How to Loop Over a String in Python
- Method 1: Using a Simple For Loop
-
Method 2: Using the
while
Loop - Method 3: Using List Comprehension
- Method 4: Using the Enumerate Function
- Conclusion
- FAQ
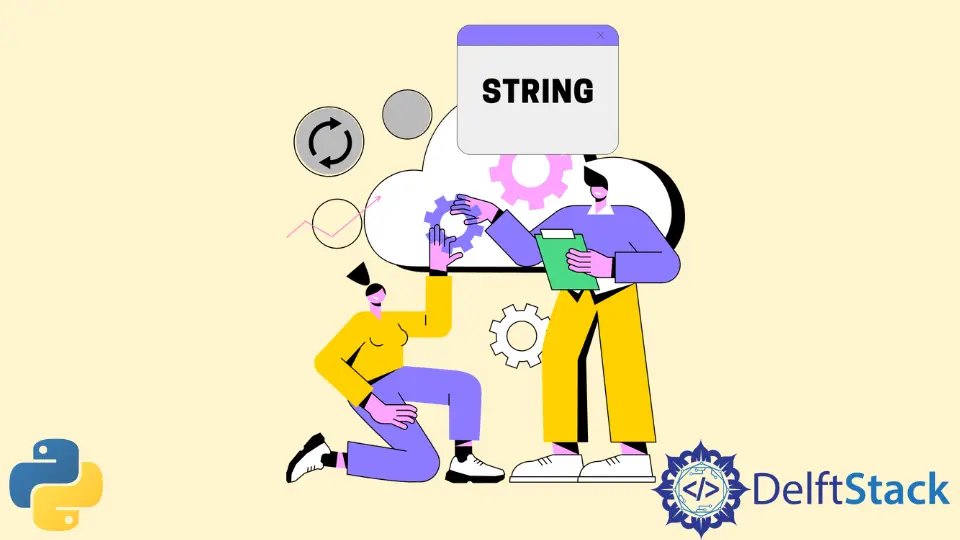
Strings are one of the most fundamental data types in Python, and understanding how to manipulate them is essential for any programmer. Iterating over a string allows you to access each character individually, making it easier to analyze or modify the string as needed.
In this tutorial, we’ll explore various methods to loop over a string in Python. Whether you’re looking to print each character, create a new string based on specific conditions, or perform more complex operations, this guide has you covered. By the end, you’ll have a solid grasp of how to effectively iterate over strings in Python, enhancing your coding skills and efficiency.
Method 1: Using a Simple For Loop
One of the most straightforward methods to loop over a string in Python is by using a for
loop. This method is not only intuitive but also highly efficient for most use cases. When you use a for
loop, you can directly access each character in the string, allowing you to perform operations on them as needed.
Here’s how you can do it:
my_string = "Hello, World!"
for char in my_string:
print(char)
Output:
H
e
l
l
o
,
W
o
r
l
d
!
In this example, we define a string called my_string
. The for
loop iterates over each character in the string, assigning it to the variable char
. The print(char)
statement then outputs each character on a new line. This method is particularly useful for tasks such as counting characters, checking for specific characters, or even transforming the string based on certain conditions.
Method 2: Using the while
Loop
Another way to loop over a string is by using a while
loop. This method provides a bit more control over the iteration process, allowing you to manipulate the index explicitly. While it may require a bit more code compared to a for
loop, it can be beneficial in scenarios where you need to apply more complex logic during iteration.
Here’s an example:
my_string = "Hello, World!"
index = 0
while index < len(my_string):
print(my_string[index])
index += 1
Output:
H
e
l
l
o
,
W
o
r
l
d
!
In this case, we initialize an index
variable to zero and use it to access each character in the string. The while
loop continues as long as the index
is less than the length of my_string
. After printing the character at the current index, we increment the index by one. This method allows for more complex operations, such as skipping characters or breaking out of the loop based on specific conditions.
Method 3: Using List Comprehension
List comprehension is a powerful feature in Python that allows you to create lists in a concise way. You can also use it to loop over a string and generate a new string or list based on certain conditions. This method is not only efficient but also makes your code cleaner and more readable.
Here’s an example of how to use list comprehension to loop over a string:
my_string = "Hello, World!"
vowels = [char for char in my_string if char in 'aeiouAEIOU']
print(vowels)
Output:
['e', 'o', 'o']
In this example, we create a list called vowels
that includes only the characters from my_string
that are vowels. The list comprehension iterates over each character in my_string
, checking if it is in the string of vowels. This method is particularly useful when you want to filter or transform data in a single line of code. It’s a great way to make your code more Pythonic and efficient.
Method 4: Using the Enumerate Function
The enumerate()
function is another excellent way to loop over a string, especially when you need to keep track of the index of each character. This function adds a counter to the iterable, allowing you to access both the index and the character simultaneously. This can be particularly useful for tasks that require both the character and its position in the string.
Here’s how to use enumerate()
:
my_string = "Hello, World!"
for index, char in enumerate(my_string):
print(f"Index {index}: {char}")
Output:
Index 0: H
Index 1: e
Index 2: l
Index 3: l
Index 4: o
Index 5: ,
Index 6:
Index 7: W
Index 8: o
Index 9: r
Index 10: l
Index 11: d
Index 12: !
In this example, enumerate(my_string)
generates pairs of index and character, which we unpack into the variables index
and char
. This allows us to print both the index and the character in a formatted string. Using enumerate()
is particularly useful when you need to know the position of a character while performing operations on the string.
Conclusion
Looping over a string in Python is a fundamental skill that can significantly enhance your programming capabilities. Whether you choose to use a simple for
loop, a while
loop, list comprehension, or the enumerate()
function, each method has its unique advantages. Understanding these methods not only improves your ability to manipulate strings but also makes your code more efficient and readable. As you continue to explore Python, mastering string manipulation will open up new avenues for creativity and problem-solving in your projects.
FAQ
-
What is the simplest way to loop over a string in Python?
The simplest way is to use afor
loop, which allows you to access each character directly. -
Can I modify the characters in a string
while
looping?
Strings in Python are immutable, meaning you cannot change them directly. However, you can create a new string based on your modifications. -
What is the advantage of using list comprehension?
List comprehension allows you to create lists in a concise and readable way, making your code cleaner and often more efficient. -
How does the enumerate function work?
Theenumerate()
function adds a counter to an iterable, allowing you to access both the index and the value during iteration. -
Are there performance differences between these methods?
Generally,for
loops and list comprehensions are more efficient thanwhile
loops due to their optimized nature in Python. However, the best method depends on your specific use case.