如何在 Java 中獲取檔案的副檔名
-
使用 Java 中的
getExtension()
方法獲取副檔名 -
用 Java 中的
lastIndexOf()
方法獲取檔案的副檔名 - 用 Java 中的字串解析獲取檔案的副檔名
-
使用 Java 中的
replaceAll()
方法獲取檔案的副檔名 -
在 Java 中使用
contains()
和lastIndexOf()
方法獲取副檔名 - 使用 Java 中的三元運算子獲取副檔名
-
在 Java 中使用
stream()
方法獲取檔案的副檔名 - 使用 Java 中的 Regex 獲取檔案的副檔名
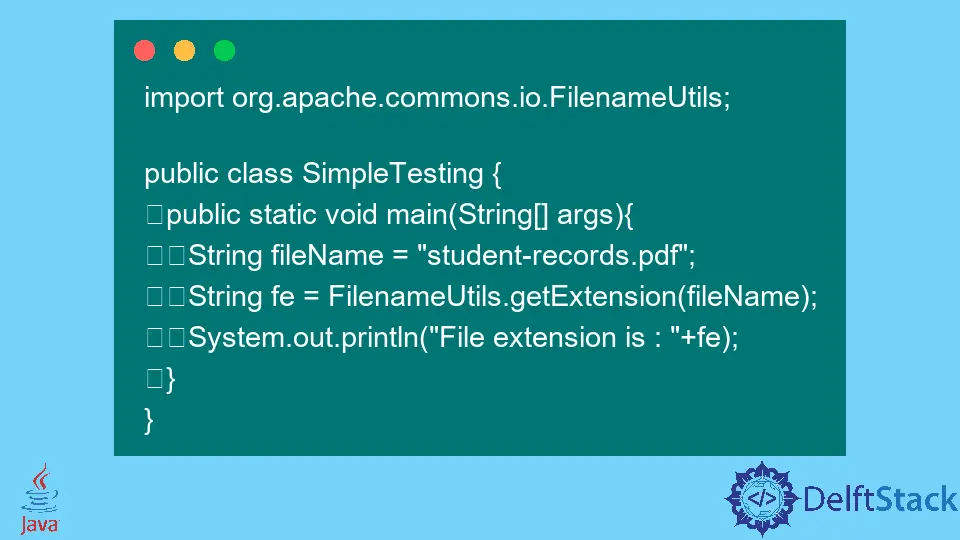
本教程介紹瞭如何在 Java 中獲取檔案的副檔名,還列舉了一些示例程式碼來理解這個話題。
使用 Java 中的 getExtension()
方法獲取副檔名
要獲得檔案的副檔名,我們可以使用 FilenameUtils
類的 getExtension()
方法。這個方法返回檔案的副檔名。由於這個方法屬於 Apache
commons 庫,所以你必須從 Apache 官網下載這個庫才能在專案中使用 JAR。
import org.apache.commons.io.FilenameUtils;
public class SimpleTesting {
public static void main(String[] args) {
String fileName = "student-records.pdf";
String fe = FilenameUtils.getExtension(fileName);
System.out.println("File extension is : " + fe);
}
}
輸出:
File extension is : pdf
用 Java 中的 lastIndexOf()
方法獲取檔案的副檔名
如果你不想使用任何內建的方法,那麼使用給定的程式碼示例,使用 lastIndexOf()
方法來獲取副檔名。這是最簡單易行的方法,只涉及字串方法。請看下面的例子。
public class SimpleTesting {
public static void main(String[] args) {
String fileName = "student-records.pdf";
String fe = "";
int i = fileName.lastIndexOf('.');
if (i > 0) {
fe = fileName.substring(i + 1);
}
System.out.println("File extension is : " + fe);
}
}
輸出:
File extension is : pdf
用 Java 中的字串解析獲取檔案的副檔名
這是另一種解決方案,包括幾種情況,其中包括(如果檔案路徑中有點 .
)。即使檔案路徑之間有點(.
),這種方法也會返回準確的結果。請看下面的例子。
public class SimpleTesting {
public static void main(String[] args) {
String fileName = "folder\s.gr\fg\student-records.pdf";
String fe = "";
char ch;
int len;
if (fileName == null || (len = fileName.length()) == 0 || (ch = fileName.charAt(len - 1)) == '/'
|| ch == '\\' || ch == '.') {
fe = "";
}
int dotInd = fileName.lastIndexOf('.'),
sepInd = Math.max(fileName.lastIndexOf('/'), fileName.lastIndexOf('\\'));
if (dotInd <= sepInd) {
fe = "";
} else {
fe = fileName.substring(dotInd + 1).toLowerCase();
}
System.out.println("File extension is : " + fe);
}
}
輸出:
File extension is : pdf
使用 Java 中的 replaceAll()
方法獲取檔案的副檔名
我們可以使用 replaceAll()
方法來獲取副檔名,就像我們在下面的例子中所做的那樣。我們在這個方法中使用正規表示式並將結果收集到一個變數中。
public class SimpleTesting {
public static void main(String[] args) {
String fileName = "folder\s.gr\fg\student-records.pdf";
String fe = "";
fe = fileName.replaceAll("^.*\\.(.*)$", "$1");
System.out.println("File extension is : " + fe);
}
}
輸出:
File extension is : pdf
在 Java 中使用 contains()
和 lastIndexOf()
方法獲取副檔名
contains()
方法用於檢查指定的字元是否存在於字串中,lastIndexOf()
方法返回指定字元的索引值,該索引值被傳遞到 substring()
方法中以獲取副檔名。我們在這段程式碼中使用這些方法來獲取副檔名。請看下面的例子。
public class SimpleTesting {
public static void main(String[] args) {
String fileName = "folder\s.gr\fg\student-records.pdf";
String fe = "";
if (fileName.contains("."))
fe = fileName.substring(fileName.lastIndexOf(".") + 1);
System.out.println("File extension is : " + fe);
}
}
輸出:
File extension is : pdf
使用 Java 中的三元運算子獲取副檔名
如果你對三元運算子(?, :
)很熟悉,那麼就把它和 substring()
和 lastIndexOf()
方法一起使用。它減少了程式碼的行數,並在一條語句中返回結果。
public class SimpleTesting {
public static void main(String[] args) {
String fileName = "folder\s.gr\fg\student-records.pdf";
String fe = "";
if (fileName.contains(".")) {
int i = fileName.lastIndexOf('.');
fe = i > 0 ? fileName.substring(i + 1) : "";
}
System.out.println("File extension is : " + fe);
}
}
輸出:
File extension is : pdf
在 Java 中使用 stream()
方法獲取檔案的副檔名
我們可以使用 Arrays
類的 stream()
方法將檔名轉換成流,並使用 split()
方法將檔名從點(.
)中斷開。請看下面的例子。
import java.util.Arrays;
public class SimpleTesting {
public static void main(String[] args) {
String fileName = "folder\s.gr\fg\student-records.pdf";
String fe = "";
if (fileName.contains(".")) {
fe = Arrays.stream(fileName.split("\\.")).reduce((a, b) -> b).orElse(null);
}
System.out.println("File extension is : " + fe);
}
}
輸出:
File extension is : pdf
使用 Java 中的 Regex 獲取檔案的副檔名
這是另一個使用 regex
包的解決方案。在這個 Java 例子中,Pattern
類的 compile()
和 matcher()
方法被用來獲取檔案的副檔名。請看下面的例子。
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class SimpleTesting {
public static void main(String[] args) {
String fileName = "folder\s.gr\fg\student-records.pdf";
String fe = "";
final Pattern PATTERN = Pattern.compile("(.*)\\.(.*)");
Matcher m = PATTERN.matcher(fileName);
if (m.find()) {
fe = m.group(2);
}
System.out.println("File extension is : " + fe);
}
}
輸出:
File extension is : pdf