如何在 Java 中获取文件的文件扩展名
-
使用 Java 中的
getExtension()
方法获取文件扩展名 -
用 Java 中的
lastIndexOf()
方法获取文件的扩展名 - 用 Java 中的字符串解析获取文件的文件扩展名
-
使用 Java 中的
replaceAll()
方法获取文件的文件扩展名 -
在 Java 中使用
contains()
和lastIndexOf()
方法获取文件扩展名 - 使用 Java 中的三元运算符获取文件扩展名
-
在 Java 中使用
stream()
方法获取文件的文件扩展名 - 使用 Java 中的 Regex 获取文件的文件扩展名
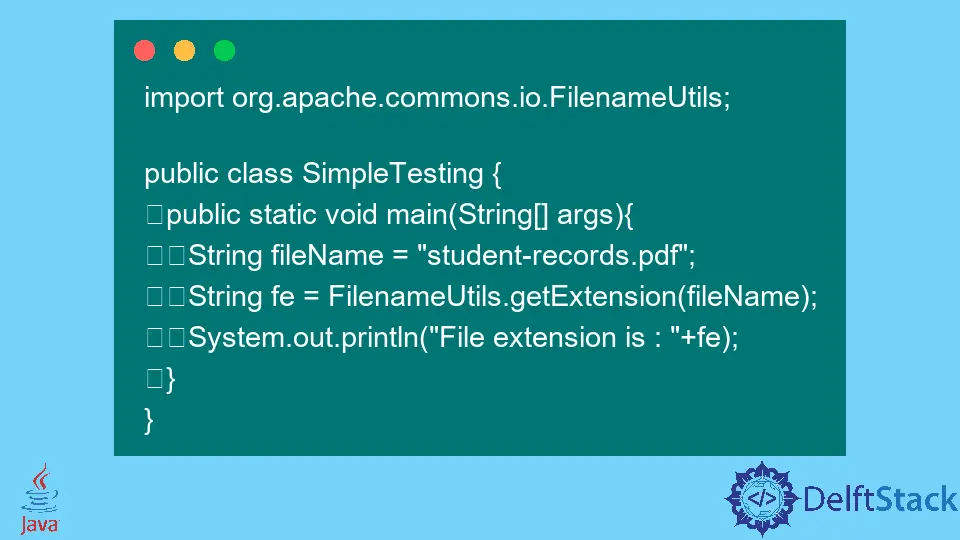
本教程介绍了如何在 Java 中获取文件的文件扩展名,还列举了一些示例代码来理解这个话题。
使用 Java 中的 getExtension()
方法获取文件扩展名
要获得文件的扩展名,我们可以使用 FilenameUtils
类的 getExtension()
方法。这个方法返回文件的扩展名。由于这个方法属于 Apache
commons 库,所以你必须从 Apache 官网下载这个库才能在项目中使用 JAR。
import org.apache.commons.io.FilenameUtils;
public class SimpleTesting {
public static void main(String[] args) {
String fileName = "student-records.pdf";
String fe = FilenameUtils.getExtension(fileName);
System.out.println("File extension is : " + fe);
}
}
输出:
File extension is : pdf
用 Java 中的 lastIndexOf()
方法获取文件的扩展名
如果你不想使用任何内置的方法,那么使用给定的代码示例,使用 lastIndexOf()
方法来获取文件扩展名。这是最简单易行的方法,只涉及字符串方法。请看下面的例子。
public class SimpleTesting {
public static void main(String[] args) {
String fileName = "student-records.pdf";
String fe = "";
int i = fileName.lastIndexOf('.');
if (i > 0) {
fe = fileName.substring(i + 1);
}
System.out.println("File extension is : " + fe);
}
}
输出:
File extension is : pdf
用 Java 中的字符串解析获取文件的文件扩展名
这是另一种解决方案,包括几种情况,其中包括(如果文件路径中有点 .
)。即使文件路径之间有点(.
