Python NumPy numpy.linalg.norm() Function
-
Syntax of
numpy.linalg.norm()
-
Example Codes:
numpy.linalg.norm()
-
Example Codes:
numpy.linalg.norm()
to Find the Norm of a Two-Dimensional Array -
Example Codes:
numpy.linalg.norm()
to Find the Vector Norm and Matrix Norm Usingaxis
Parameter -
Example Codes:
numpy.linalg.norm()
to Useord
Parameter
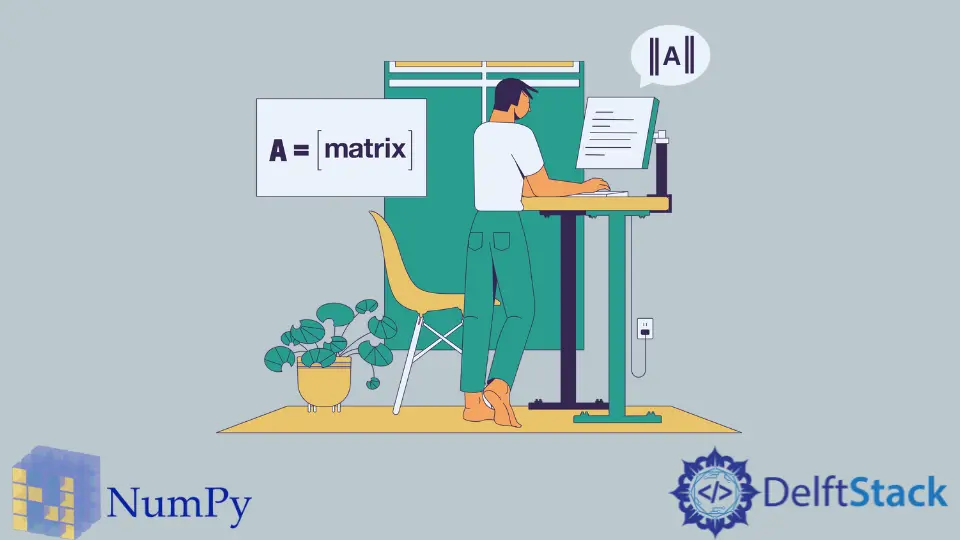
Python NumPy numpy.linalg.norm()
function finds the value of the matrix norm or the vector norm. The parameter ord
decides whether the function will find the matrix norm or the vector norm. It has several defined values.
Syntax of numpy.linalg.norm()
numpy.linalg.norm(x, ord=None, axis=None, keepdims=False)
Parameters
x |
It is an array-like structure. It is the input array used for finding the value of the norm. The default value for the axis parameter is None so, the array should be one-dimensional or two-dimensional provided ord is None . |
ord |
The returned value of the function depends on this parameter. It defines the order of the norm. It has several values, check here. |
axis |
It is an integer, None or 2 tuple of integers. If it is an integer then it represents the axis along which the function will find the vector norm. Its default value is None which means that the function will find either matrix norm or vector norm. If it is a 2 tuple integer value then the function will return the value of matrix norm. |
keepdims |
It is a boolean parameter. Its default value is False . If its value is True then it shows the dimensions of the normed axis with the size equal to one. |
Return
It returns the norm of the matrix or a vector in the form of a float
value or an N-dimensional array.
Example Codes: numpy.linalg.norm()
We will use this function to find the norm of a one-dimensional array.
from numpy import linalg as la
import numpy as np
x = np.array(
[89, 34, 56, 87, 90, 23, 45, 12, 65, 78, 9, 34, 12, 11, 2, 65, 78, 82, 28, 78]
)
norm = la.norm(x)
print("The value of norm is:")
print(norm)
Output:
The value of norm is:
257.4800963181426
It has returned a float
value which is the value of the norm.
Example Codes: numpy.linalg.norm()
to Find the Norm of a Two-Dimensional Array
We will pass a two-dimensional array now.
from numpy import linalg as la
import numpy as np
x = np.array([[11, 12, 5], [15, 6, 10], [10, 8, 12], [12, 15, 8], [34, 78, 90]])
norm = la.norm(x)
print("The value of norm is:")
print(norm)
Output:
The value of norm is:
129.35223229616102
If we set the ord
parameter to any other value than None
and pass an array that is neither one-dimensional nor two-dimensional, the function will generate a ValueError
as the axis
parameter is None
.
from numpy import linalg as la
import numpy as np
x = np.array([[[4, 2], [6, 4]], [[5, 8], [7, 3]]])
norm = la.norm(x, "nuc")
print("The value of norm is:")
print(norm)
Output:
Traceback (most recent call last):
File "C:\Test\test.py", line 6, in <module>
norm = la.norm(x,'nuc')
File "<__array_function__ internals>", line 5, in norm
File "D:\WinPython\WPy64-3820\python-3.8.2.amd64\lib\site-packages\numpy\linalg\linalg.py", line 2557, in norm
raise ValueError("Improper number of dimensions to norm.")
ValueError: Improper number of dimensions to norm.
Example Codes: numpy.linalg.norm()
to Find the Vector Norm and Matrix Norm Using axis
Parameter
We will find the vector norm first.
from numpy import linalg as la
import numpy as np
x = np.array([[11, 12, 5], [15, 6, 10], [10, 8, 12], [12, 15, 8], [34, 78, 90]])
norm = la.norm(x, axis=0)
print("The vector norm is:")
print(norm)
Output:
The vector norm is:
[41.78516483 80.95060222 91.83136719]
Note that the function has returned an N-dimensional array as the computed vector norm.
Now, we will compute the matrix norm. We will pass the axis
parameter as the 2- tuple
of the integer value.
from numpy import linalg as la
import numpy as np
x = np.array([[11, 12, 5], [15, 6, 10], [10, 8, 12], [12, 15, 8], [34, 78, 90]])
norm = la.norm(x, axis=(0, 1))
print("The value of matrix norm is:")
print(norm)
Output:
The value of matrix norm is:
129.35223229616102
Example Codes: numpy.linalg.norm()
to Use ord
Parameter
The parameter ord
has several values.
from numpy import linalg as la
import numpy as np
x = np.array([[11, 12, 5], [15, 6, 10], [10, 8, 12], [12, 15, 8], [34, 78, 90]])
norm = la.norm(x, "fro")
print("The value of matrix norm is:")
print(norm)
Output:
The value of matrix norm is:
129.35223229616102
The function has generated the value of the Frobenius
matrix norm.
from numpy import linalg as la
import numpy as np
x = np.array([[11, 12, 5], [15, 6, 10], [10, 8, 12], [12, 15, 8], [34, 78, 90]])
norm = la.norm(x, "nuuc")
print("The value of matrix norm is:")
print(norm)
Output:
The value of matrix norm is:
152.28781231351272
The function has generated the matrix norm. It is the sum of the singular values.