How to Print Values Without Spaces in Between in Python
-
Use the String Formatting With the Modulo
%
Sign to Print Values Without Spaces in Between in Python -
Use the String Formatting With the
str.format()
Function to Print Values Without Spaces in Between in Python - Use String Concatenation to Print Values Without Spaces in Between in Python
-
Use the
f-string
for String Formatting to Print Values Without Spaces in Between in Python -
Use the
sep
Parameter of theprint
Statement to Print Values Without Spaces in Between in Python - String Interpolation Using Template Strings to Print Values Without Spaces in Between in Python
- Conclusion
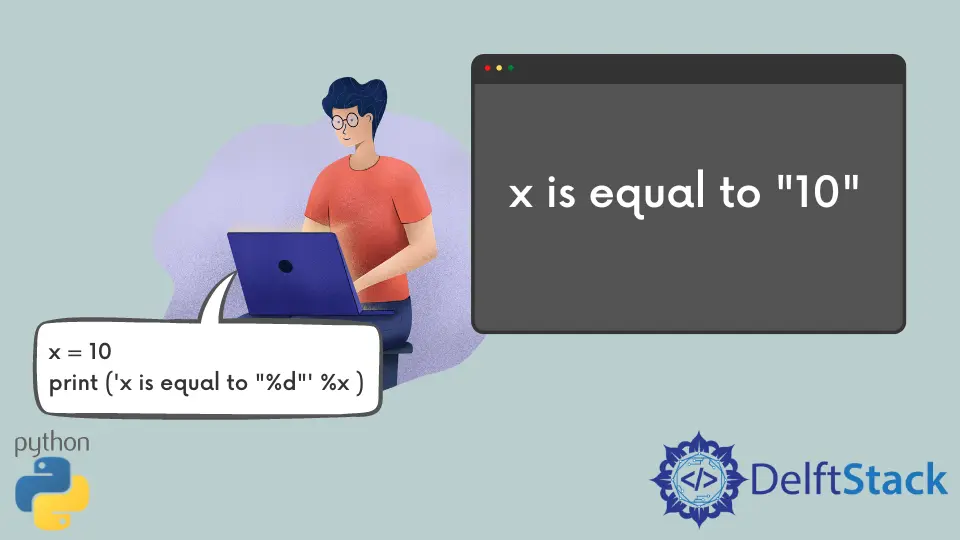
When we generally use the print
statement, we sometimes use a comma (,
) as a separator, which sometimes leads to unnecessary spaces between values. Fortunately, you can utilize some alternatives in Python that help you handle these spacing problems.
In this guide, we’ll teach you how to use the different methods to print without spaces between values in Python.
We will take a simple code for all the methods, which takes up a print
statement and contains several arguments separated by commas. For example, the following program below uses the comma operator to print the values.
x = 10
print('The number of mangoes I have are "', x, '"')
Output:
The number of mangoes I have are " 10 "
We should note that there are unnecessary spaces between the number 10
and the double quotes surrounding it. The aim is to prevent or remove this excessive or unnecessary spacing.
Use the String Formatting With the Modulo %
Sign to Print Values Without Spaces in Between in Python
String formatting gives more customization options to the users to go with the classic print
statement. The %
sign is also known as an interpolation or a string formatting operator.
String formatting can be implemented in two ways, and using the %
sign is one of those options.
The %
sign, followed by a letter that represents the conversion type, works as a placeholder for the variable. The code below uses the %
sign to print without spaces between values in Python.
x = 10
print('The number of mangoes I have are "%d"' % x)
Output:
The number of mangoes I have are "10"
In this code snippet, we initialize a variable x
with the value 10
. Then, we use the print
function to display a message.
Inside the message, we have a placeholder %d
, which is used for formatting the string. The %d
placeholder is replaced by the value of the variable x
when the string is printed.
So, when the code is executed, it prints the following message: The number of mangoes I have are "10"
. Here, the %d
is replaced by the value of x
, which is 10
, resulting in the final output displayed in the console.
Use the String Formatting With the str.format()
Function to Print Values Without Spaces in Between in Python
When using the string formatting, braces {}
are used to mark the spot in the statement where the variable would be substituted.
The str.format()
has been introduced in Python 3 and is available to use in the latest versions of Python. This function is utilized for the efficient handling of complex string formatting.
The following code uses the str.format()
function to print without spaces between values in Python.
x = 10
print('The number of mangoes I have are "{}"'.format(x))
Output:
The number of mangoes I have are "10"
In this code, we begin by assigning a value of 10
to the variable x
. Following that, we use the print
function to output a message.
Within the message, we have a placeholder {}
enclosed in double quotes. We use the format
method to substitute the placeholder with the value of the variable x
when the string is displayed.
Consequently, when we execute the code, it produces the following output: The number of mangoes I have are "10"
. Here, the {}
is replaced by the value of x
, which is 10
, leading to the final output exhibited in the console.
It is recommended to use the format()
function instead of the old %
operator in the newer versions of Python.
