How to Escape Quotation Marks in PHP
-
Use the Backslash
\
Before the Quotation to Escape the Quotation Marks -
Use the Heredoc Syntax
<<<
to Escape the Quotation Marks From a String in PHP - Use the Single Quotes or the Double Quotes Alternately to Escape the Quotation Marks in PHP
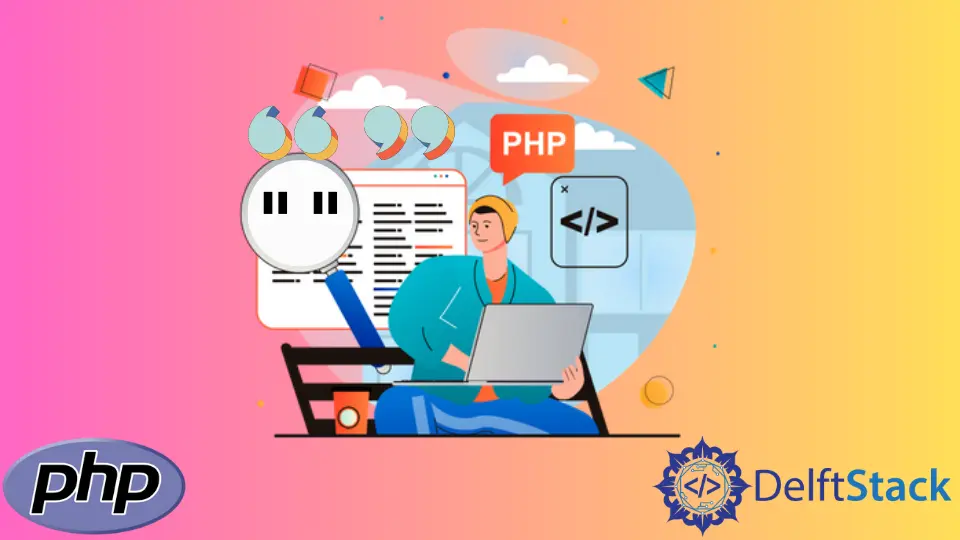
This article will introduce methods to escape the quotation mark in a string in PHP.
Use the Backslash \
Before the Quotation to Escape the Quotation Marks
We can use the backslash \
to escape special characters in PHP. When we try to incorporate the quotation marks in the string in PHP, the script will throw a parse error. So, we need to escape the quotation mark so that the script executes without any error. We can use this technique when we need to print a string that contains a direct speech. Let’s consider a direct speech sentence, She asked me, " Are you going out tonight ?".
. When we print this string, a parse error will be thrown. It is because the whole string will be wrapped inside the " "
double-quotes. When the double quotes before the Are
are encountered during the flow of the code, the compiler does not expect string after it. It rather expects a semicolon or concatenation operator. Thus, the compiler throws a parse error.
For example, write backslashes right before the two double quotes that enclose the text Are you going out tonight ?
. Assign the string in a variable $text
and print the variable using the echo
command. Thus, it will display a complete direct speech sentence with double quotes.
Example Code:
#php 7.x
<?php
$text = "She asked me, \" Are you going out tonight ?\"";
echo $text
?>
Output:
She asked me, " Are you going out tonight ?".
Use the Heredoc Syntax <<<
to Escape the Quotation Marks From a String in PHP
We can use the heredoc syntax <<<
to escape quotation marks from a string in PHP. An identifier follows right after the syntax and the string in the new line. The string or the text inside the identifier is called heredoc text. We should use the same identifier after the string in the new line in the first column to denote the end of the heredoc. We should use the semicolon after the closing identifier to indicate the end. The heredoc text is regarded to be inside double quotes without the use of the double-quotes. The double quotes and single quotes in the heredoc text automatically get escaped. We can still use variables in the heredocs and apply quotation marks to them.
For example, create two variables, $start
and $end
, to store the strings hello
and goodbye
. Create another variable $heredoc
and write the heredoc syntax <<<
to it. Write an identifier term
right after the syntax. In the next line, write the heredoc text. Wrap the $start
variable with double quotes at the start of the text. Write the text We can use the "heredocs" to incorporate the 'single quotes' and the "double quotes" in a string.
. Note to wrap the words heredocs
and double qoutes
with double quotes and the word single quote
with a single quote as written above. In the end, wrap the $end
variable with double quotes. In the next line, write the term
identifier with a semicolon after ending the heredoc. Then, print the $heredoc
variable.
The example above outputs the text with a single quote and double quotes. The use of heredoc syntax <<<
makes it easy to escape the quotation marks from a string in PHP. Please check the PHP Manual to learn more about the heredoc.
Code Example:
#php 7.x
<?php
$start = "hello";
$end = "goodbye";
$heredoc = <<<term
"$start", We can use the "heredocs" to incorporate the 'single quote' and the "double quotes" in a string. "$end".
term;
echo $heredoc;
?>
Output:
"hello", We can use the "heredocs" to incorporate the 'single quote' and the "double quotes" in a string. "goodbye".
Use the Single Quotes or the Double Quotes Alternately to Escape the Quotation Marks in PHP
We can use the double quotes to escape the single quote and use the single quote to escape the double quotes. Thus, we can escape the quotation marks in a string in PHP. There is a slight difference while using the double quotes and the single quotes in the string. We can perform the string interpolation while using the double quotes, but the single quote doesn’t allow this. Interpolation is a method of referencing the variables in a string to evaluate their values.
For example, write a string Over and over again
wrapped inside a single quote. Wrap the word over
with double quotes. Use the echo
command to display the string. In the following line, wrap the same string with double quotes and use the single quote to wrap the same word over
. Print the string.
The double quotes are escaped in the first string in the example below, and the single quote is escaped in the second string. It is possible due to the use of alternate quotes to wrap the whole string.
Example Code:
# php 7.x
<?php
echo 'Over and "over" again'."<br>";
echo "Over and 'over' again";
?>
Output:
Over and "over" again
Over and 'over' again
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn