How to Check if a String Contains Word in Python
-
Use the
if/in
Statement in Python to Check the String if It Contains a Word -
Use the
find()
Method in Python to Check the String if It Contains a Word - Use Regular Expressions in Python to Check the String if It Contains a Word
-
Use the
index()
Method With Exception Handling in Python to Check the String if It Contains a Word - Conclusion
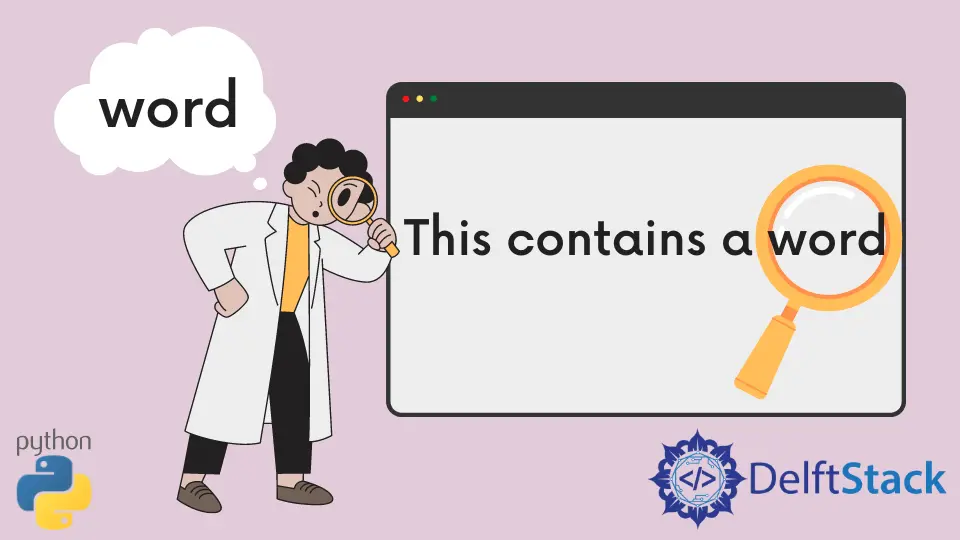
Checking if a string contains a specific word is a common task in Python, often necessary for text processing and analysis.
This tutorial will introduce various methods to find whether a specified word is inside a string variable or not in Python.
Use the if/in
Statement in Python to Check the String if It Contains a Word
If we want to check whether a given string contains a specified word in it or not, we can use the if/in
statement in Python. The if/in
statement returns True
if the word is present in the string and False
if the word is not in the string.
The following code snippet shows us how to use the if/in
statement to determine whether a string contains a word or not.
Example Code:
string = "This contains a word"
if "word" in string:
print("Found")
else:
print("Not Found")
Output:
Found
We checked whether the string variable string
contains the word word
inside it or not with the if/in
statement in the program above. This approach compares both strings character-wise; this means that it doesn’t compare whole words and can give us wrong answers, as demonstrated in the following code snippet.
Example Code:
string = "This contains a word"
if "is" in string:
print("Found")
else:
print("Not Found")
Output:
Found
The output shows that the word is
is present inside the string variable string
. But, in reality, the word is
is just a part of the first word This
in the string
variable.
This problem has a simple solution. We can surround the word and the string
variable with white spaces to compare the whole word.
Example Code:
string = "This contains a word"
if " is " in (" " + string + " "):
print("Found")
else:
print("Not Found")
Output:
Not Found
In the code above, we employ an if
statement that evaluates whether the substring " is "
is within the modified string, which includes additional white spaces on both ends. By doing so, we ensure that we are comparing complete words rather than parts of words.
This time, the output printed Not Found
because no such word as " is "
is present inside the string
variable.
Use the find()
Method in Python to Check the String if It Contains a Word
In Python, you can use the find()
method to check if a string contains a specific word or substring.
The find()
method searches for a substring (word) within a string and returns the first occurrence’s index. If the substring is not found, it returns -1
.
This approach provides a simple way to perform a containment check without explicitly using an if
statement.
Example Code 1:
my_string = "This contains a word"
if my_string.find(" is ") != -1:
print("Found")
else:
print("Not Found")
Output:
Not Found
In the first example, we have a string variable my_string
containing the phrase. We utilize the find()
method to check if the substring " is "
is present within the string.
The find()
method returns the first occurrence’s index of the substring or -1
if not found. In our case, the substring " is "
is not found within the string, so the condition evaluates to False
, and the code executes the else
block, and the output is Not Found
.
Now, let’s have another example where the substring is present within the string (my_string
).
Example Code 2:
my_string = "This contains a word"
if my_string.find("word"):
print("Found")
else:
print("Not Found")
Output:
Found
In this second example, we have a string variable my_string
containing the phrase. We use the find()
method to search for the substring "word"
within the string.
However, it’s important to note that the find()
method returns the first occurrence’s index of the substring. In this case, the substring "word"
is present at index 16
, and since it is a non-zero value, the condition evaluates to True
.
As a result, the code executes the if
block and the output is Found
. It’s crucial to be aware that this behavior may lead to unexpected outcomes, as the condition is satisfied for any non-zero index, not just when the substring is found at the beginning of the string.
Use Regular Expressions in Python to Check the String if It Contains a Word
Regular expressions (regex) can be used in Python to check if a string contains a specific word. The re
module in Python provides functions for working with regular expressions.
Specifically, you can use the re.search()
function to search for a pattern (word) within a given string. If the pattern is found, re.search()
returns a match object; otherwise, it returns None
.
Using regular expressions to check for the presence of a word in a string provides a powerful and flexible way to handle more complex patterns and conditions. However, it’s important to note that regular expressions introduce a level of complexity and might be overkill for simple cases where other string methods or functions suffice.
In the below example, \b
denotes word boundaries.
Example Code:
import re
my_string = "This contains a word"
if re.search(r"\bword\b", my_string):
print("Found")
else:
print("Not Found")
Output:
Found
In this code, we begin by importing the regular expression module (re
). We have a string variable my_string
containing the phrase.
We then use the re.search()
function to look for the pattern specified by \bword\b
, where \b
denotes word boundaries. This ensures that we match the entire word "word"
and not a part of a larger word.
As the substring (word
) is indeed present within the string, the condition evaluates to True
. Consequently, the code executes the if
block and the output is Found
.
This approach using regular expressions provides a means of accurately identifying the presence of a specific word within a string.
Use the index()
Method With Exception Handling in Python to Check the String if It Contains a Word
The index()
method is similar to find()
, but it raises a ValueError
if the substring is not found. Exception handling is used to manage the case where the substring is not present.
In Python, we can use the index()
method with exception handling to check if a string contains a specific word. The method raises a ValueError
if the word is not found, allowing you to handle the absence of the word in a controlled manner.
This approach is suitable for simple cases but introduces exception-handling overhead.
Example Code:
my_string = "This contains a word"
try:
index = my_string.index("word")
print("Found at index", index)
except ValueError:
print("Not Found")
Output:
Found at index 16
In this code, we begin by assigning the string "This contains a word"
to the variable my_string
. We then use the index()
method to find the first occurrence’s index of the substring "word"
within the string.
Since "word"
is present at index 16
in our string, the code executes the try
block, and the output is Found at index 16
. The except
block is not triggered in this case.
This method provides a precise way to identify the presence of a word and its index in the string.
Conclusion
We covered various methods to check if a specified word is present in a string in Python. We initially used the if/in
statement for simplicity but noted its potential for incorrect results due to character-wise comparison.
We then introduced the find()
method, which returns the first occurrence’s index or -1
if not found, ensuring more accurate word matching. Using regular expressions with re.search()
and \b
for word boundaries provided a powerful pattern-based approach.
Lastly, we explored the index()
method with exception handling, a robust alternative to find()
. Selecting a method depends on the specific needs of the task, each offering its advantages for string manipulation in Python.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn