PyQt5 Tutorial - MenuBar
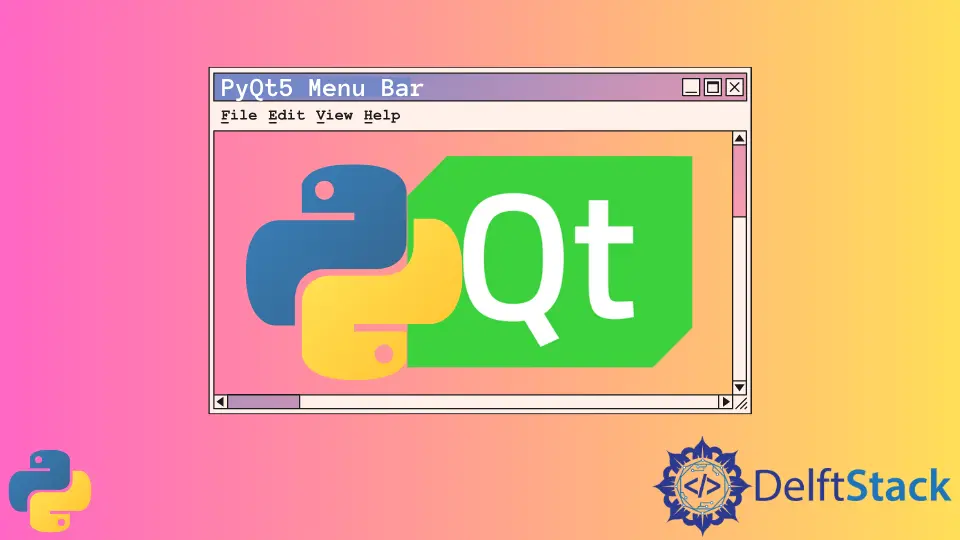
A menu bar is normally in the top left corner of the GUI and under the title bar. If the user clicks the item on the menu, it could take actions like opening files, save files or exit the application.
We will learn how to initiate a menubar, bind the action to the menu, add the shortcuts and show the status text.
PyQt5 Menubar Basic Example
We will create our first PyQt5 menubar example that has File
menu as in most editors. We only include Exit
sub-menu for simplicity.
import sys
from PyQt5.QtWidgets import QMainWindow, QAction, qApp, QApplication
class basicMenubar(QMainWindow):
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
self.initUI()
def initUI(self):
self.setGeometry(200, 200, 200, 200)
exitAction = QAction("&Exit", self)
exitAction.setShortcut("Ctrl+Q")
exitAction.setStatusTip("Exit application")
exitAction.triggered.connect(qApp.quit)
self.statusBar()
menubar = self.menuBar()
fileMenu = menubar.addMenu("&File")
fileMenu.addAction(exitAction)
self.setWindowTitle("PyQt5 Basic Menubar")
self.show()
if __name__ == "__main__":
app = QApplication(sys.argv)
ex = basicMenubar()
sys.exit(app.exec_())
menubar = self.menuBar()
QMainWindow
has the method menuBar()
to create the menubar.
fileMenu = menubar.addMenu("&File")
fileMenu.addAction(exitAction)
fileMenu
is added to the menubar with the name File
, and it consists of menu entry that associates with QAction
object - exitAction
.
exitAction = QAction("&Exit", self)
exitAction.setShortcut("Ctrl+Q")
exitAction.setStatusTip("Exit application")
exitAction.triggered.connect(qApp.quit)
It creates the QAction
object to exit the application and saves this object to the variable exitAction
.
This QAction
object has the name as &Exit
and is associated with the short cut CTRL+Q.
exitAction.setStatusTip("Exit application")
It shows the extra message in the status bar self.statusBar
when the user hovers the mouse pointer over this menu entry.
exitAction.triggered.connect(qApp.quit)
We connect the event that this exit action is triggered to the application’s quit slot.
PyQt5 Menubar Menu Item Icons
We have two methods to set the icon of the menu item in PyQt5 menu bar.
