How to Sort a List of Lists in Python
-
Sort a List of Lists in Python Using the
itemgetter()
Function From the Operator Module With thesorted()
Function -
Sort a List of Lists in Python Using the
lambda
Expression Along With thesorted()
Function -
Sort a List of Lists in Python Using the
sort()
Function - Sort a List of Lists in Python Using a Custom Function
- Conclusion
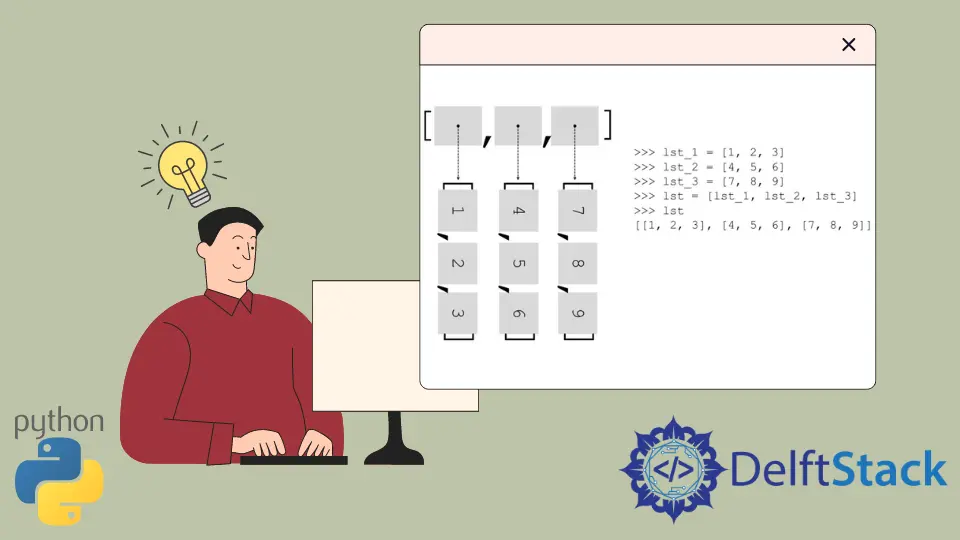
A list is one of the most powerful data structures used in Python. We can sort a list in Python by arranging all its elements in ascending or descending order based on the requirement.
We can also have nested lists in Python. These can be thought of as a list of lists. Sorting a list of lists arranges all the inner lists according to the specified index as the key.
In this tutorial, we will sort a list of lists in Python based on some indexes.
Sort a List of Lists in Python Using the itemgetter()
Function From the Operator Module With the sorted()
Function
One way to sort lists of lists is by using the itemgetter()
function from the operator
module in conjunction with the sorted()
function. This approach offers a clean and concise syntax, allowing for the sorting of a list of lists based on specific indices.
The itemgetter()
function is a key player in this sorting technique. It takes an index number as a parameter and returns a function that fetches the element at that index.
When combined with the sorted()
function, which by default sorts lists in ascending order, it provides a powerful mechanism for sorting lists of lists.
The syntax is as follows:
sorted_list = sorted(list_of_lists, key=itemgetter(index))
Here, list_of_lists
is the target list that contains sublists, and index
is the specific index within each sublist that serves as the sorting key. The resulting sorted_list
is arranged based on the specified index in ascending order.
Let’s consider a practical example to illustrate the application of itemgetter()
in sorting a list of lists. Assume we have a list A
containing sublists of integers:
from operator import itemgetter
A = [[10, 8], [90, 2], [45, 6]]
sorted_A = sorted(A, key=itemgetter(0))
print("Sorted List A based on index 0:", sorted_A)
In this code snippet, itemgetter(0)
indicates that the sorting should be performed based on the elements at index 0
of each sublist.
Output:
Sorted List A based on index 0: [[10, 8], [45, 6], [90, 2]]
In this output, the sublists are sorted in ascending order based on their first element.
Now, let’s explore another example with a list B
containing sublists of mixed data types:
from operator import itemgetter
B = [[50, "Yes"], [20, "No"], [100, "Maybe"]]
sorted_B = sorted(B, key=itemgetter(1))
print("Sorted List B based on index 1:", sorted_B)
Here, itemgetter(1)
specifies that the sorting should be based on the elements at index 1
of each sublist.
Output:
Sorted List B based on index 1: [[100, 'Maybe'], [20, 'No'], [50, 'Yes']]
This time, the sublists are sorted in ascending order based on their second element.
Sorting in Descending Order
Sorting in descending order is also a common requirement, and the itemgetter()
function, along with sorted()
, makes it straightforward. Consider a list C
with sublists containing numerical and string elements:
from operator import itemgetter
C = [[10, 8, "Cat"], [90, 2, "Dog"], [45, 6, "Bird"]]
sorted_C_desc = sorted(C, key=itemgetter(1), reverse=True)
print("Reverse sorted List C based on index 1:", sorted_C_desc)
In this example, itemgetter(1)
specifies that the sorting should be based on the elements at index 1
of each sublist. The reverse=True
parameter ensures the sorting is done in descending order.
Output:
Reverse sorted List C based on index 1: [[10, 8, 'Cat'], [45, 6, 'Bird'], [90, 2, 'Dog']]
Here, the sublists are sorted based on their second element (index 1
) in descending order.
Sort a List of Lists in Python Using the lambda
Expression Along With the sorted()
Function
In addition to the combination of itemgetter()
from the operator
module and sorted()
, Python offers an alternative method using lambda
expressions. This is a concise way to create small, anonymous functions, and it pairs seamlessly with the sorted()
function for custom sorting of lists of lists.
The lambda
expression allows us to define a one-line function directly within the sorted()
function call. The syntax is as follows:
sorted_list = sorted(list_of_lists, key=lambda x: x[index])
Here, list_of_lists
is the target list containing sublists, and the lambda x: x[index]
part defines a function that extracts the element at the specified index from each sublist. The resulting sorted_list
is arranged based on this custom function in ascending order.
Let’s dive into an example to illustrate the application of lambda
expressions in sorting a list of lists. Consider a list A
with sublists of integers:
A = [[100, "Yes"], [40, "Maybe"], [60, "No"]]
sorted_A = sorted(A, key=lambda x: x[0])
print("Sorted List A based on index 0:", sorted_A)
In this example, lambda x: x[0]
indicates that the sorting should be performed based on the elements at index 0
of each sublist.
Output:
Sorted List A based on index 0: [[40, 'Maybe'], [60, 'No'], [100, 'Yes']]
Here, the sublists are sorted in ascending order based on their first element.
Now, let’s explore another example with a list B
containing sublists of mixed data types:
B = [[2, "Dog"], [0, "Bird"], [7, "Cat"]]
sorted_B = sorted(B, key=lambda x: x[1])
print("Sorted List B based on index 1:", sorted_B)
In this case, lambda x: x[1]
specifies that the sorting should be based on the elements at index 1
of each sublist.
Output:
Sorted List B based on index 1: [[0, 'Bird'], [7, 'Cat'], [2, 'Dog']]
This time, the sublists are sorted in ascending order based on their second element.
Sorting in Descending Order
To sort in descending order, we can include the reverse=True
parameter within the sorted()
function, as demonstrated in the following example:
C = [[60, 5], [90, 7], [30, 10]]
sorted_C_desc = sorted(C, key=lambda x: x[0], reverse=True)
print("Reverse sorted List C based on index 0:", sorted_C_desc)
Here, lambda x: x[0]
signifies sorting based on the elements at index 0
of each sublist, and reverse=True
ensures the sorting is done in descending order.
Output:
Reverse sorted List C based on index 0: [[90, 7], [60, 5], [30, 10]]
Sorting Based on Multiple Criteria
In scenarios where sorting a list of lists involves multiple criteria, the lambda
expression, combined with the sorted()
function, also provides a solution. We can use the lambda
expression to create an inline function that captures the logic for sorting based on multiple criteria.
The syntax is as follows:
sorted_list = sorted(
list_of_lists, key=lambda x: (x[first_criteria], x[second_criteria], ...)
)
Here, list_of_lists
is the target list containing sublists, and the lambda
function (x[first_criteria], x[second_criteria], ...)
defines the multiple criteria for sorting. The resulting sorted_list
will be arranged based on the specified criteria in ascending order.
Let’s consider an example where we have a list D
with sublists representing books, each having attributes title
, author
, and publication_year
:
D = [
["Python Crash Course", "Eric Matthes", 2015],
["Fluent Python", "Luciano Ramalho", 2014],
["Clean Code", "Robert C. Martin", 2008],
]
sorted_books = sorted(D, key=lambda x: (x[2], x[1], x[0]))
print("Sorted Books:", sorted_books)
In this example, the lambda
function lambda x: (x[2], x[1], x[0])
is used as the key function for sorting the list of books. This function returns a tuple containing the criteria for sorting: publication_year
, author
, and title
in ascending order.
Output:
Sorted Books: [['Clean Code', 'Robert C. Martin', 2008], ['Fluent Python', 'Luciano Ramalho', 2014], ['Python Crash Course', 'Eric Matthes', 2015]]
The ability to customize sorting logic using lambda
expressions makes this approach highly adaptable and well-suited for diverse use cases.
Sort a List of Lists in Python Using the sort()
Function
The sort()
function is another method for sorting lists of lists in Python. Unlike the sorted()
function, sort()
works directly on the list itself, modifying it in place.
This method is particularly useful when the original list needs to be rearranged without creating a new sorted list.
The sort()
function is applied to the list of lists with an optional key
parameter to specify the sorting criteria. Here’s the syntax:
list_of_lists.sort(key=lambda x: x[index])
Here, list_of_lists
is the target list that contains sublists, and lambda x: x[index]
represents a custom sorting function based on the element at the specified index in each sublist. The sorting is performed in ascending order.
In order to sort in descending order, the reverse=True
parameter is added:
list_of_lists.sort(key=lambda x: x[index], reverse=True)
Let’s explore a practical example to understand how the sort()
function can be employed. Consider a list A
with sublists of integers:
A = [[55, 90], [45, 89], [90, 70]]
A.sort()
print("New sorted list A is:", A)
A.sort(reverse=True)
print("New reverse sorted list A is:", A)
In this example, A.sort()
sorts the sublists based on the first element in ascending order, and A.sort(reverse=True)
modifies the list to be sorted in descending order.
Output:
New sorted list A is: [[45, 89], [55, 90], [90, 70]]
New reverse sorted list A is: [[90, 70], [55, 90], [45, 89]]
Additionally, the key=len
parameter can be used to sort the list based on the length of the inner lists. Consider the following example:
B = [[5, 90, "Hi", 66], [80, 99], [56, 32, 80]]
B.sort(key=len)
print("New sorted list B is:", B)
In this case, the sublists in B
are sorted based on their lengths in ascending order, resulting in the output:
New sorted list B is: [[80, 99], [56, 32, 80], [5, 90, 'Hi', 66]]
The sort()
function provides a convenient way to modify the original list of lists in place, catering to scenarios where preserving the original order is not essential.
Whether it’s ascending, descending, or based on specific criteria, the sort()
function is a valuable tool for efficient list manipulation in Python.
Sort a List of Lists in Python Using a Custom Function
In certain scenarios, custom sorting logic may be required, and Python allows us to define our functions to serve as the sorting key. This approach is particularly useful when the sorting criteria are more complex or involve multiple elements within the sublists.
By providing a custom function to the sorted()
or sort()
functions, we can achieve a high degree of flexibility in sorting lists of lists.
The idea behind using a custom function is to define the logic by which the elements in the sublists should be compared. This function takes an element from the list and returns a value based on which the sorting will be done.
The syntax is as follows:
def custom_sort(item):
# custom logic to determine the sort order
return some_value
sorted_list = sorted(list_of_lists, key=custom_sort)
Here, list_of_lists
is the target list of lists, and custom_sort
is a user-defined function that encapsulates the custom sorting logic. This function is then used as the key
parameter in the sorted()
or sort()
functions.
Let’s consider an example where we want to sort a list C
based on the sum of elements in each sublist:
C = [[60, 5], [90, 7], [30, 10]]
def custom_sort_by_sum(sublist):
return sum(sublist)
sorted_C_by_sum = sorted(C, key=custom_sort_by_sum)
print("Sorted List C based on the sum of elements:", sorted_C_by_sum)
In this example, the custom_sort_by_sum
function takes a sublist and calculates the sum of its elements using sum(sublist)
. The sorted()
function then uses this custom function as the sorting key.
Output:
Sorted List C based on the sum of elements: [[30, 10], [60, 5], [90, 7]]
Whether it’s based on mathematical operations, string comparisons, or any other custom logic, using a custom function grants a high level of control over the sorting process in Python.
Conclusion
In conclusion, learning how to sort lists of lists in Python involves understanding various methods and choosing the one that best fits the specific requirements of your data. Whether it’s a simple numerical sort, complex object sorting, or multi-criteria sorting, Python provides a diverse set of tools to cater to different scenarios.
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python