How to Convert a Map Object Into a List in Python
-
Use the
list()
Method to Convert a Map Object Into a List in Python - Use the List Comprehension Method to Convert a Map Object to a List in Python
-
Use the Iterable Unpacking Operator
*
to Convert a Map Object Into a List in Python
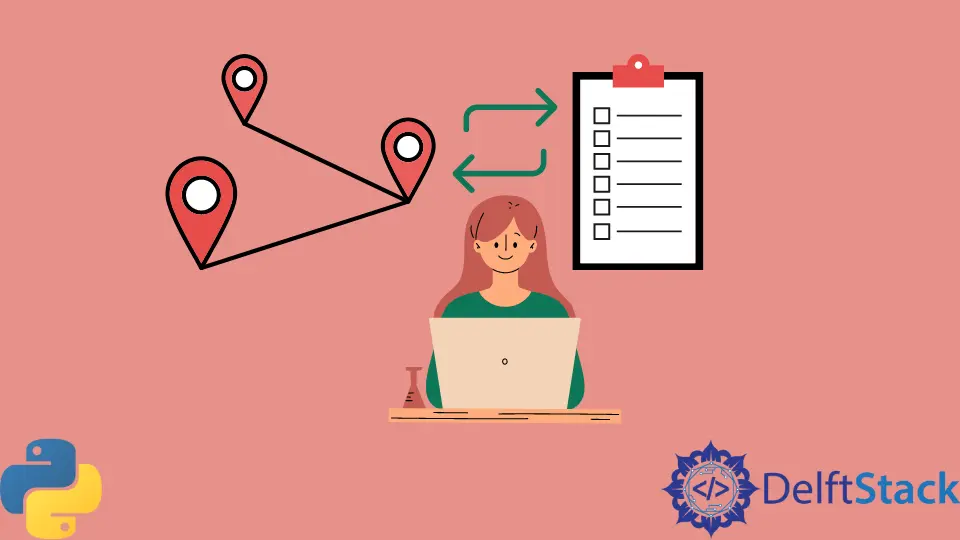
Python provides a map()
function, which you can utilize to apply a particular function to all the given elements in any specified iterable. This function returns an iterator itself as the output. It is also possible to convert map objects to the sequence objects like tuple and list by utilizing their own factory functions.
This tutorial will discuss and demonstrate the different methods you can use to convert a map object into a list in Python.
Use the list()
Method to Convert a Map Object Into a List in Python
Lists are part of the four built-in datatypes provided in Python and can be utilized to stock several items in a single variable. Lists are ordered, changeable, and have a definite count.
The list()
function is used to create a list object in Python. This method is used to convert a specific tuple into a list. The following code uses the list()
method to convert a map object into a list in Python:
a = list(map(chr, [70, 50, 10, 96]))
print(a)
Output:
['F', '2', '\n', '`']
Numerous processes that operate or execute over the iterables return iterators themselves in Python 3; this simplifies the language even more. This also leads to a better and more efficient program run.
Use the List Comprehension Method to Convert a Map Object to a List in Python
The list comprehension method is a relatively shorter and very graceful way to create lists formed on the basis of given values of an already existing list. This method can be used in this case, along with a simple iteration to create a list from the map object.
The program below uses this method to convert a map object into a list in Python:
a = [chr(i) for i in [70, 50, 10, 96]]
print(a)
Output:
['F', '2', '\n', '`']
Use the Iterable Unpacking Operator *
to Convert a Map Object Into a List in Python
In Python, the term unpacking
can be defined as an operation whose primary purpose is to assign the iterable with all the values to a List or a Tuple, provided it’s done in a single assignment statement.
The star *
sign is used as the iterable unpacking operator. The iterable unpacking operator can work efficiently and excellently for both Tuples and List.
The following code uses the iterable unpacking operator *
to convert a map object into a list in Python:
a = [*map(chr, [70, 50, 10, 96])]
print(a)
Output:
['F', '2', '\n', '`']
In most cases, this method is more efficient than the other two. Still, with a very small margin as the iterable unpacking operator, the *
method is shorter by just a single character from the list comprehension method. All three methods work perfectly fine, and you can use any of these methods in regular everyday programming.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python