Standard Pixmap in PyQt5 Default Style
We could set the menu item icon with the standard icons by using the standard pixmap in the default style.
self.style().standardIcon(QStyle.SP_DialogCancelButton)
The above code selects the standard icon of dialog cancellation.
import sys
from PyQt5.QtWidgets import QMainWindow, QAction, qApp, QApplication, QStyle
class basicMenubar(QMainWindow):
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
self.initUI()
def initUI(self):
self.setGeometry(200, 200, 200, 200)
exitAction = QAction(
self.style().standardIcon(QStyle.SP_DialogCancelButton), "&Exit", self
)
exitAction.setShortcut("Ctrl+Q")
exitAction.setStatusTip("Exit application")
exitAction.triggered.connect(qApp.quit)
self.statusBar()
menubar = self.menuBar()
fileMenu = menubar.addMenu("&File")
fileMenu.addAction(exitAction)
self.setWindowTitle("PyQt5 Basic Menubar")
self.show()
if __name__ == "__main__":
app = QApplication(sys.argv)
ex = basicMenubar()
sys.exit(app.exec_())
Use Image File With QIcon
Besides the above method to use the default icons from QStyle
, we could also use any image file as the icon by using the QIcon
class.
QIcon("exit.png")
It sets the image file exit.png
to be the icon. The exit.png
file should be in the same folder as the Python script file.
import sys
from PyQt5.QtWidgets import QMainWindow, QAction, qApp, QApplication, QStyle
from PyQt5.QtGui import QIcon
class basicMenubar(QMainWindow):
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
self.initUI()
def initUI(self):
self.setGeometry(200, 200, 200, 200)
exitAction = QAction(QIcon("exit.png"), "&Exit", self)
exitAction.setShortcut("Ctrl+Q")
exitAction.setStatusTip("Exit application")
exitAction.triggered.connect(qApp.quit)
self.statusBar()
menubar = self.menuBar()
fileMenu = menubar.addMenu("&File")
fileMenu.addAction(exitAction)
self.setWindowTitle("PyQt5 Basic Menubar")
self.show()
if __name__ == "__main__":
app = QApplication(sys.argv)
ex = basicMenubar()
sys.exit(app.exec_())
PyQt5 Menubar Checkable Menu Item
Checkable menu item could be checked or unchecked and the state is toggled every time the user clicks it.
We need to set the keyword argument checkable
to be True
to make the menu item checkable when we create the QAction
object.
import sys
from PyQt5.QtWidgets import QMainWindow, QAction, qApp, QApplication, QLabel
from PyQt5.QtGui import QIcon
from PyQt5.QtCore import Qt
class basicMenubar(QMainWindow):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
self.setGeometry(200, 200, 200, 200)
self.label = QLabel("The toggle state is ")
self.label.setAlignment(Qt.AlignCenter)
self.setCentralWidget(self.label)
toggleAction = QAction("&Toggle Label", self, checkable=True)
toggleAction.setStatusTip("Toggle the label")
toggleAction.triggered.connect(self.toggleLabel)
exitAction = QAction(QIcon("exit.png"), "&Exit", self)
exitAction.setShortcut("Ctrl+Q")
exitAction.setStatusTip("Exit application")
exitAction.triggered.connect(qApp.quit)
self.statusBar()
menubar = self.menuBar()
fileMenu = menubar.addMenu("&File")
fileMenu.addAction(toggleAction)
fileMenu.addAction(exitAction)
self.setWindowTitle("PyQt5 Basic Menubar")
self.show()
def toggleLabel(self, state):
self.label.setText("The toggle state is {}".format(state))
if __name__ == "__main__":
app = QApplication(sys.argv)
ex = basicMenubar()
sys.exit(app.exec_())
toggleAction = QAction("&Toggle Label", self, checkable=True)
The checkable
property should be set to be True
to make the menu item checkable when we initiate the QAction
object.
Or we could use the setCheckable()
method after the object is created.
self.setCheckable(True)
The state of the checkable item is the parameter to the callback function. Therefore, we should list it in the argument when defining the function. Like,
def toggleLabel(self, state):
The state
is a boolean type, with the value as True
when selected and False
when not selected.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook