How to Add Custom Form Validation in AngularJS
- Form Validators in AngularJS
- Custom Validators in AngularJS
- Steps to Create or Add Custom Form Validation in AngularJS
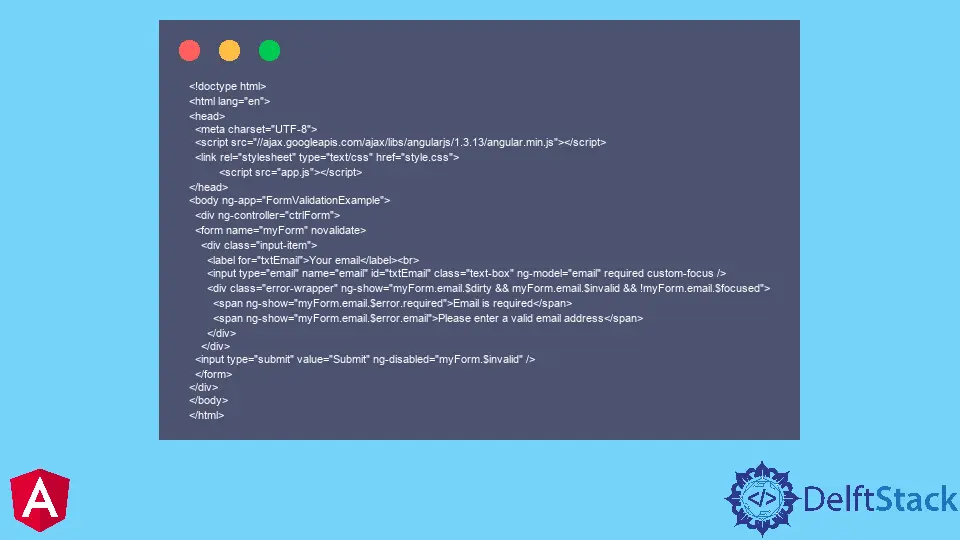
Validation is a process of scanning the correctness of input data, typically for error correction or for data consistency. AngularJS custom validation is a powerful library that allows developers to create custom validation rules.
In this article, we will discuss everything about AngularJS custom validation. Let’s start with form validators.
Form Validators in AngularJS
There are two types of fundamental forms supported by Angular.
- Template-driven forms
- Reactive forms
The template-driven form provides a declarative, easy-to-use, well-tested way to create a form. This is the default form in Angular.
The reactive form is a more low-level API that gives you more control over the behavior of your form. It is designed to use reactive data sources such as observables or event emitters.
Every field in a form can function as a FormControl
, returning specific info about that field, such as whether the form is valid, the actual field value, and other details.
Custom Validators in AngularJS
Angular has a built-in system for validating data called the Validator API. But, when it comes to more complex validation rules or when the validation rules are specific to your application, you can use custom validators.
Custom validators in Angular are very useful because they allow developers to create their own validation rules without relying on external libraries. They also make it more straightforward for developers to extend the validation logic outside of Angular and other application parts.
It is important to note that AngularJS custom validation can be used with any other validation library, such as jQuery, but it’s not required.
Steps to Create or Add Custom Form Validation in AngularJS
The following steps will guide you in creating AngularJS custom form validation.
- Create a new AngularJS project.
- Include the
ng-bootstrap
CSS file in ourindex.html
file. - Add the
ng-bootstrap
JavaScript file to ourindex.html
file. - Create a custom form with an input field in the HTML page and include it in the body section of
index.html
. - Create an Angular controller and add it to
index.html
. - Create an Angular form directive and add it to
index.html
. - Inject the form controller into our angular controller.
Let’s discuss an example of custom form validation in AngularJS.
HTML Code:
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<script src="//ajax.googleapis.com/ajax/libs/angularjs/1.3.13/angular.min.js"></script>
<link rel="stylesheet" type="text/css" href="style.css">
<script src="app.js"></script>
</head>
<body ng-app="FormValidationExample">
<div ng-controller="ctrlForm">
<form name="myForm" novalidate>
<div class="input-item">
<label for="txtEmail">Your email</label><br>
<input type="email" name="email" id="txtEmail" class="text-box" ng-model="email" required custom-focus />
<div class="error-wrapper" ng-show="myForm.email.$dirty && myForm.email.$invalid && !myForm.email.$focused">
<span ng-show="myForm.email.$error.required">Email is required</span>
<span ng-show="myForm.email.$error.email">Please enter a valid email address</span>
</div>
</div>
<input type="submit" value="Submit" ng-disabled="myForm.$invalid" />
</form>
</div>
</body>
</html>
JavaScript Code:
angular.module('FormValidationExample', [])
.controller('ctrlForm', ['$scope', function($scope) {
$scope.checkboxes_motives = { 'checked': false, items: {} };
$scope.choices = ['work', 'play'];
$scope.selectAllMotives = function(value) {
angular.forEach($scope.choices, function (motive) {
$scope.checkboxes_motives.items[motive] = value;
});
};
}])
.directive('customFocus', [function() {
var FOCUS_CLASS = "custom-focused";
return {
restrict: 'A',
require: 'ngModel',
link: function(scope, element, attrs, ctrl) {
ctrl.$focused = false;
element.bind('focus', function(evt) {
element.addClass(FOCUS_CLASS);
scope.$apply(function() {ctrl.$focused = true;});
}).bind('blur', function(evt) {
element.removeClass(FOCUS_CLASS);
scope.$apply(function() {ctrl.$focused = false;});
});
}
}
}]);
In this example, we validated an email address. If you write an incorrect email address here, it will show an error until or unless you make it correct.
Click here to check the live demonstration of the code as mentioned above.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook