Multiple Inheritance in Python
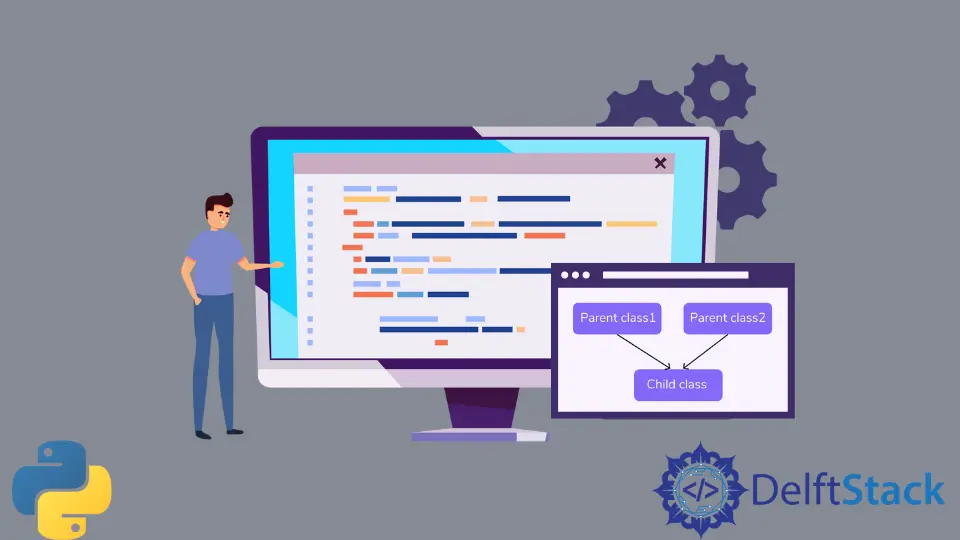
Inheritance allows us to use parent class features in a child class and is an essential feature of object-oriented programming. It helps with the reusability and transitivity of data from the parent class to the child class and compensates for data loss.
Multiple inheritance is when the child class inherits the methods and features from two or more parent classes. It is beneficial to derive all data at once. Still, on the other hand, it comes with usage complexity and ambiguity. It is ambiguous to tell which feature is from which parent if multiple parents possess the same feature. It occurs when multiple inheritance is not used or implemented correctly. Virtual inheritance, use of Method Resolution Order (MRO), and super()
function can somehow reduce its risks.
We see a basic example of multiple inheritances in the following code.
class Father:
def drive(self):
print("Father drives his son to school")
class Mother:
def cook(self):
print("Mother loves to cook for her son")
class Son(Father, Mother):
def love(self):
print("I love my Parents")
c = Son()
c.drive()
c.cook()
c.love()
Output:
Father drives his son to school
Mother loves to cook for her son
I love my parents
The child class Son
is derived from the parent classes Father
and Mother
, which allows it to use the functions drive()
and cook()
to give the desired output.
The super()
function refers to the parent or sibling class in the inherited child class and returns a temporary object that allows the child class to use all the superclass methods.
This is usually done in case of ambiguity when the inheritance starts crossing paths, i.e., when the two parent classes are also derived from the super base class.
For Example,
class Animals:
def __init__(self, animalsName):
print(animalsName, "is an animal.")
class Mammal(Animals):
def __init__(self, Name):
print(Name, "is a mammal.")
super().__init__(Name)
class donotFly(Mammal):
def __init__(self, name):
print(name, "cannot fly.")
super().__init__(name)
class donotSwim(Mammal):
def __init__(self, name):
print(name, "cannot swim.")
super().__init__(name)
class Cat(donotSwim, donotFly):
def __init__(self):
print("A cat.")
super().__init__("Cat")
cat = Cat()
print("")
bat = donotSwim("Bat")
Output:
A cat.
Cat cannot swim.
Cat cannot fly.
Cat is a mammal.
Cat is an animal.
Bat cannot swim.
Bat is a mammal.
Bat is an animal.
A child class Cat
is created, inherited by the two-parent classes donotswim
and donotfly
. Then, the Mammals class inherits them themselves. The Mammals
class furthermore inherits the properties of the superclass Animals
. Therefore, in this case, we used the super()
function to easily access the superclass methods.