Timer Functions in Python
-
Use of
time.time()
Function in Python -
Use of
time.Process_time()
Function in Python -
Use of
time.Perf_counter
Function in Python -
Use of
time.monotonic()
Function in Python
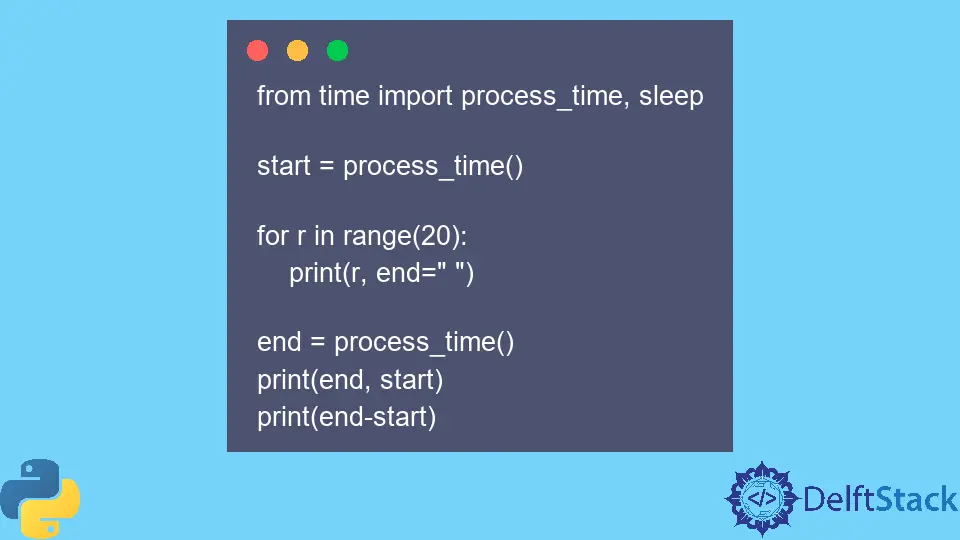
Python is a very vast programming language that has very high usage all over the world. Programmers make numerous programs that require a considerable amount of lines of python code. To monitor and analyze those codes based on running time, we can use Python timer functions.
The time
module is the most important here as it contains all the functions that help keep a check of the time and analyze it.
In this tutorial, we will discuss various Python Timer functions using the time module.
Use of time.time()
Function in Python
This function returns time in seconds. It is the passing seconds after the epoch - 1st January 1970, 00:00:00 (UTC). This function uses the time set of the computer system to return the output, i.e., the number of seconds.
Example:
import time
start = time.time()
for r in range(1, 20000):
pass
end = time.time()
print(format(end - start))
In between start
and end
, comes the main body of the code. Here, a for
loop is taken as an example.
Output:
3.252345085144043
Note that output i.e seconds is a float value.
Use of time.Process_time()
Function in Python
This function returns time in fractional seconds. Time reference of the whole process is also recorded in the function and not only the time elapsed during the process.
Example:
from time import process_time, sleep
start = process_time()
for r in range(20):
print(r, end=" ")
end = process_time()
print(end, start)
print(end - start)
Output:
0 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 10.756645 10.75523
0.0014150000000014984
Time taken in time.sleep()
is not measured by this function which means it only measures the time difference between two consecutive time references matters.
Use of time.Perf_counter
Function in Python
Also known as Performance Counter, this function helps get the time count between two references in a more accurate manner. This function should only be applied to small processes as it is highly accurate.
We can also use time.sleep()
in between this function. By this function, the execution of the code can be suspended for several seconds. sleep()
function takes a float value as an argument.
Example:
from time import perf_counter, sleep
n = 10
start = perf_counter()
for r in range(n):
sleep(2)
end = perf_counter()
print(end - start)
Output:
20.03540569800043
Returned value shows the total time elapsed. As the sleep
function is set at 2, it took 20.035 seconds to complete the whole process where the input value was 10.
Use of time.monotonic()
Function in Python
If the user changes the time while executing a python code, it can make a huge difference when implementing the timer function in Python. In this situation, the monotonic timer function makes sure that time references adapt themselves according to changes made by the user externally.
Example:
from time import monotonic, sleep
n = 10
start = monotonic()
for r in range(n):
sleep(2)
end = monotonic()
print(end - start)
Output:
20.029595676999634
The start and the end references ensure that the program adapts itself to any change made by the user.
Lakshay Kapoor is a final year B.Tech Computer Science student at Amity University Noida. He is familiar with programming languages and their real-world applications (Python/R/C++). Deeply interested in the area of Data Sciences and Machine Learning.
LinkedIn