How to Delete Pandas DataFrame Column
-
Using the
drop()
Method - Deleting Multiple Columns
-
Using the
del
Keyword -
Using
pop()
Method - Conclusion
- FAQ
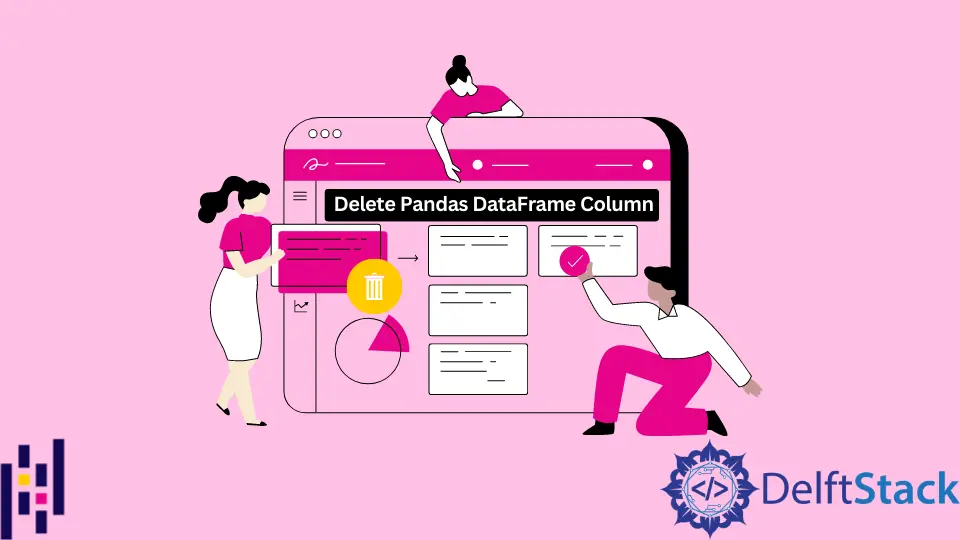
When working with data in Python, Pandas is an invaluable tool. One common task you may encounter is needing to delete columns from a DataFrame. Whether you’re cleaning up your data or simply trying to focus on specific variables, knowing how to remove columns effectively can streamline your workflow.
In this article, we’ll explore several methods to delete columns from a Pandas DataFrame, providing clear examples and explanations along the way. By the end of this guide, you’ll be equipped with the skills to manipulate your DataFrames effortlessly. Let’s dive in!
Using the drop()
Method
The drop()
method in Pandas is one of the most straightforward ways to remove a column from a DataFrame. This method allows you to specify the column you want to drop and whether you want to do this operation in place or return a new DataFrame without the specified column.
Here’s how you can use the drop()
method to delete a column:
import pandas as pd
data = {
'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35],
'City': ['New York', 'Los Angeles', 'Chicago']
}
df = pd.DataFrame(data)
df = df.drop('Age', axis=1)
print(df)
Output:
Name City
0 Alice New York
1 Bob Los Angeles
2 Charlie Chicago
In this example, we created a DataFrame with three columns: ‘Name’, ‘Age’, and ‘City’. By using the drop()
method, we specified the column ‘Age’ to be removed. The axis=1
argument indicates that we are dropping a column (as opposed to a row). The result is a new DataFrame without the ‘Age’ column, which is printed to the console. This method is particularly useful when you want to create a new DataFrame that excludes certain columns without modifying the original one.
Deleting Multiple Columns
Sometimes, you may need to delete more than one column at a time. The drop()
method can handle this as well. You can pass a list of column names to remove multiple columns simultaneously.
Here’s how to delete multiple columns:
import pandas as pd
data = {
'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35],
'City': ['New York', 'Los Angeles', 'Chicago'],
'Score': [85, 90, 95]
}
df = pd.DataFrame(data)
df = df.drop(['Age', 'Score'], axis=1)
print(df)
Output:
Name City
0 Alice New York
1 Bob Los Angeles
2 Charlie Chicago
In this example, we have added a new column called ‘Score’ to our DataFrame. By passing a list of columns—['Age', 'Score']
—to the drop()
method, we effectively removed both columns in one operation. The resulting DataFrame retains only the ‘Name’ and ‘City’ columns. This method is efficient when you need to clean up your DataFrame by removing several unnecessary columns at once.
Using the del
Keyword
Another way to remove a column from a Pandas DataFrame is by using the del
keyword. This method is useful when you want to delete a column without needing to create a new DataFrame. It directly modifies the existing DataFrame.
Here’s how you can use the del
keyword:
import pandas as pd
data = {
'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35],
'City': ['New York', 'Los Angeles', 'Chicago']
}
df = pd.DataFrame(data)
del df['City']
print(df)
Output:
Name Age
0 Alice 25
1 Bob 30
2 Charlie 35
In this example, we used the del
keyword to remove the ‘City’ column directly from the DataFrame df
. After executing this line, the DataFrame now only contains the ‘Name’ and ‘Age’ columns. This method is quick and efficient, especially when you’re sure you want to permanently remove a column from your DataFrame.
Using pop()
Method
The pop()
method is another handy way to delete a column from a DataFrame. Unlike drop()
, which returns a new DataFrame, pop()
removes the specified column from the original DataFrame and returns it. This can be especially useful if you need to use the data from the column before removing it.
Here’s how to use the pop()
method:
import pandas as pd
data = {
'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35],
'City': ['New York', 'Los Angeles', 'Chicago']
}
df = pd.DataFrame(data)
age_column = df.pop('Age')
print(df)
print(age_column)
Output:
Name City
0 Alice New York
1 Bob Los Angeles
2 Charlie Chicago
0 25
1 30
2 35
Name: Age, dtype: int64
In this example, we first removed the ‘Age’ column from the DataFrame df
using the pop()
method. The removed column is stored in the variable age_column
. After executing the code, the DataFrame now only contains the ‘Name’ and ‘City’ columns, while the ‘Age’ column is printed separately. This method is particularly useful when you want to keep the data from the removed column for further analysis or processing.
Conclusion
Deleting columns from a Pandas DataFrame is a fundamental skill that can significantly enhance your data manipulation capabilities. Whether you choose to use the drop()
, del
, or pop()
methods, each approach has its own advantages depending on your specific needs. By mastering these techniques, you’ll be able to clean and organize your data more effectively, leading to better insights and analysis. Remember to choose the method that best fits your workflow and the requirements of your project.
FAQ
-
What is the best method to delete a column in Pandas?
The best method depends on your needs.drop()
is versatile, whiledel
is quick for direct modifications, andpop()
is useful when you want to keep the data. -
Can I delete multiple columns at once in Pandas?
Yes, you can delete multiple columns by passing a list of column names to thedrop()
method. -
Does deleting a column permanently remove it from the DataFrame?
Usingdrop()
without theinplace=True
parameter creates a new DataFrame without the specified column, whiledel
andpop()
remove it permanently. -
How can I check if a column exists before deleting it?
You can check if a column exists using thein
keyword, likeif 'column_name' in df.columns:
before attempting to delete it. -
What happens if I try to delete a non-existent column?
If you try to delete a column that doesn’t exist usingdrop()
, it will raise a KeyError unless you use theerrors='ignore'
parameter. Usingdel
orpop()
will also raise an error.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - Pandas DataFrame
- How to Get Pandas DataFrame Column Headers as a List
- How to Convert Pandas Column to Datetime
- How to Convert a Float to an Integer in Pandas DataFrame
- How to Sort Pandas DataFrame by One Column's Values
- How to Get the Aggregate of Pandas Group-By and Sum