How to Convert Bytearray to String in Python
-
Convert
bytearray
tostring
With thebytes()
Function in Python -
Convert
bytearray
tostring
With thebytearray.decode()
Function in Python
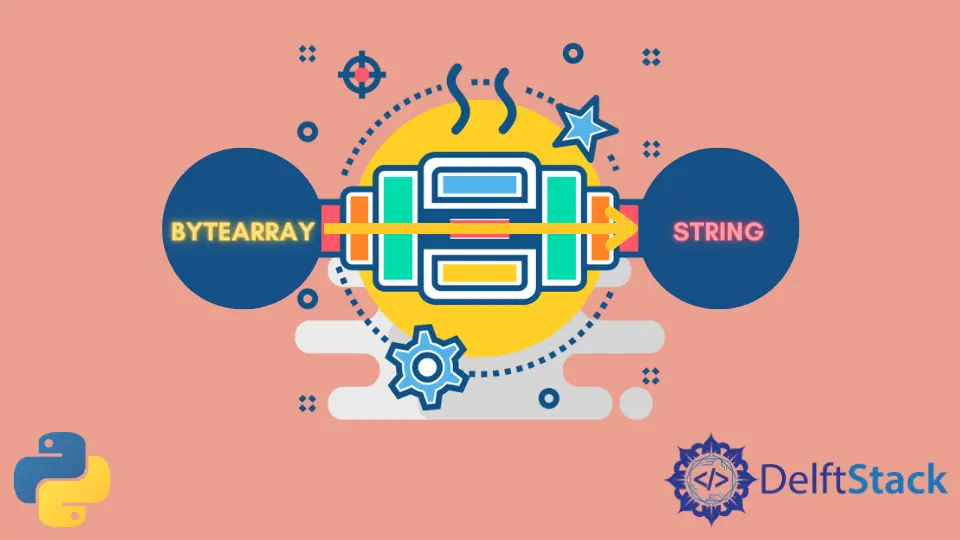
You can use two primary methods to convert a bytearray
into a String
in Python: bytes()
and bytearray.decode()
.
In this tutorial, we’ll show you how you can use these functions as methods for this special conversion.
Convert bytearray
to string
With the bytes()
Function in Python
If we have a bytearray
containing string characters with the utf-8
encoding and want to convert that array into a string
variable, we can use the built-in bytes()
function in Python.
The bytes()
function returns an immutable bytes object that can then be stored inside a string
variable. The following code snippet demonstrates how we can convert a bytearray
to a string
with the bytes()
function.
b = bytearray("test", encoding="utf-8")
str1 = bytes(b)
print(str1)
Output:
b"test"
We converted the bytearray object b
into a string variable str1
with the bytes()
function in the code above. First, we encoded the text test
with a utf-8
encoding inside an object of bytearray
. We then converted the bytearray
to string
with the bytes()
function and stored the result inside the string variable str1
.
In the end, we printed the data inside the str1
variable. The output shows that this process adds a b
object at the start of our original data and then encloses the data inside single quotes. This problem is addressed in the method discussed next.
Convert bytearray
to string
With the bytearray.decode()
Function in Python
As we can see, the bytes()
function converts a bytearray
to a string
but adds additional data to the original string. This problem can be solved by string manipulation, but it is a cumbersome process. The bytearray.decode()
function automatically does that for us. This method decodes the data originally encoded inside the bytearray
.
The following code snippet demonstrates how we can convert a bytearray
to string
with the bytearray.decode()
function.
b = bytearray("test", encoding="utf-8")
str1 = b.decode()
print(str1)
Output:
test
We converted the bytearray object b
into a string variable str1
with the b.decode()
function in the code above. First, we encoded the text test
with a utf-8
encoding inside an object of bytearray
. We then converted the bytearray
to string
with the b.decode()
function and stored the result inside the string variable str1
. In the end, we printed the data inside the str1
variable. The output shows that this process does not add any additional data to our originally encoded data.
From the demonstrations above, it is clear that the bytearray.decode()
method is far superior to the byte()
method for converting a bytearray
object into a string
variable.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn