How to Prevent Form Submission JavaScript
- Method 1: Using Event Listeners
- Method 2: Using the onsubmit Attribute
- Method 3: Using jQuery for Form Validation
- Conclusion
- FAQ
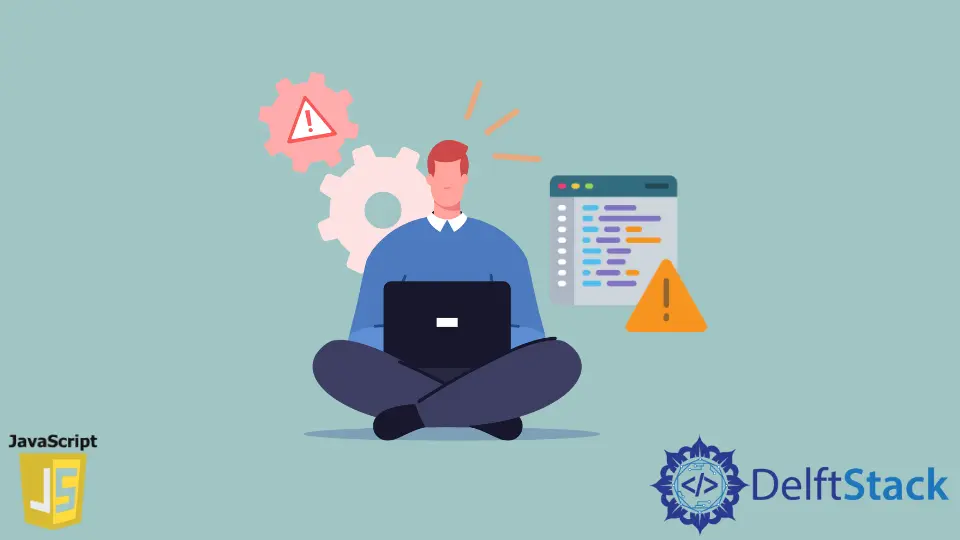
When it comes to web development, handling form submissions is a crucial aspect. Sometimes, you may want to prevent a form from being submitted until certain conditions are met. This can be particularly important for validating user input or ensuring that a user has completed all necessary fields.
In this tutorial, we will explore how to prevent form submission using JavaScript, making your forms more interactive and user-friendly. Whether you are new to web development or looking to refine your skills, understanding how to control form submission can significantly enhance the user experience on your website. Let’s dive into the various methods of preventing form submission in JavaScript.
Method 1: Using Event Listeners
One of the most common ways to prevent form submission in JavaScript is by using event listeners. By attaching an event listener to the form’s submit event, you can control whether the form should be submitted or not. This method is straightforward and effective for basic validation.
Here’s how you can implement it:
document.getElementById('myForm').addEventListener('submit', function(event) {
event.preventDefault();
// Additional validation logic can go here
console.log('Form submission prevented');
});
Output:
Form submission prevented
In this example, we’re targeting a form with the ID myForm
. The addEventListener
method listens for the submit
event. When the form is submitted, the event handler function is triggered. The event.preventDefault()
method stops the form from being submitted. You can add further validation logic inside this function to check if all required fields are filled out or if the input values meet specific criteria. If the validation fails, the form submission will be halted, allowing you to provide feedback to the user.
Method 2: Using the onsubmit Attribute
Another effective way to prevent form submission is by using the onsubmit
attribute directly in the HTML form tag. This method is less common in modern web development but still serves as a quick and simple way to manage form submission prevention.
Here’s an example:
<form id="myForm" onsubmit="return validateForm()">
<input type="text" id="name" required>
<input type="submit" value="Submit">
</form>
<script>
function validateForm() {
const name = document.getElementById('name').value;
if (name === "") {
alert('Name is required!');
return false; // Prevents form submission
}
return true; // Allows form submission
}
</script>
Output:
Name is required!
In this example, we have a form with an onsubmit
attribute that calls the validateForm()
function. Inside this function, we check if the name
input field is empty. If it is, an alert is shown, and the function returns false
, which prevents the form from being submitted. If the input is valid, the function returns true
, allowing the form to proceed with submission. This method is particularly useful for quick validations without needing to write extensive JavaScript code.
Method 3: Using jQuery for Form Validation
If you are using jQuery in your project, it can simplify the process of preventing form submission. jQuery provides a clean and concise way to handle events, making your code more readable and easier to manage.
Here’s how to prevent form submission using jQuery:
<form id="myForm">
<input type="text" id="email" required>
<input type="submit" value="Submit">
</form>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script>
$(document).ready(function() {
$('#myForm').on('submit', function(event) {
if ($('#email').val() === '') {
alert('Email is required!');
event.preventDefault();
}
});
});
</script>
Output:
Email is required!
In this example, we use jQuery to attach an event listener to the form’s submit event. When the form is submitted, we check if the email input field is empty. If it is, we display an alert and call event.preventDefault()
to stop the form submission. This method is advantageous because jQuery handles cross-browser compatibility issues, allowing you to focus on writing your validation logic without worrying about inconsistencies across different browsers.
Conclusion
Preventing form submission in JavaScript is an essential skill for web developers. By using event listeners, the onsubmit
attribute, or jQuery, you can effectively manage user input and enhance the overall user experience. Whether you choose to implement simple validation or more complex logic, mastering these techniques will help you create more robust and user-friendly web forms. Remember that the goal is to ensure that users provide the necessary information before allowing them to submit the form. Happy coding!
FAQ
-
How can I prevent form submission without JavaScript?
You cannot fully prevent form submission without JavaScript, but you can use HTML5 validation attributes likerequired
,pattern
, andminlength
to enforce some rules. -
Is it better to use jQuery or plain JavaScript for form validation?
It depends on your project requirements. jQuery simplifies DOM manipulation and event handling, making it easier for beginners. However, for modern web development, plain JavaScript is often preferred for its performance and reduced dependency. -
Can I prevent form submission on mobile devices?
Yes, the methods described in this article work on mobile devices as well. Just ensure that the JavaScript is correctly linked and that the event listeners are set up properly. -
What should I do if the form validation fails?
If validation fails, you should provide clear feedback to the user, indicating what needs to be corrected. This can be done through alerts, inline messages, or highlighting the fields that require attention. -
Are there any libraries for form validation?
Yes, there are several libraries available, such as Parsley.js, Formik, and Yup, which can help streamline the form validation process in your web applications.