NumPy Magnitude in Python
-
Calculate NumPy Magnitude With the
numpy.linalg.norm()
Function in Python -
Calculate NumPy Magnitude With the
numpy.dot()
Function in Python -
Calculate NumPy Magnitude With the
numpy.einsum()
Function in Python
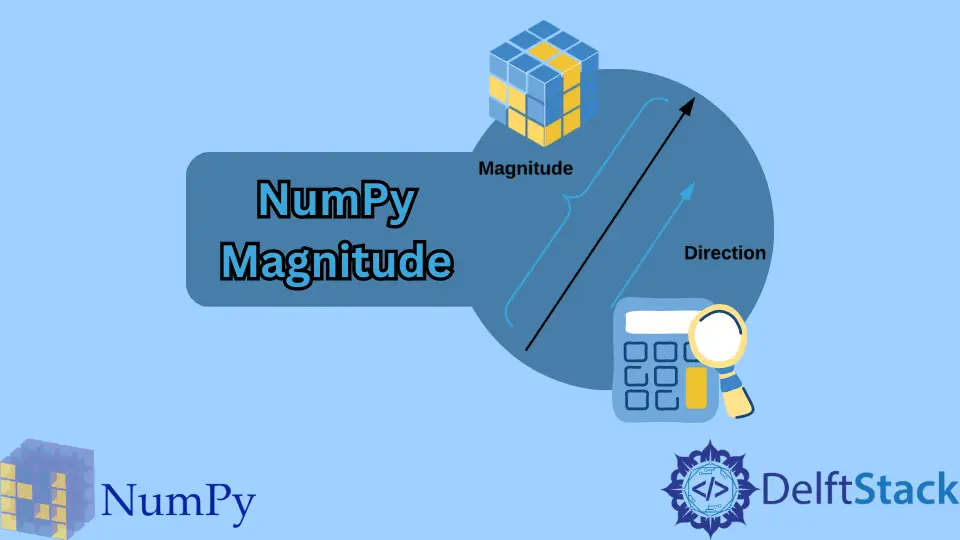
This tutorial will introduce the methods to calculate the magnitude of a vector in NumPy.
Calculate NumPy Magnitude With the numpy.linalg.norm()
Function in Python
Suppose we have a vector in the form of a 1-dimensional NumPy array, and we want to calculate its magnitude. We can use the numpy.linalg.norm()
function for this purpose. The numpy.linalg
sub-module in the NumPy
library contains functions related to linear algebra. The norm()
function inside the numpy.linalg
sub-module takes the vector as an input parameter and returns its magnitude.
import numpy as np
v = np.array([1, 1, 3, 4])
magnitude = np.linalg.norm(v)
print(magnitude)
Output:
5.196152422706632
In the above code, we calculated the magnitude of the vector v
with the np.linalg.norm(v)
function. We first created our vector v
in the form of a 1D NumPy array with the n.array()
function. We then calculated its magnitude with the np.linalg.norm(v)
function and stored the output inside the magnitude
variable.
Calculate NumPy Magnitude With the numpy.dot()
Function in Python
We can also use the numpy.dot()
function along with the numpy.sqrt()
function to calculate the magnitude of a vector in NumPy. The numpy.dot()
function calculates the dot-product between two different vectors, and the numpy.sqrt()
function is used to calculate the square root of a particular number. We can calculate the dot-product of the vector with itself and then take the square root of the result to determine the magnitude of the vector.
import numpy as np
v = np.array([1, 1, 3, 4])
magnitude = np.sqrt(v.dot(v))
print(magnitude)
Output:
5.196152422706632
We first created our vector v
in the form of a 1D NumPy array with the n.array()
function. We then calculated the dot-product of the vector v
with itself by v.dot(v)
function and took square root of the resultant value with the np.sqrt(v.dot(v))
function. The result of the square root is the magnitude of the vector v
.
Calculate NumPy Magnitude With the numpy.einsum()
Function in Python
The numpy.einsum()
function performs the Einstein summation convention on its operands. This method is similar to taking the dot-product of a vector with itself. We can use the numpy.einsum()
function along with the numpy.sqrt()
function to achieve the same goal as the previous examples. We can determine the magnitude of a vector by performing Einstein summation convention and then taking the square root of the resultant value.
import numpy as np
v = np.array([1, 1, 3, 4])
magnitude = np.sqrt(np.einsum("i,i", v, v))
print(magnitude)
Output:
5.196152422706632
We first created our vector v
in the form of a 1D NumPy array with the n.array()
function. We then performed the Einstein summation convention with the np.einsum('i,i',v,v)
function. We then calculated the square root of the resultant value and stored the result in the magnitude
variable.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn