How to Get Combinations of Two Arrays in NumPy
-
Get NumPy Array Combinations With the
itertools.product()
Function in Python -
Get NumPy Array Combinations With the
numpy.meshgrid()
Function in Python -
Get NumPy Array Combinations With the
for-in
Method in Python
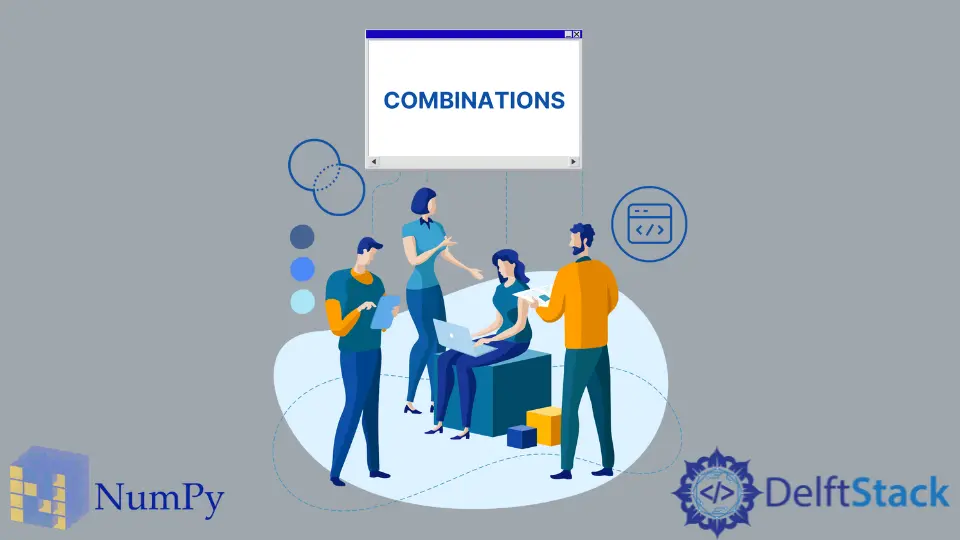
This article will introduce how to find the cartesian product of two NumPy arrays in Python.
Get NumPy Array Combinations With the itertools.product()
Function in Python
The itertools
package provides many functions related to combination and permutation. We can use the itertools.product()
function cartesian product of two iterables. The itertools.product()
function takes the iterables as input parameters and returns the cartesian product of the iterables.
import itertools as it
import numpy as np
array = np.array([1, 2, 3])
combinations = it.product(array, array)
for combination in combinations:
print(combination)
Output:
(1, 1)
(1, 2)
(1, 3)
(2, 1)
(2, 2)
(2, 3)
(3, 1)
(3, 2)
(3, 3)
In the above code, we calculated the cartesian cross-product of the array
with itself by using the product()
function inside the itertools
package and stored the result in combinations
.
Get NumPy Array Combinations With the numpy.meshgrid()
Function in Python
We can also use the meshgrid()
function inside the NumPy package to calculate the cartesian product of two NumPy arrays. The numpy.meshgrid()
function takes the arrays as input arguments and returns the cross-product of the two arrays.
import numpy as np
array = np.array([1, 2, 3])
combinations = np.array(np.meshgrid(array, array)).T.reshape(-1, 2)
print(combinations)
Output:
[[1 1]
[1 2]
[1 3]
[2 1]
[2 2]
[2 3]
[3 1]
[3 2]
[3 3]]
In the above code, we calculated the cartesian cross-product of the array
with itself by using the meshgrid()
function in NumPy. We then converted the outcome of this operation into an array with the np.array()
function and reshaped it with the numpy.reshape()
function. We then stored the new reshaped result inside the combinations
array.
Get NumPy Array Combinations With the for-in
Method in Python
Another more straightforward method of achieving the same goal as the previous two examples is to use the for-in
iterator. The for-in
iterator is used to iterator through each element inside an iterable in Python. This method can be used without importing any new package or library.
import numpy as np
array = np.array([1, 2, 3])
array2 = np.array([1, 2, 3])
combinations = np.array([(i, j) for i in array for j in array2])
print(combinations)
Output:
[[1 1]
[1 2]
[1 3]
[2 1]
[2 2]
[2 3]
[3 1]
[3 2]
[3 3]]
We calculated the cartesian cross-product of both arrays using a nested for-in
iterator in the above code. We saved the result inside the NumPy array combinations
with the np.array()
function.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn