How to Concatenate List of String in Python
-
Use the
join()
Method to Convert the List Into a Single String in Python -
Use the
map()
Function to Convert the List of Any Data Type Into a Single String in Python -
Use the
for
Loop to Convert List Into a Single String in Python
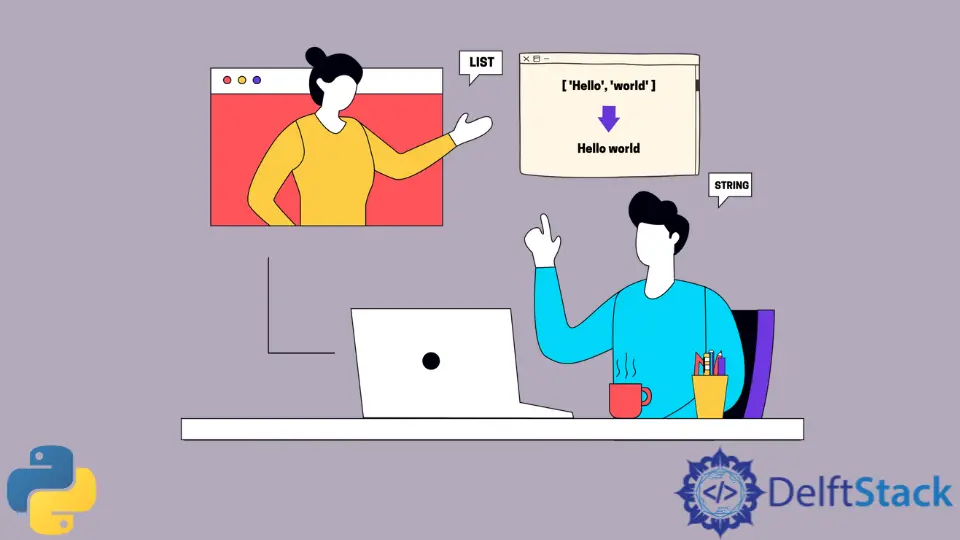
This article will introduce methods to concatenate items in the Python list to a single string.
Use the join()
Method to Convert the List Into a Single String in Python
The join()
method returns a string in which the string separator joins the sequence of elements. It takes iterable data as an argument.
This method can be visualized as follow:
"separator".join(["List", "of", " string"])
We call the join()
method from the separator
and pass a list of strings as a parameter. It returns the string accordingly to the separator being used. If a newline character \n
is used in the separator, it will insert a new line for each list element. If one uses a comma ,
in the separator, it simply makes a commas-delimited string. The join()
method returns a string in an iterable. A TypeError
will be raised if any non-string values are iterable, including byte objects. An expression called generator expression is used to have all data types work for it.
For example, create a variable words_list
and write some list elements on it. They are Joey
, doesnot
, share
and food
. Use a separator " "
to call the join()
method. Use the words_list
variable as the argument in the function. Use the print()
function on the whole expression.
In the example below, the join()
function takes the words_list
variable as an argument. Then, the separator " "
is inserted between each list element. Finally, as an output, it returns the string Joey does not share food
.
Example Code:
# python 3.x
words_list = ["Joey", "doesnot", "share", "food"]
print(" ".join(words_list))
Output:
Joey doesnot share food
Use the map()
Function to Convert the List of Any Data Type Into a Single String in Python
The map()
function applies a specific function passed as an argument to an iterable object like list and tuple. The function is passed without calling it. It means there are no parentheses in the function. It seems the map()
function would be a more generic way to convert python lists to strings.
This can be visualized as :
data: d1, d2, d3, .... dn
function: f
map(function, data):
returns iterator over f(d1), f(d2), f(d3), .... f(dn)
For example, create a variable word_list
and store some list items into it. They are Give
, me
, a
, call
, at
and 979797
. Then, write a map()
function and pass a function str
and a variable words_list
as arguments to the map()
function. Write a join()
function and take the map
object as its argument. Use an empty string " "
to call the join()
function. Print the expression using the print()
funtion.
The str
function is called to all list elements, so all elements are converted to the string type. Then, space " "
is inserted between each map object, and it returns the string as shown in the output section.
# python 3.x
words_list = ["Give", "me", "a", "call", "at", 979797]
print(" ".join(map(str, words_list)))
Output:
Give me a call at 979797
Use the for
Loop to Convert List Into a Single String in Python
We can use the for
loop to get a single string from the list. In this method, we iterate over all the values, then append each value to an empty string. It is a straightforward process but takes more memory. We add a separator alongside the iterator to append in an empty string.
For example, create a variable words_list
and store the list items. Next, create an empty string sentence
. Use the for
loop and use the variable word
as an iterator. Use the str()
method on the word
and add it to the variable sentence
. Then, add a "."
as the string to the function. After that, assign the expression to the variable sentence
. Print the variable outside the loop.
In this example, the python list words_list
contains a list of elements. The empty string variable sentence
is used to append list elements on looping. Inside the loop, the str()
method typecasts the elements to string, and "."
acts as a separator between each iterable item which gets appended to the empty string sentence
.
Example Code :
# python 3.x
words_list = ["Joey", "doesnot", "share", "food"]
sentence = ""
for word in words_list:
sentence += str(word) + "."
print(sentence)
Output:
Joey.doesnot.share.food