How to Set Session Timeout in PHP
-
Use the
session_unset()
andsession_destroy()
Functions to Set the Session Timeout in PHP -
Use the
unset()
Function to Set the Session Timeout in PHP -
Use the
session_regenerate_id()
Function to Change the Current Session ID in PHP
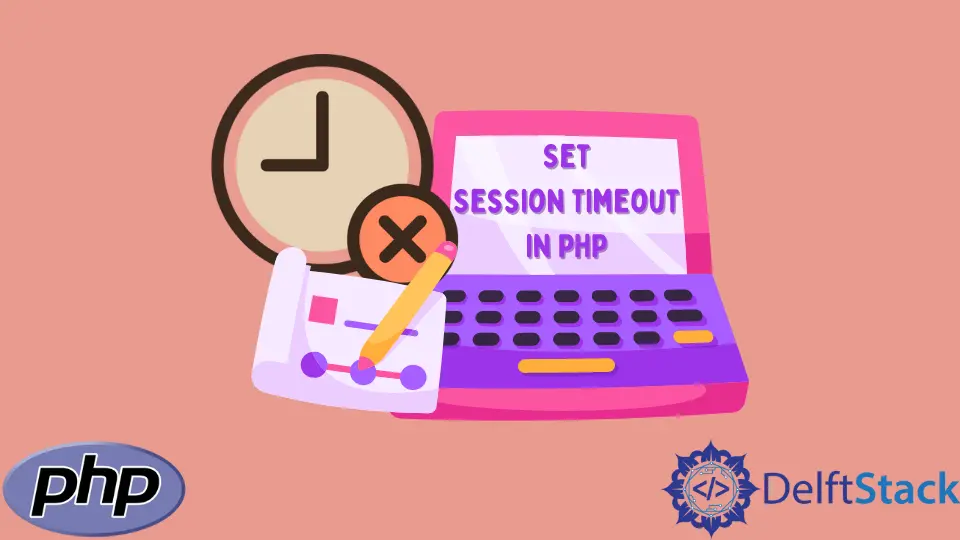
This article will introduce a method to destroy the session in PHP using the session_unset()
and the session_destroy()
functions. We will destroy the session after 30 minutes. It checks the time interval from the last activity to the current time to destroy the session.
We will also demonstrate another way to set the session timeout in PHP using the unset()
function. The unset()
function takes the session variable as the parameter. This method uses an array to store the session variable.
We will introduce another method to invalidate the current session id using the session_regenerate_id()
function. This function generates a new session id for the current session destroying the previous session-id.
Use the session_unset()
and session_destroy()
Functions to Set the Session Timeout in PHP
We can use the session_unset()
function to unset the $_SESSION
variable at the run-time and use the session_destroy()
function to destroy the session from the storage. The time()
function returns the current time. We can use the $_SESSION
variable to store the timestamp of the last activity. Thus, we can check the time by calculating the differences from the last activity to the current time. In this way, we check the time interval of a session.
For example, start the session in the server with the session_start()
function. Check if the session has been created with the isset()
function. Use the &&
operator to check the other condition inside the issset()
function. Subtract the session variable start
from the time()
function and check the result if it is greater than 1800. Use the session_unset()
and session_destroy()
inside the if
condition. Then display the message saying the session has been destroyed. Outside the if
condition, create a session variable $_SESSION['start']
and assign the time()
function to it to update the session.
The if
condition fails as the session variable has not been yet created in the example below. The session will be created when the condition fails. The session expires after 30 minutes if the user does not send the request to the server. The latter part of the isset()
function checks the total active time of the session. 1800
denotes 1800 seconds which is equivalent to 30 minutes. If the total active time exceeds 30 minutes, the if condition will be true. Then, it destroys the session. The output of the code corresponds to the session being destroyed after more than 30 minutes of the active time. Check the PHP Manual to learn more about the session_unset()
function.
Example Code:
# php 7.*
<?php
session_start();
if (isset($_SESSION['start']) && (time() - $_SESSION['start'] > 1800)) {
session_unset();
session_destroy();
echo "session destroyed";
}
$_SESSION['start'] = time();
?>
Output:
session destroyed
Use the unset()
Function to Set the Session Timeout in PHP
We can use the unset()
function to destroy the session of a particular session variable in PHP. The function takes the $_SESSION
variable as the parameter. This method stores the session in an array. We can use the associative array to store the session name and the session start time. We can use the time()
function to get the current time. We can check the session time interval following the method mentioned above, subtracting the active session time from the current time.
For example, use the session_start()
function to start the session. Write a session variable $_SESSION['start']
and assign an associative array to it. Write the keys 0
and registered
and write the values active
and time()
for the respective keys. Check whether the time interval is greater than 30 minutes. If the condition is true, write the unset()
function with the $_SESSION['start']
as the parameter. Then, display the message session destroyed
. Outside the if
condition block, update the session variable $_SESSION['start']
with the time()
function.
In the example below, the array contains the session variable. The value of the session variable is active
. The time()
function creates the current timestamp in the array, and the key registered
holds the value. Please, check the PHP Manual to learn more about the $_SESSION
superglobal variable.
Example Code:
#php 7.x
<?php
session_start();
$_SESSION['start'] = array(0=> 'active', 'registered' => time())
if ((time() - $_SESSION['start']['registered']) > (60 * 30)) {
unset($_SESSION['start']);
echo "session destroyed";
}
$_SESSION['start'] = time();
?>
Output:
session destroyed
Use the session_regenerate_id()
Function to Change the Current Session ID in PHP
We can use the session_regenerate_id()
function to change the session id of the current session. In this way, we can prevent our script from session fixation. The boolean value true
in the function enables the generation of a new session-id. In this method, we can print the session id using the session_id()
function before and after the regeneration of the session id. But the session does not expire with the change of the session-id. It only asks the user to log in again. Therefore, it prevents the session fixation.
For example, start the session with the session_id()
function. Display the current session id using the session_id()
function. Check whether the session has been created and create a session $_SESSION['start']
and assign the time()
function. Find the time interval of the session being active as we did in the above method. If the condition is true use the session_regenerate_id(true)
function. Update the session variable. At the end of the script, print the new session id with the session_id()
function.
Example Code:
#php 7.x
<?php
session_start();
print("Old: ".session_id());
echo "<br>";
if (!isset($_SESSION['start'])) {
$_SESSION['start'] = time();
} else if (time() - $_SESSION['start'] > 1800) {
session_regenerate_id(true);
$_SESSION['start'] = time();
}
print("Now: ".session_id());
?>
Output:
Old: t38kssej37un38mtsoa6lovh05
Now: mak7i3in708c6rl2udd8gbfo3r
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn