Session ID in PHP
-
Check Session ID With
session_id()
Function -
Set and Get a User Session ID From
$_session[]
Variable in PHP -
Get Session ID With
var_dump()
in PHP - Session ID and Uniqueness
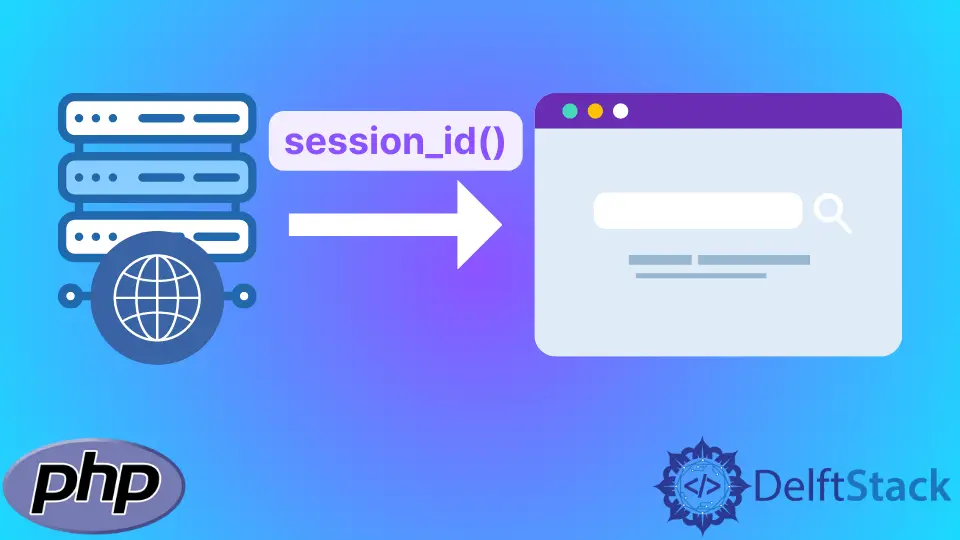
One of the main use of a session id is the identification of users or events on a web application. This article will explain how you can work with PHP session id with the help of built-in PHP functions like session_start()
and session_id()
.
Check Session ID With session_id()
Function
Before you can check for a session ID, you need to start a PHP session with session_start()
. Afterward, you can call on the session_id()
function. This function will return the current session id.
<?php
session_start();
echo session_id();
?>
Output:
3rd5hnbqgjhi3fq4b2edsajq81
Set and Get a User Session ID From $_session[]
Variable in PHP
PHP $_SESSION
variable is an associative array that contains the current script’s PHP session variables. You can add a specific key-value pair to the array, delete it from the array, or empty the entire $_SESSION
variable.
You can store the details of authenticated users in the $_SESSION
variable. Before that, you need to start a PHP session. Once the session is active, you can register a session for an authenticated user. Afterward, you can use the session id to track the user across the system. When the user logs out, you can destroy the session.
In the next code block, you’ll find details on how to set and get a user session id.
<?php
// Start the session
session_start();
// get the session id
$session_id = session_id();
// The username of the user. On most occasions,
// you'll get this from a MySQL query
$username = "DelftStack";
// Register a session for the user
$_SESSION['username'] = $username;
// Display the session id and the registered
// register
echo "The session id is: " . $session_id;
echo "<br /> The session has been registered to: " . $username;
?>
Output:
The session id is: d7ao75228pobka332fqeho10l3
The session has been registered to: DelftStack
Remember, your session id will be different than the one shown above.
You can destroy the session with the following code:
<?php
if (isset($_SESSION['username'])) {
// Reset the session
unset($_SESSION);
// Destroy the session
session_destroy();
if (empty($_SESSION)) {
echo "Session destroyed...";
}
}
?>
Output:
Session destroyed...
Get Session ID With var_dump()
in PHP
The var_dump()
function will dump the details about a variable, including the $_SESSION[]
variable. Start the session and store the session id in a variable to get started with this process. Once the session id is in a variable, you can dump it with var_dump
.
The next code block shows how to get session id with var_dump()
.
<?php
// Start the session
session_start();
// get the session id
$session_id = session_id();
// store the session id in the
// session variable
$_SESSION['id'] = $session_id;
// Dump the username
var_dump($_SESSION['id']);
?>
Output:
string(26) "7qfm3qvjj1vku6h78p73qh9jmn"
Session ID and Uniqueness
It’s recommended not to start a new session when you need a unique identifier for a user. That’s when a function like uniqid()
comes into play. But, if you have an active session, you can use session_id()
. Still, do not rely on it for uniqueness.
Here is why: A web browser with multiple tabs will use the same process. As a result, they will use the same session identifier. It means different user connections will have the same id.
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn