NumPy에서 두 배열의 조합 가져 오기
-
Python에서
itertools.product()
함수를 사용하여 NumPy 배열 조합 가져 오기 -
Python에서
numpy.meshgrid()
함수를 사용하여 NumPy 배열 조합 가져 오기 -
Python에서
for-in
메소드를 사용하여 NumPy 배열 조합 가져 오기
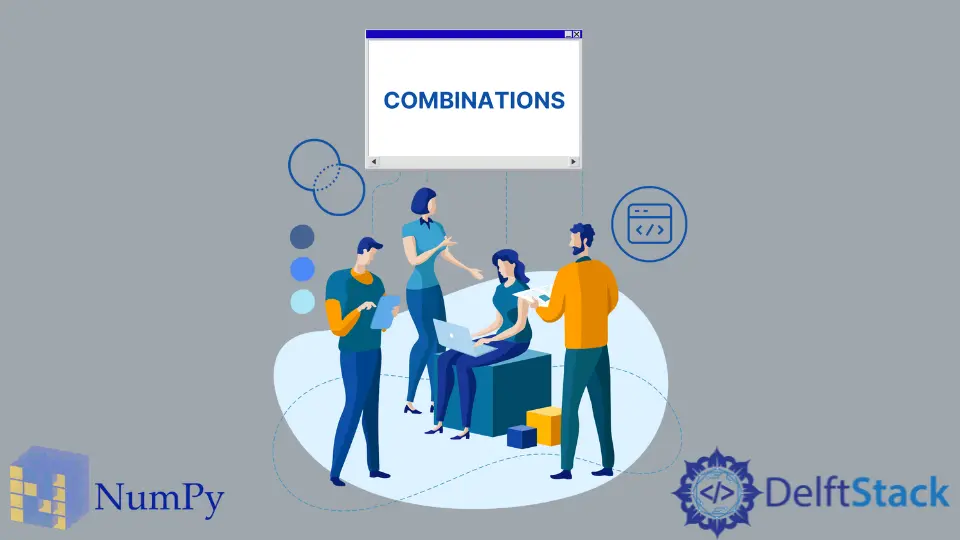
이 기사에서는 Python에서 두 개의 NumPy 배열의 데카르트 곱을 찾는 방법을 소개합니다.
Python에서itertools.product()
함수를 사용하여 NumPy 배열 조합 가져 오기
itertools
패키지는 조합 및 순열과 관련된 많은 기능을 제공합니다. 두 개의 iterables의 itertools.product()
function 데카르트 곱을 사용할 수 있습니다. itertools.product()
함수는 이터 러블을 입력 매개 변수로 취하고 이터 러블의 데카르트 곱을 반환합니다.
import itertools as it
import numpy as np
array = np.array([1, 2, 3])
combinations = it.product(array, array)
for combination in combinations:
print(combination)
출력:
(1, 1)
(1, 2)
(1, 3)
(2, 1)
(2, 2)
(2, 3)
(3, 1)
(3, 2)
(3, 3)
위의 코드에서itertools
패키지 내의product()
함수를 사용하여array
의 데카르트 외적을 계산하고 결과를combinations
에 저장했습니다.
Python에서numpy.meshgrid()
함수를 사용하여 NumPy 배열 조합 가져 오기
NumPy 패키지 내의 meshgrid()
함수를 사용하여 두 NumPy 배열의 데카르트 곱을 계산할 수도 있습니다. numpy.meshgrid()
함수는 배열을 입력 인수로 취하고 두 배열의 외적을 반환합니다.
import numpy as np
array = np.array([1, 2, 3])
combinations = np.array(np.meshgrid(array, array)).T.reshape(-1, 2)
print(combinations)
출력:
[[1 1]
[1 2]
[1 3]
[2 1]
[2 2]
[2 3]
[3 1]
[3 2]
[3 3]]
위의 코드에서 우리는 NumPy의meshgrid()
함수를 사용하여array
의 데카르트 외적을 계산했습니다. 그런 다음이 연산의 결과를np.array()
함수를 사용하여 배열로 변환하고numpy.reshape()
함수를 사용하여 모양을 변경했습니다. 그런 다음combinations
배열 안에 새로운 재구성 된 결과를 저장했습니다.
Python에서for-in
메소드를 사용하여 NumPy 배열 조합 가져 오기
앞의 두 예와 동일한 목표를 달성하는 또 다른보다 간단한 방법은for-in
반복자를 사용하는 것입니다. for-in
반복자는 Python에서 반복 가능한 내부의 각 요소를 반복하는 데 사용됩니다. 이 방법은 새 패키지 또는 라이브러리를 가져 오지 않고 사용할 수 있습니다.
import numpy as np
array = np.array([1, 2, 3])
array2 = np.array([1, 2, 3])
combinations = np.array([(i, j) for i in array for j in array2])
print(combinations)
출력:
[[1 1]
[1 2]
[1 3]
[2 1]
[2 2]
[2 3]
[3 1]
[3 2]
[3 3]]
위 코드에서 중첩 된for-in
반복자를 사용하여 두 배열의 데카르트 외적을 계산했습니다. 결과를np.array()
함수를 사용하여 NumPy 배열combinations
에 저장했습니다.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn