How to Rename a File in Python
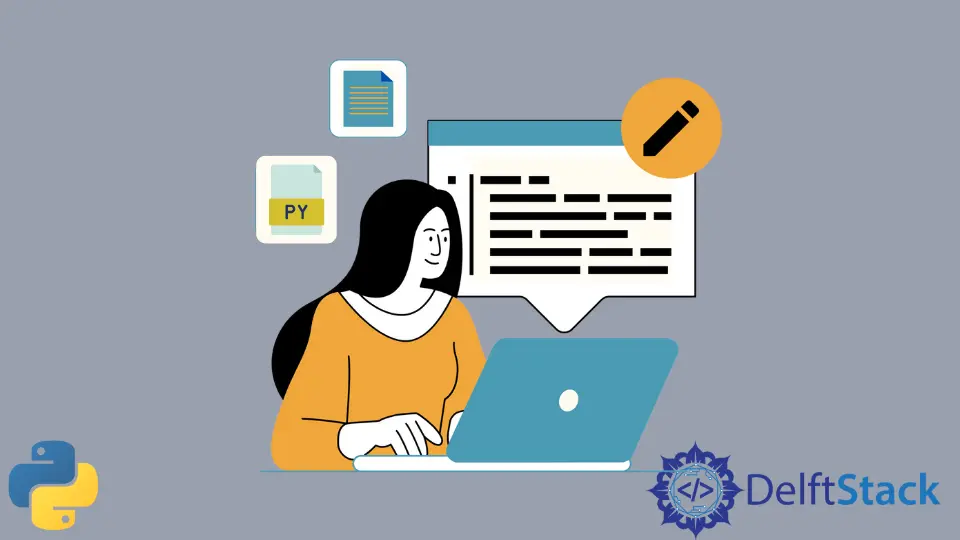
If you wish to rename a file in Python, choose one of the following options.
- Use
os.rename()
to rename a file. - Use
shutil.move()
to rename a file.
Rename a File in Python Using os.rename()
The function os.rename()
can be used to rename a file in Python.
For example,
import os
file_oldname = os.path.join("c:\\Folder-1", "OldFileName.txt")
file_newname_newfile = os.path.join("c:\\Folder-1", "NewFileName.NewExtension")
os.rename(file_oldname, file_newname_newfile)
In the above example,
file_oldname
- the old file name.
file_newname_newfile
- the new file name.
Result:
- The file named
file_oldname
is renamed tofile_newname_newfile
- The content which was present in
file_oldname
will be found infile_newname_newfile
.
Pre-requisites:
-
Import the
os
module.import os
-
Be aware of the current directory.
If the source/destination file doesn’t exist in the current directory where the code is executed, mention the absolute or relative path to the files.
-
The source file should exist. Otherwise it displays the following error.
[WinError 2] The system cannot find the file specified
-
The destination file should not exist. Else the following error is displayed -
[WinError 183] Cannot create a file when that file already exists
Rename a File in Python Using shutil.move()
The function shutil.move()
can also be used to rename a file in Python.
For example,
import shutil
file_oldname = os.path.join("c:\\Folder-1", "OldFileName.txt")
file_newname_newfile = os.path.join("c:\\Folder-1", "NewFileName.NewExtension")
newFileName = shutil.move(file_oldname, file_newname_newfile)
print("The renamed file has the name:", newFileName)
In the above example,
file_oldname
: the old file name.
file_newname_newfile
: the new file name.
Result:
- The file named
file_oldname
is renamed tofile_newname_newfile
- The content which was present in
file_oldname
will now be found infile_newname_newfile
. - The return value -
newFileName
, which is the new file name.
Pre-requisites:
-
Import the
shutil
module as,import shutil
-
Be aware of the current directory.
If the source/destination file doesn’t exist in the current directory where the code is executed, mention the absolute or relative path to the files.
-
The source file should exist. Else the following error is displayed -
[WinError 2] The system cannot find the file specified.
-
If the destination file already exists, no error is displayed. Also, if any content was present in the destination file, it is overwritten with the content in the source file.