),这种方法也会返回准确的结果。请看下面的例子。
public class SimpleTesting {
public static void main(String[] args) {
String fileName = "folder\s.gr\fg\student-records.pdf";
String fe = "";
char ch;
int len;
if (fileName == null || (len = fileName.length()) == 0 || (ch = fileName.charAt(len - 1)) == '/'
|| ch == '\\' || ch == '.') {
fe = "";
}
int dotInd = fileName.lastIndexOf('.'),
sepInd = Math.max(fileName.lastIndexOf('/'), fileName.lastIndexOf('\\'));
if (dotInd <= sepInd) {
fe = "";
} else {
fe = fileName.substring(dotInd + 1).toLowerCase();
}
System.out.println("File extension is : " + fe);
}
}
输出:
File extension is : pdf
使用 Java 中的 replaceAll()
方法获取文件的文件扩展名
我们可以使用 replaceAll()
方法来获取文件扩展名,就像我们在下面的例子中所做的那样。我们在这个方法中使用正则表达式并将结果收集到一个变量中。
public class SimpleTesting {
public static void main(String[] args) {
String fileName = "folder\s.gr\fg\student-records.pdf";
String fe = "";
fe = fileName.replaceAll("^.*\\.(.*)$", "$1");
System.out.println("File extension is : " + fe);
}
}
输出:
File extension is : pdf
在 Java 中使用 contains()
和 lastIndexOf()
方法获取文件扩展名
contains()
方法用于检查指定的字符是否存在于字符串中,lastIndexOf()
方法返回指定字符的索引值,该索引值被传递到 substring()
方法中以获取文件扩展名。我们在这段代码中使用这些方法来获取文件扩展名。请看下面的例子。
public class SimpleTesting {
public static void main(String[] args) {
String fileName = "folder\s.gr\fg\student-records.pdf";
String fe = "";
if (fileName.contains("."))
fe = fileName.substring(fileName.lastIndexOf(".") + 1);
System.out.println("File extension is : " + fe);
}
}
输出:
File extension is : pdf
使用 Java 中的三元运算符获取文件扩展名
如果你对三元运算符(?, :
)很熟悉,那么就把它和 substring()
和 lastIndexOf()
方法一起使用。它减少了代码的行数,并在一条语句中返回结果。
public class SimpleTesting {
public static void main(String[] args) {
String fileName = "folder\s.gr\fg\student-records.pdf";
String fe = "";
if (fileName.contains(".")) {
int i = fileName.lastIndexOf('.');
fe = i > 0 ? fileName.substring(i + 1) : "";
}
System.out.println("File extension is : " + fe);
}
}
输出:
File extension is : pdf
在 Java 中使用 stream()
方法获取文件的文件扩展名
我们可以使用 Arrays
类的 stream()
方法将文件名转换成流,并使用 split()
方法将文件名从点(.
)中断开。请看下面的例子。
import java.util.Arrays;
public class SimpleTesting {
public static void main(String[] args) {
String fileName = "folder\s.gr\fg\student-records.pdf";
String fe = "";
if (fileName.contains(".")) {
fe = Arrays.stream(fileName.split("\\.")).reduce((a, b) -> b).orElse(null);
}
System.out.println("File extension is : " + fe);
}
}
输出:
File extension is : pdf
使用 Java 中的 Regex 获取文件的文件扩展名
这是另一个使用 regex
包的解决方案。在这个 Java 例子中,Pattern
类的 compile()
和 matcher()
方法被用来获取文件的扩展名。请看下面的例子。
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class SimpleTesting {
public static void main(String[] args) {
String fileName = "folder\s.gr\fg\student-records.pdf";
String fe = "";
final Pattern PATTERN = Pattern.compile("(.*)\\.(.*)");
Matcher m = PATTERN.matcher(fileName);
if (m.find()) {
fe = m.group(2);
}
System.out.println("File extension is : " + fe);
}
}
输出:
File extension is : pdf