How to Reset Form Using AngularJS setPristine
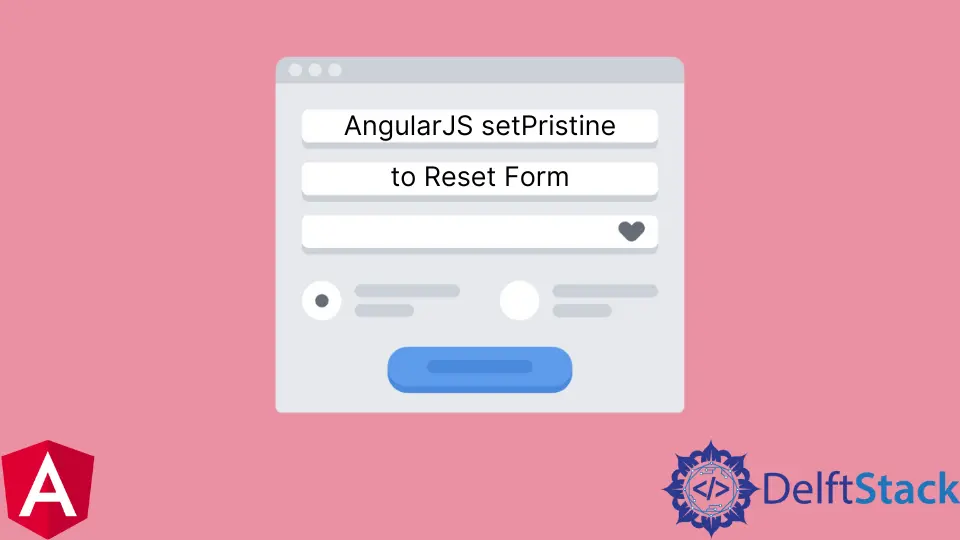
One of the things to get used to as developers is the various jargon used to describe some functions. An example of such are functions like $setPristine
state and $dirty
state.
To better understand what the $setPristine
state means, we will compare it with the $dirty
state.
When a web user visits a page and has to fill a form, as soon as the user types into a form field, that field is in a $dirty
state. Before the user types into that form field, the form is in a $setPristine
state because the form field is untouched.
The $setPristine
state is handy when a user fills a form and clicks submit, we want that form field to reset and the user’s data cleared. Now let us look at how to get this function up and running.
How to Reset a Form to $setPristine
State in AngularJS
First, let us create an HTML structure, including an input field where we will type in some words. Then a reset button will clear off the input field, returning it to a clean state.
We could also say we will return the form from its dirty state to its pristine state.
<div ng-app="myApp">
<div ng-controller="MyCtrl">
<form name="form">
<input name="requiredField" ng-model="model.requiredField" required/> (Required, but no other validators)
<p ng-show="form.requiredField.$errror.required">Field is required</p>
<button ng-click="reset()">Reset form</button>
</form>
<p>Pristine: {{form.$pristine}}</p>
</div>
</div>
Next up, we head into the JavaScript section of our project. First, we need to provide a method in our controller that will reset the model because once we reset the form, we also have to reset the model.
Also, we will include the resettableform
module in the project. In the end, the JavaScript file will look like below.
var myApp = angular.module('myApp', ['resettableForm']);
function MyCtrl($scope) {
$scope.reset = function() {
$scope.form.$setPristine();
$scope.model = '';
};
}
(function(angular) {
function indexOf(array, obj) {
if (array.indexOf) return array.indexOf(obj);
for ( var i = 0; i < array.length; i++) {
if (obj === array[i]) return i;
}
return -1;
}
function arrayRemove(array, value) {
var index = indexOf(array, value);
if (index >=0)
array.splice(index, 1);
return value;
}
var PRISTINE_CLASS = 'ng-pristine';
var DIRTY_CLASS = 'ng-dirty';
var formDirectiveFactory = function(isNgForm) {
return function() {
var formDirective = {
restrict: 'E',
require: ['form'],
compile: function() {
return {
pre: function(scope, element, attrs, ctrls) {
var form = ctrls[0];
var $addControl = form.$addControl;
var $removeControl = form.$removeControl;
var controls = [];
form.$addControl = function(control) {
controls.push(control);
$addControl.apply(this, arguments);
}
form.$removeControl = function(control) {
arrayRemove(controls, control);
$removeControl.apply(this, arguments);
}
form.$setPristine = function() {
element.removeClass(DIRTY_CLASS).addClass(PRISTINE_CLASS);
form.$dirty = false;
form.$pristine = true;
angular.forEach(controls, function(control) {
control.$setPristine();
});
}
},
};
},
};
return isNgForm ? angular.extend(angular.copy(formDirective), {restrict: 'EAC'}) : formDirective;
};
}
var ngFormDirective = formDirectiveFactory(true);
var formDirective = formDirectiveFactory();
angular.module('resettableForm', []).
directive('ngForm', ngFormDirective).
directive('form', formDirective).
directive('ngModel', function() {
return {
require: ['ngModel'],
link: function(scope, element, attrs, ctrls) {
var control = ctrls[0];
control.$setPristine = function() {
this.$dirty = false;
this.$pristine = true;
element.removeClass(DIRTY_CLASS).addClass(PRISTINE_CLASS);
}
},
};
});
})(angular);
At this point, if we have followed the code snippets, everything should work fine. We could include this CSS snippet in our code to beautify things.
input.ng-dirty.ng-invalid {
background-color: red;
}
input[required].ng-pristine {
background-color: yellow;
}
input[required].ng-dirty.ng-valid {
background-color: green;
color: white;
}
Conclusion
Setting a form back to its pristine state helps show a web user that the website they visited pays attention to details; it also depicts the website as clean.
Additionally, when we reset a form back to its pristine state, we reset the model, which improves the website’s overall performance because the project bundle is lighter since nothing unnecessary is stored.
Fisayo is a tech expert and enthusiast who loves to solve problems, seek new challenges and aim to spread the knowledge of what she has learned across the globe.
LinkedIn