Tkinter Tutorial - Message Box
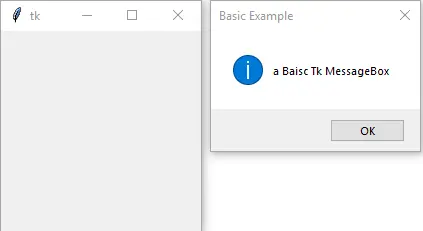
The Tkinter message box is the pop-up that appears on the screen to give you plain text information or ask the user a question like Are you sure to quit? Yes or No?
Tkinter Message Box
#!/usr/bin/python3
import tkinter as tk
from tkinter import messagebox
messagebox.showinfo("Basic Example", "a Basic Tk MessageBox")
from tkinter import messagebox
We need to import messagebox
from tkinter
.
messagebox.showinfo("Basic Example", "a Basic Tk MessageBox")
showinfo
is one of the show functions in messagebox
. It shows the information in the message box, where Basic Example
is the title in the box and a Basic Tk MessageBox
is the shown information.
The show functions in the Tkinter messagebox
are,
show Function | Description |
---|---|
showinfo |
plain infomartion |
showwarning |
warning information |
showerror |
error information |
askquestion |
ask the user question |
askokcancel |
answers are ok and cancel |
askyesno |
answers are yes and no |
askretrycancel |
answers are retry and cancel |
Tkinter Message Box Example
import tkinter as tk
from tkinter import messagebox
messagebox.showwarning("Warning Example", "Warning MessageBox")
messagebox.showerror("Error Example", "Error MessageBox")
messagebox.askquestion("Ask Question Example", "Quit?")
messagebox.askyesno("Ask Yes/No Example", "Quit?")
messagebox.askokcancel("Ask OK Cancel Example", "Quit?")
messagebox.askretrycancel("Ask Retry Cancel Example", "Quit?")
Tkinter Message Box Example in the GUI
The above examples demonstrate the message boxs to give us the first impression. But normally, the message box pops up after the user clicks a button.
We will introduce how to bind the message box with a button click and the action or command after the user clicks different options in the message box.
import tkinter as tk
from tkinter import messagebox
root = tk.Tk()
root.geometry("300x200")
def ExitApp():
MsgBox = tk.messagebox.askquestion("Exit App", "Really Quit?", icon="error")
if MsgBox == "yes":
root.destroy()
else:
tk.messagebox.showinfo("Welcome Back", "Welcome back to the App")
buttonEg = tk.Button(root, text="Exit App", command=ExitApp)
buttonEg.pack()
root.mainloop()
We construct the message box in the function ExitApp()
that is bond to the button buttonEg
.
if MsgBox == 'yes':
The return value of the clicked option is yes
or no
in askquestion
message box.
The following action could be closing the app, showing another message box or other defined behavior.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook