PyQt5 教程 - 菜单栏
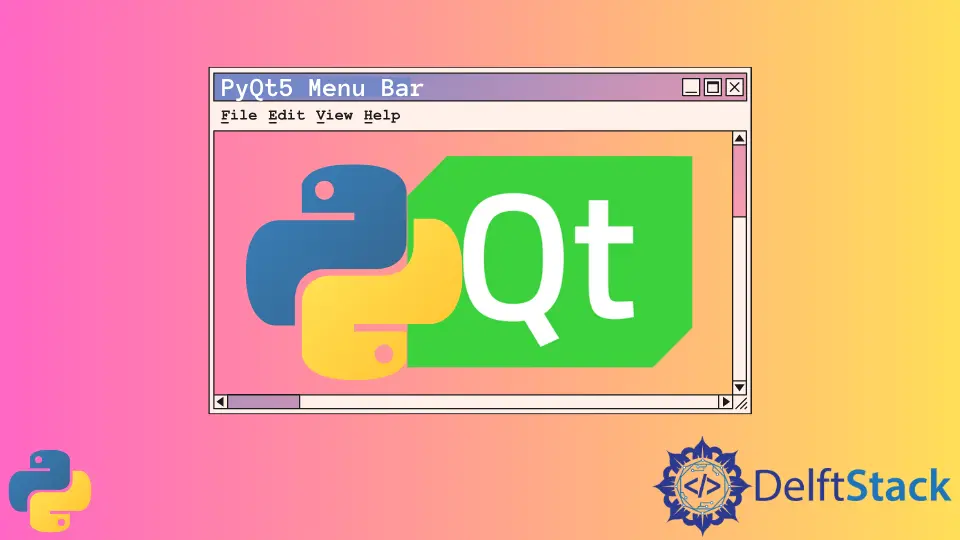
菜单栏通常位于 GUI 的左上角和标题栏下方。如果用户单击菜单上的项目,则可能会执行打开文件,保存文件或退出应用程序之类的操作。
我们将学习如何启动菜单栏,将操作绑定到菜单,添加快捷方式以及显示状态文本。
PyQt5 菜单栏基本示例
与大多数编辑器一样,我们将创建第一个具有 File
菜单的 PyQt5 菜单栏示例。为了简单起见,我们仅包含 Exit
子菜单。
import sys
from PyQt5.QtWidgets import QMainWindow, QAction, qApp, QApplication
class basicMenubar(QMainWindow):
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
self.initUI()
def initUI(self):
self.setGeometry(200, 200, 200, 200)
exitAction = QAction("&Exit", self)
exitAction.setShortcut("Ctrl+Q")
exitAction.setStatusTip("Exit application")
exitAction.triggered.connect(qApp.quit)
self.statusBar()
menubar = self.menuBar()
fileMenu = menubar.addMenu("&File")
fileMenu.addAction(exitAction)
self.setWindowTitle("PyQt5 Basic Menubar")
self.show()
if __name__ == "__main__":
app = QApplication(sys.argv)
ex = basicMenubar()
sys.exit(app.exec_())
menubar = self.menuBar()
QMainWindow
具有 menuBar()
方法来创建菜单栏。
fileMenu = menubar.addMenu("&File")
fileMenu.addAction(exitAction)
fileMenu
以名称 File
添加到菜单栏,它由与 QAction
对象-exitAction
相关联的菜单项组成。
exitAction = QAction("&Exit", self)
exitAction.setShortcut("Ctrl+Q")
exitAction.setStatusTip("Exit application")
exitAction.triggered.connect(qApp.quit)
它创建 QAction
对象以退出应用程序,并将该对象保存到变量 exitAction
中。
这个 QAction
对象的名称为&Exit
,并与快捷键 CTRL+Q 相关联。
exitAction.setStatusTip("Exit application")
当用户将鼠标指针悬停在此菜单项上时,它将在状态栏 self.statusBar
中显示额外的消息。
exitAction.triggered.connect(qApp.quit)
我们将触发此退出操作的事件连接到应用程序的退出 slot
。
PyQt5 菜单栏菜单项图标
我们有两种方法可以在 PyQt5 菜单栏中设置菜单项的图标。
PyQt5 默认样式的标准像素图
我们可以使用默认样式的标准像素图将菜单项图标设置为标准图标。
self.style().standardIcon(QStyle.SP_DialogCancelButton)
上面的代码选择对话框取消的标准图标。
import sys
from PyQt5.QtWidgets import QMainWindow, QAction, qApp, QApplication, QStyle
class basicMenubar(QMainWindow):
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
self.initUI()
def initUI(self):
self.setGeometry(200, 200, 200, 200)
exitAction = QAction(
self.style().standardIcon(QStyle.SP_DialogCancelButton), "&Exit", self
)
exitAction.setShortcut("Ctrl+Q")
exitAction.setStatusTip("Exit application")
exitAction.triggered.connect(qApp.quit)
self.statusBar()
menubar = self.menuBar()
fileMenu = menubar.addMenu("&File")
fileMenu.addAction(exitAction)
self.setWindowTitle("PyQt5 Basic Menubar")
self.show()
if __name__ == "__main__":
app = QApplication(sys.argv)
ex = basicMenubar()
sys.exit(app.exec_())
将图像文件与 QIcon
一起使用
除了上述使用 QStyle 中默认图标的方法外,我们还可以使用 QIcon
类将任何图像文件用作图标。
QIcon("exit.png")
它将图像文件 exit.png
设置为图标。exit.png
文件应与 Python 脚本文件位于同一文件夹中。
import sys
from PyQt5.QtWidgets import QMainWindow, QAction, qApp, QApplication, QStyle
from PyQt5.QtGui import QIcon
class basicMenubar(QMainWindow):
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
self.initUI()
def initUI(self):
self.setGeometry(200, 200, 200, 200)
exitAction = QAction(QIcon("exit.png"), "&Exit", self)
exitAction.setShortcut("Ctrl+Q")
exitAction.setStatusTip("Exit application")
exitAction.triggered.connect(qApp.quit)
self.statusBar()
menubar = self.menuBar()
fileMenu = menubar.addMenu("&File")
fileMenu.addAction(exitAction)
self.setWindowTitle("PyQt5 Basic Menubar")
self.show()
if __name__ == "__main__":
app = QApplication(sys.argv)
ex = basicMenubar()
sys.exit(app.exec_())
PyQt5 菜单栏可选中的菜单项
Checkable
菜单项可以选中或取消选中,并且每次用户单击它时都会切换状态。
我们需要在创建 QAction
对象时将关键字参数 checkable
设置为 True
,以使菜单项可检查。
import sys
from PyQt5.QtWidgets import QMainWindow, QAction, qApp, QApplication, QLabel
from PyQt5.QtGui import QIcon
from PyQt5.QtCore import Qt
class basicMenubar(QMainWindow):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
self.setGeometry(200, 200, 200, 200)
self.label = QLabel("The toggle state is ")
self.label.setAlignment(Qt.AlignCenter)
self.setCentralWidget(self.label)
toggleAction = QAction("&Toggle Label", self, checkable=True)
toggleAction.setStatusTip("Toggle the label")
toggleAction.triggered.connect(self.toggleLabel)
exitAction = QAction(QIcon("exit.png"), "&Exit", self)
exitAction.setShortcut("Ctrl+Q")
exitAction.setStatusTip("Exit application")
exitAction.triggered.connect(qApp.quit)
self.statusBar()
menubar = self.menuBar()
fileMenu = menubar.addMenu("&File")
fileMenu.addAction(toggleAction)
fileMenu.addAction(exitAction)
self.setWindowTitle("PyQt5 Basic Menubar")
self.show()
def toggleLabel(self, state):
self.label.setText("The toggle state is {}".format(state))
if __name__ == "__main__":
app = QApplication(sys.argv)
ex = basicMenubar()
sys.exit(app.exec_())
toggleAction = QAction("&Toggle Label", self, checkable=True)
应该将 checkable
属性设置为 True
,以使我们在启动 QAction
对象时可以选中菜单项。
或者我们可以在创建对象后使用 setCheckable()
方法。
self.setCheckable(True)
可选中项的状态是回调函数的参数。因此,在定义函数时,应在参数中列出它。比如,
def toggleLabel(self, state):
状态是布尔类型,选中时为 True
,未选中时为 False
。