How to Create a Countdown Timer in Python
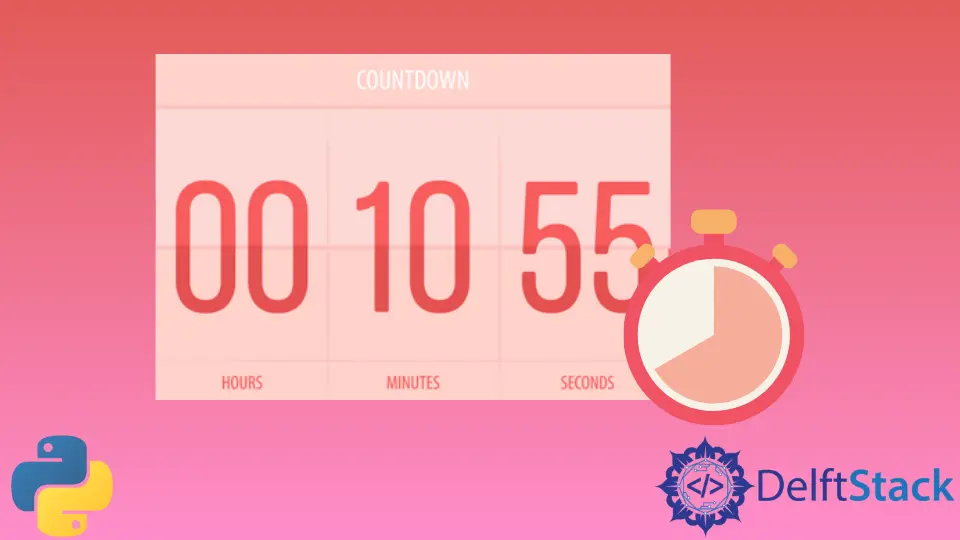
This tutorial introduces how to create a countdown timer in Python.
The code accepts an input of how long the countdown should be and will start the countdown immediately after the input is entered.
Using the time
Module and the sleep()
Function to Create a Countdown Timer in Python
The time
module is a general Python module containing time-related utility functions and variables. The main function used in this tutorial is the sleep()
function, which is an asynchronous function that suspends the execution of a single thread for n
seconds.
If your program is single-threaded, which is the case for this tutorial, then the sleep()
function will stop the whole program from running until the elapsed time given has been reached. With this, along with validated user input, we can create a simple countdown timer in Python.
The first thing to do is import the time
module to use the sleep()
function.
import time
Then declare a function to act as the countdown timer. Let’s call this function countdown()
. The function will accept a single parameter: the number of seconds (num_of_secs
) the timer will count down to.
The num_of_secs
variable will be continuously decremented in a loop until it reaches 0
(which translates to False
and ends the loop without any extra condition).
Within the loop, format the input variable num_of_secs
into MM:SS
format and print it out every time it decrements. To do this, use the built-in Python function divmod()
, which accepts two numbers and returns the product and the remainder of the two numbers, respectively. Then format the tuple result of divmod()
into MM:SS
format using the built-in string function format()
.
def countdown(num_of_secs):
while num_of_secs:
m, s = divmod(num_of_secs, 60)
min_sec_format = "{:02d}:{:02d}".format(m, s)
{:02d}
formats the argument into a 2-digit integer (because of the symbol 02d
). If the integer is less than 2 digits, it will add leading 0
’s to it.
Next, with each iteration of the loop, call time.sleep(1)
, which means it delays each iteration for 1 second and will continue after elapsing.
Before calling the sleep()
function, print out the formatted string that resembles the MM:SS
format of the current value of the input variable num_of_secs
.
Also, add another argument within the print()
function with the property end
and the value /r
, which is a new line to imitate the behavior of an actual timer. This argument overwrites the previous print()
output each time the loop is executed, overwriting everything before the carriage return is denoted by the symbol /r
.
def countdown(num_of_secs):
while num_of_secs:
m, s = divmod(num_of_secs, 60)
min_sec_format = "{:02d}:{:02d}".format(m, s)
print(min_sec_format, end="/r")
time.sleep(1)
num_of_secs -= 1
print("Countdown finished.")
Afterward, decrement the input variable min_sec_format
by 1 after all the prior lines have been executed.
Finally, print out a concluding statement outside the loop that denotes that the timer is done executing. It concludes the countdown()
method and will function as a countdown timer.
The next thing to do is provide a way for the user to input the number of seconds to trigger the countdown. For this, we can use the built-in input()
function to accept user input.
Catch the input into a variable and use it as an argument for the countdown function. Make sure to cast the input variable to int
for validation.
inp = input("Input number of seconds to countdown: ")
countdown(int(inp))
The entire code should look like this:
import time
def countdown(num_of_secs):
while num_of_secs:
m, s = divmod(num_of_secs, 60)
min_sec_format = "{:02d}:{:02d}".format(m, s)
print(min_sec_format, end="/r")
time.sleep(1)
num_of_secs -= 1
print("Countdown finished.")
inp = input("Input number of seconds to countdown: ")
countdown(inp)
Output:
Countdown finished.
The final output would display Countdown Finished
, but will imitate how a timer works and clear each print()
line until it reaches 00:00
.
So if you’d input 5
seconds, the stack trace would look like this:
00:05
00:04
00:03
00:02
00:01
Countdown finished.
There you have it. You now have successfully created a simple countdown timer in Python using just built-in functions and the sleep()
function from the time
module.
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedIn