在 Python 中建立倒數計時器
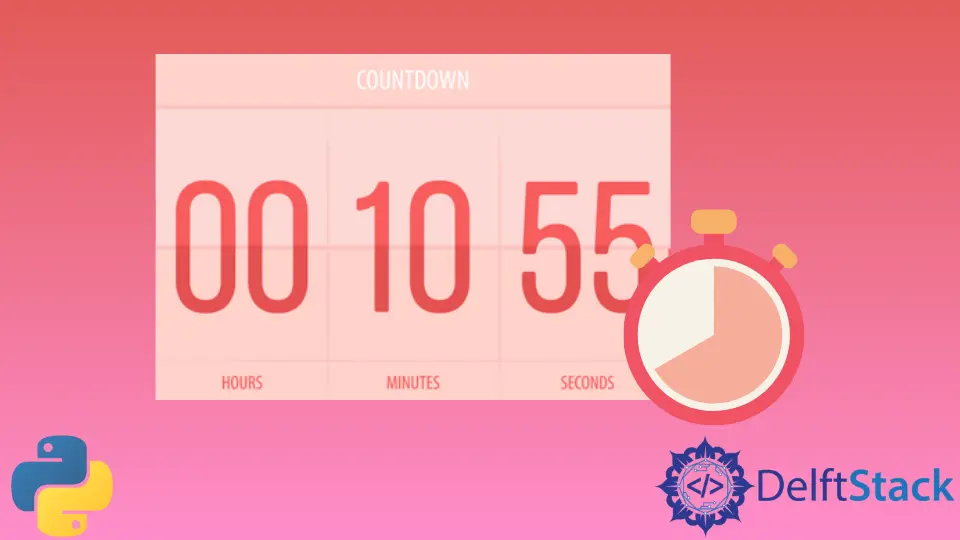
本教程介紹如何在 Python 中建立倒數計時器。
該程式碼接受倒計時應該多長時間的輸入,並在輸入後立即開始倒計時。
在 Python 中使用 time
模組和 sleep()
函式建立倒數計時器
time
模組是一個通用的 Python 模組,包含與時間相關的實用函式和變數。本教程中使用的主要函式是 sleep()
函式,它是一個非同步函式,可將單個執行緒的執行暫停 n
秒。
如果你的程式是單執行緒的,這就是本教程的情況,那麼 sleep()
函式將停止整個程式執行,直到達到給定的經過時間。有了這個,連同經過驗證的使用者輸入,我們可以在 Python 中建立一個簡單的倒數計時器。
首先要做的是匯入 time
模組以使用 sleep()
函式。
import time
然後宣告一個函式作為倒數計時器。我們稱這個函式為 countdown()
。該函式將接受一個引數:計時器將倒計時的秒數(num_of_secs
)。
num_of_secs
變數將在迴圈中不斷遞減,直到達到 0
(轉換為 False
並在沒有任何額外條件的情況下結束迴圈)。
在迴圈中,將輸入變數 num_of_secs
格式化為 MM:SS
格式,並在每次遞減時將其列印出來。為此,請使用 Python 內建函式 divmod()
,該函式接受兩個數字並分別返回乘積和兩個數字的餘數。然後使用內建字串函式 format()
將 divmod()
的元組結果格式化為 MM:SS
格式。
def countdown(num_of_secs):
while num_of_secs:
m, s = divmod(num_of_secs, 60)
min_sec_format = "{:02d}:{:02d}".format(m, s)
{:02d}
將引數格式化為 2 位整數(因為符號 02d
)。如果整數小於 2 位,它將新增前導 0
。
接下來,在迴圈的每次迭代中,呼叫 time.sleep(1)
,這意味著它會將每次迭代延遲 1 秒,並在經過後繼續。
在呼叫 sleep()
函式之前,列印出類似於輸入變數 num_of_secs
的當前值的 MM:SS
格式的格式化字串。
此外,在 print()
函式中新增另一個引數,其屬性為 end
,值為 /r
,這是一個模擬實際計時器行為的新行。每次執行迴圈時,此引數都會覆蓋之前的 print()
輸出,覆蓋由符號 /r
表示的回車之前的所有內容。
def countdown(num_of_secs):
while num_of_secs:
m, s = divmod(num_of_secs, 60)
min_sec_format = "{:02d}:{:02d}".format(m, s)
print(min_sec_format, end="/r")
time.sleep(1)
num_of_secs -= 1
print("Countdown finished.")
之後,在所有前面的行都執行完後,將輸入變數 min_sec_format
減 1。
最後,在迴圈外列印出一個結束語句,表示計時器已完成執行。它結束了 countdown()
方法並將用作倒數計時器。
接下來要做的是為使用者提供一種方法來輸入觸發倒計時的秒數。為此,我們可以使用內建的 input()
函式來接受使用者輸入。
將輸入捕獲到變數中並將其用作倒計時函式的引數。確保將輸入變數轉換為 int
以進行驗證。
inp = input("Input number of seconds to countdown: ")
countdown(int(inp))
整個程式碼應該是這樣的:
import time
def countdown(num_of_secs):
while num_of_secs:
m, s = divmod(num_of_secs, 60)
min_sec_format = "{:02d}:{:02d}".format(m, s)
print(min_sec_format, end="/r")
time.sleep(1)
num_of_secs -= 1
print("Countdown finished.")
inp = input("Input number of seconds to countdown: ")
countdown(inp)
輸出:
Countdown finished.
最終輸出將顯示 Countdown Finished
,但會模仿計時器的工作方式並清除每個 print()
行,直到到達 00:00
。
因此,如果你輸入 5
秒,堆疊跟蹤將如下所示:
00:05
00:04
00:03
00:02
00:01
Countdown finished.
你有它。你現在已經成功地使用 Python 中的內建函式和 time
模組中的 sleep()
函式成功地建立了一個簡單的倒數計時器。
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedIn