.
Now let’s try setting 1
as a default value.
# angular
import { Component, VERSION } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
ngSelect = 1;
}
After assigning the default value, we will notice that the select form shows 1
as a current default value. In that way, we can select any value as a default value in Angular.
Output:
Set Default Value From an Array of Options in Angular
We will discuss setting a default value from an array of options.
First of all, we will create two variables: ngSelect
, the default option for our select form, and the second will be options
that will have all the values we want to display in Select options.
Let’s add this code in app.component.ts
.
# angular
import { Component, VERSION } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
options = [1,2,3,4,5,6,7,8,9,10]
ngSelect = 1;
}
In the options
array, we set values from 1
to 10
; in ngSelect
, we set 1
as a default value.
We will create the select form in app.component.html
.
# angular
<select class='form-control' id="selectOptions">
<option *ngFor="let opt of options"
[selected]="opt === ngSelect"
[value]="opt">
{{ opt }}
</option>
</select>
In this code, you can see that we have set [selected]
to ngSelect
, so when any option is the same as ngSelect
, it will automatically be selected.
Output:
Select Default Value From Dynamic Values in Angular
We will discuss setting a default value if we have dynamic values for options.
First of all, we will create two variables: ngSelect
, the default option for our select form, and the second will be options
that will have all the values we want to display in Select options.
We know there will be dynamic values, so let’s have a random numbers array and set the default value for the first item in that array.
# angular
import { Component, VERSION } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
options = [3,6,1,4,2,10,7,5,9,8]
ngSelect = this.options[0];
}
app.component.html
will have the following code.
# angular
<select class="form-control" id="selectOptions">
<option
*ngFor="let opt of options"
[selected]="opt === ngSelect"
[value]="opt"
>
{{ opt }}
</option>
</select>
Output:
You can see that the first item of that array is set as a default value. We can change this by changing the index of an array.
This tutorial taught us to set the default value for the select
form in Angular. We also discussed three default values with static, dynamic, or array values.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn