JavaScript Math.log() Method
-
Syntax of JavaScript
Math.log()
: -
Example Code: Use the
Math.log()
Method to Get the Natural Logarithm of Positive Numbers -
Example Code: Use the
Math.log()
Method With Zeros and Negative Numbers -
Example Code: Use the
Math.log()
Method to Change the Base of the Logarithm
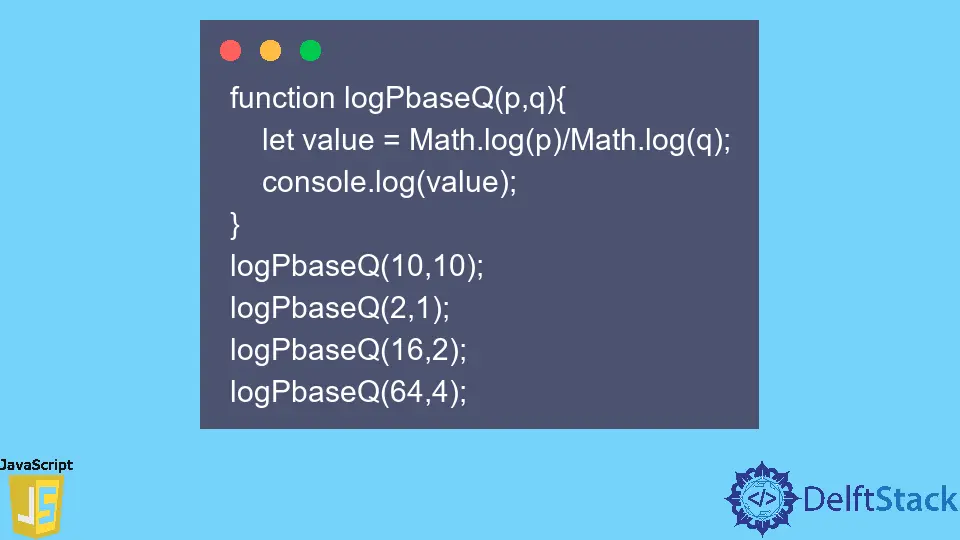
Users can use the Math.log()
method to get the natural logarithm of the numeric value. The natural logarithm’s base is e
.
Syntax of JavaScript Math.log()
:
Math.log(value);
Parameters
value |
It is a positive number value to find its natural logarithm. |
Return
The Math.log()
method returns the natural logarithm for the positive value
and NaN
for the negative values. It returns -Infinity
for 0.
Example Code: Use the Math.log()
Method to Get the Natural Logarithm of Positive Numbers
We can use the Math.log()
method to find the log of any positive numeric values on the base e
. We have taken different numeric values in the example below to determine its natural logarithm.
In the output, users can see that if we take the value < 1
, it always returns the negative result, and for others, it gives the positive output.
let value = 20;
console.log(Math.log(value));
console.log(Math.log(10.23));
console.log(Math.log(0.352));
Output:
2.995732273553991
2.325324579963535
-1.04412410338404
Example Code: Use the Math.log()
Method With Zeros and Negative Numbers
In the following example, we used the Math.log()
method with the 0 value, which returns the -Infinity
. Also, we have used the Math.log()
method with the negative values, which produces NaN
values that we can see in the output.
let value = 0;
let value1 = -20;
console.log(Math.log(value));
console.log(Math.log(value1));
console.log(Math.log(-0.533));
console.log(Math.log(-1.533));
Output:
-Infinity
NaN
NaN
NaN
Example Code: Use the Math.log()
Method to Change the Base of the Logarithm
We can modify the base of the logarithm using the Math.log()
method. We have created the function below to get the logarithm of values
with the different bases.
The below function will print the logarithm of p with base q rather than e
. Also, we can conclude that when we use base 1, the method always returns the positive Infinity value.
function logPbaseQ(p,q){
let value = Math.log(p)/Math.log(q);
console.log(value);
}
logPbaseQ(10,10);
logPbaseQ(2,1);
logPbaseQ(16,2);
logPbaseQ(64,4);
Output:
1
Infinity
4
3
The Math.log()
method helps find the logarithm of any positive value with a different base, which we have seen in this article.