如何在 Matplotlib 中绘制数据列表的直方图
Suraj Joshi
2024年2月15日
Matplotlib
Matplotlib Visualizations
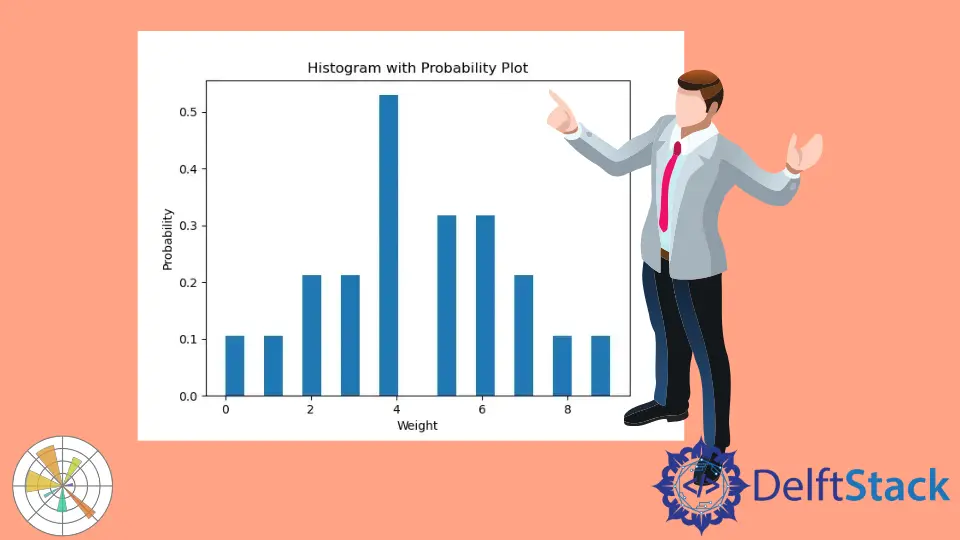
我们可以使用 plt.hist()
方法从数据列表中绘制直方图。
plt.hist()
方法的语法
matplotlib.pyplot.hist(x,
bins=None,
range=None,
density=False,
weights=None,
cumulative=False,
bottom=None,
histtype='bar',
align='mid',
orientation='vertical',
rwidth=None,
log=False,
color=None,
label=None,
stacked=False, *,
data=None,
**kwargs)
示例:从数据列表中使用 Matplotlib 绘制直方图
import matplotlib.pyplot as plt
data = [3, 4, 2, 3, 4, 5, 4, 7, 8, 5, 4, 6, 2, 1, 0, 9, 7, 6, 6, 5, 4]
n, bins, patches = plt.hist(data)
plt.xlabel("Values")
plt.ylabel("Frequency")
plt.title("Histogram")
plt.show()
输出:
在这里,我们在一个列表 data
中有数值。我们将这个列表传入 plt.hist()
命令,从值列表中生成一个直方图。plt.hist()
方法会返回二进制的频率、二进制的端点以及用于创建直方图的补丁列表。
在本例中,我们没有设置 bins
参数的值。默认情况下,bins
的值是 10,所以脚本会从 10 个 bins 的数据列表中创建直方图。
此外,我们可以在制作直方图时使用 bins
命令控制直方块的数量。
import matplotlib.pyplot as plt
data = [3, 4, 2, 3, 4, 5, 4, 7, 8, 5, 4, 6, 2, 1, 0, 9, 7, 6, 6, 5, 4]
n, bins, patches = plt.hist(data, bins=20)
plt.xlabel("Values")
plt.ylabel("Frequency")
plt.title("Histogram with 20 bins")
plt.show()
输出:
这个过程显示了由 20 个直方块组成的直方图,这是平均划分整个列表值范围的结果。
默认情况下,density
参数的值被设置为 False
;这意味着我们在直方图中得到每个 bin
的精确计数的图。如果我们想让列表中每个 bin 的概率密度图,我们需要将 density
设置为 True
。如果 density
为 True
,直方图下的区域积分为 1
。
import matplotlib.pyplot as plt
data = [3, 4, 2, 3, 4, 5, 4, 7, 8, 5, 4, 6, 2, 1, 0, 9, 7, 6, 6, 5, 4]
n, bins, patches = plt.hist(data, bins=20, density=True)
plt.xlabel("Weight")
plt.ylabel("Probability")
plt.title("Histogram with Probability Plot")
plt.show()
输出:
现在我们可以使用 color
参数设置直方图的颜色。
import matplotlib.pyplot as plt
data = [3, 4, 2, 3, 4, 5, 4, 7, 8, 5, 4, 6, 2, 1, 0, 9, 7, 6, 6, 5, 4]
n, bins, patches = plt.hist(data, bins=20, density=True, color="red")
plt.xlabel("Weight")
plt.ylabel("Probability")
plt.title("Red Histogram Plot")
plt.show()
输出:
此方法生成红色的直方图。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn