How to Export Array to CSV in JavaScript
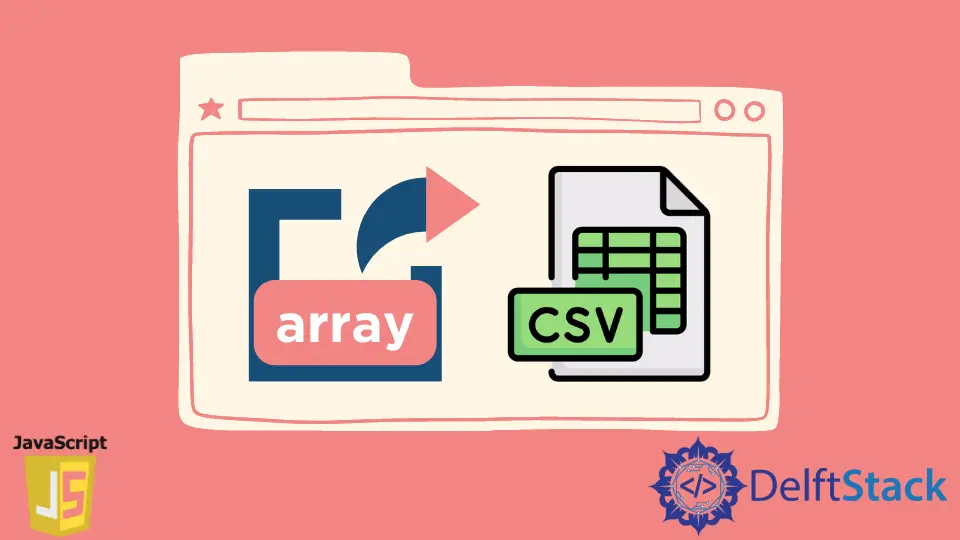
This tutorial will demonstrate how to export JavaScript array information to a CSV file on the client side.
To convert the array information into a CSV file, we need to correctly parse the JavaScript data into CSV format. We need to store it as an object that can handle the encoding and CSV data format.
In our example, we use an array of arrays to store the data.
const data =
[['rahul', 'delhi', 'accounts dept'], ['rajeev', 'UP', 'sales dept']];
let csvContent = 'data:text/csv;charset=utf-8,';
data.forEach(function(rowArray) {
let row = rowArray.join(',');
csvContent += row + '\r\n';
});
We store content from the data
array to the csvContent
object. We create rows of each array and store it in a separate object.
Alternatively, we can use the following code as it a shorter way of achieving the same result using arrow functions.
const data =
[['rahul', 'delhi', 'accounts dept'], ['rajeev', 'UP', 'sales dept']];
let csvContent =
'data:text/csv;charset=utf-8,' + data.map(e => e.join(',')).join('\n');
The map()
function calls a function on each element in the array and creates a new array. The join()
method will join the components from the existing array to a string with the help of a separator. In our case, we use a comma as a separator.
To download and encode the above-created CSV object, we use the following code.
var encodedUri = encodeURI(csvContent);
window.open(encodedUri);
Now we will export this csvContent
object to an external file. The EncodeURI
is used to encode the URI. This function encodes special characters except (, / ? : @ & = + $)
and returns a string value that represents the encoded URI.
To give the CSV file a particular name we need to create a hidden DOM node and have to set the download function.
This is done below.
var encodedUri = encodeURI(csvContent);
var link = document.createElement('a');
link.setAttribute('href', encodedUri);
link.setAttribute('download', 'my_data.csv');
document.body.appendChild(link);
link.click();
The setAttribute()
method adds a specific attribute value to the given element. In the above code link.click()
is used to download to directly download the file name provided in the link.setAttribute()
function.
If we want to have our data inserted within double quotes, we can use the JSON.stringify()
function while creating the CSV data object.
See the code below.
const data =
[['rahul', 'delhi', 'accounts dept'], ['rajeev', 'UP', 'sales dept']];
var csv = data.map(function(d) {
return JSON.stringify(d);
})
.join('\n')
.replace(/(^\[)|(\]$)/mg, '');
console.log(csv);
Output:
"rahul" "delhi" "accounts"
"rajev" "UP" "sales dept"