Use String Concatenation to Print Values Without Spaces in Between in Python
The +
operator, also known as the string concatenation operator, can be used in this case to prevent unnecessary spacing between the values. It’s a direct alternative to comma separation and can be used along with the print
statement.
Here is an example code that shows the use of string concatenation in the print statement.
x = 10
print('The number of mangoes I have are "' + str(x) + '"')
Output:
The number of mangoes I have are "10"
In this code, we initiate a variable x
and assign it a value of 10
. Subsequently, we use the print
function to display a message.
Inside the message, we have a string containing double quotes, and we concatenate the value of the variable x
to this string. To ensure the concatenation works correctly, we convert the integer value of x
to a string using the str()
function.
As a result, when we execute the code, it generates the following output: The number of mangoes I have are "10"
. Here, we have successfully combined the string with the value of x
as a string, resulting in the final output displayed in the console.
Use the f-string
for String Formatting to Print Values Without Spaces in Between in Python
Python 3.6 introduced the f-string
, which is another method of achieving string formatting; however, it has the edge over the two other processes for string formatting mentioned above because it’s comparatively faster than its other two peers.
The following code uses the fstring
formatting to print without spaces between values in Python.
x = 10
print(f'The number of mangoes I have are "{x}"')
Output:
The number of mangoes I have are "10"
In this code, we begin by initializing a variable x
with a value of 10
. Next, we use the print
function to display a message.
Within this message, we utilize an f-string
, denoted by the f
prefix before the string. Inside the f-string
, we include curly braces {}
with the variable x
placed inside them.
When the code is executed, Python automatically substitutes the value of the variable x
within the curly braces and displays the string. As a result, the output is: The number of mangoes I have are "10"
.
Using an f-string
in this manner simplifies the process of incorporating variables into strings, making the code more concise and readable.
Use the sep
Parameter of the print
Statement to Print Values Without Spaces in Between in Python
You can modify the spacing between the arguments of the print
statement by using the sep
parameter.
The sep
parameter can only be found and used in Python 3 and later versions. It can also be utilized for the formatting of the output strings.
The following code uses the sep
parameter to print without spaces between values in Python.
x = 10
print('The number of mangoes I have are "', x, '"', sep="")
Output:
The number of mangoes I have are "10"
In this code, we start by creating a variable x
and assigning it a value of 10
. Subsequently, we use the print
function to display a message.
Within the message, we have a string that is broken into multiple parts. We have the text "The number of mangoes I have are"
followed by a comma, then the variable x
, followed by another comma, and finally, double quotes "
surrounding x
.
To ensure that there are no extra spaces or separators between these parts when they are printed, we set the sep
parameter to an empty string ""
. When the code is executed, Python combines these parts to form the final output: The number of mangoes I have are "10"
.
The sep
parameter helps us control how the different parts are separated in the output. In this case, by setting it to an empty string, we achieve the desired format without any extra spaces or separators.
String Interpolation Using Template Strings to Print Values Without Spaces in Between in Python
Python’s string.Template
can be used to interpolate values into a template string. It allows you to specify placeholders and then substitute values without adding spaces.
Here is an example code that shows the use of string.Template
.
from string import Template
x = 10
template = Template('The number of mangoes I have are "$x"')
result = template.substitute(x=x)
print(result)
Output:
The number of mangoes I have are "10"
In this code, we start by importing the Template
class from the string
module. We then create a variable x
and assign it the value 10
.
Next, we define a template string using the Template
class, where we have a placeholder $x
representing the value of the variable x
. The template string is: 'The number of mangoes I have are "$x"'
.
Moving forward, we use the substitute()
method of the Template
class to substitute the value of the variable x
into the placeholder $x
. When we call template.substitute(x=x)
, Python replaces $x
with the value of x
, which is 10
in this case, and as a result, the template string is formatted to: 'The number of mangoes I have are "10"'
.
Finally, we print the formatted string using the print()
function. When the code is executed, it produces the following output: 'The number of mangoes I have are "10"'
.
Here, the placeholder $x
has been successfully replaced by the value of the variable x
, resulting in the final output displayed in the console.
Conclusion
The article discussed different methods for printing values in Python without introducing unwanted spaces. It begins by addressing the common problem of unnecessary spaces caused by using commas as separators in the print
statement.
The discussed alternatives include string formatting with the modulo %
sign, which utilizes placeholders to format strings, and string formatting with str.format()
, employing curly braces for efficient variable substitution. Another approach involves string concatenation using the +
operator, ensuring the combination of strings and variables does not introduce extra spaces.
The article also introduces f-strings
, a concise and faster method introduced in Python 3.6 for string formatting. Additionally, the sep
parameter in Python 3 allows developers to control the spacing between printed values.
Furthermore, the article introduces string interpolation using string.Template
, providing precise variable substitution without adding spaces. These methods equip Python developers with diverse tools to handle spacing issues, ensuring clean and professional code formatting tailored to specific preferences and requirements.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn