How to Convert Bytes to Gigabytes Using JavaScript
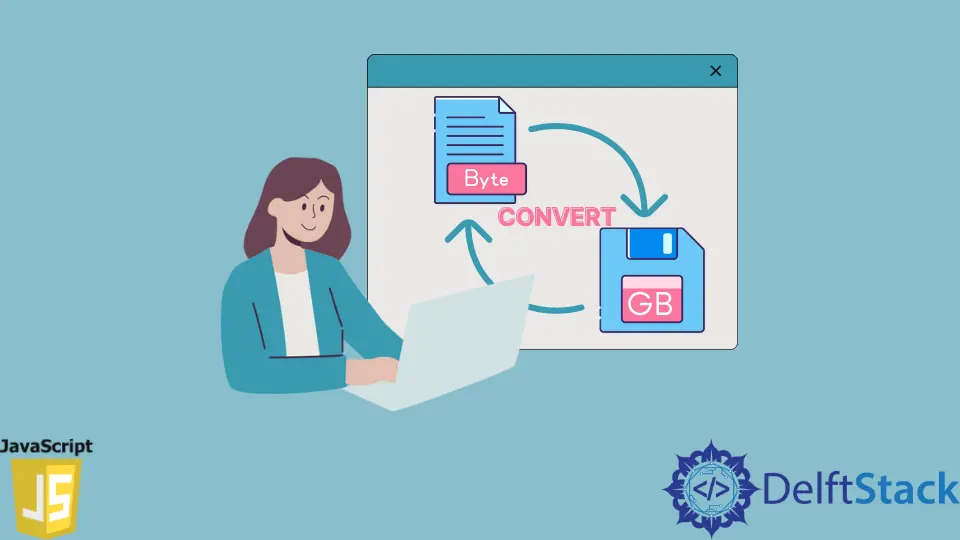
Have you ever wondered how many bytes are there in a Gigabyte? Gigabyte is a commonly used unit of measurement. Gigabyte is used widely in our day to day world file size of a movie, the capacity of a hard disc, even the size of the Random Access Memory in our laptops or mobile phones all we represent with gigabytes. All the unit in these cases used to address these are in Gigabytes. There is a bit of mathematics required for the conversion of bytes to gigabytes. It is similar to converting meters to kilometres. Let us see how we can do the conversion in JavaScript. There are a couple of approaches for the conversion of bytes to gigabytes, which are as follows.
- Converting Gigabytes to Bytes in decimal base
- Converting Gigabytes to Bytes in Binary Base
Converting to Decimal Base
The most common doubt that arises to everyone is if a kilobyte value is 1024 bytes or 1000 bytes. On searching in Google, we get mixed responses. But the underlying concept is simple. In a decimal number system, one Kilobyte value is 1000
bytes, whereas, in a binary number system, the value is 1024
bytes. Hence the conversion parameters change based on this ideology.
Rationale to Conversion With Decimal Base
Before we begin the explanation, we shall look at the key conversion units. As we are dealing with a decimal number base, we will use the following conversion rationale.
- 1 KiloByte = 1000 Byte
- 1 Megabyte = 1000 KiloByte
- 1 GigaByte = 1000 MegaByte
Hence, 1 GigaByte = 1000 * 1000 * 1000 Bytes. In other words, One GigaByte is equivalent to 10 to the power of 9 (10^9) bytes. Hence, simply searching for 1 GB
in google gives us a representation like 1e+9
. 1e+9
is equal to 1
with 9
zeroes. That is the gist of the conversion calculation.
Conversion Code
We have now understood the calculation behind the conversions and the various units of Bytes. Let us quickly go through the javascript code to convert a number (number of Bytes) given as an input. The code will return the Gigabyte equivalent value for it and display it on the screen.
<h3><center>Byte to GB</center></h3>
<div>
<center>
<input type="text" id="bytes_input"><label>Bytes</label>
</center>
</div>
<div>
<center>
<button onclick="convertToGB()">Convert</button>
<button onclick="reset()">Reset</button>
</center>
</div>
<center>
<div>
<label>Result: </label> <label id="gbValue">0</label> GB
</div>
</center>
window.onload =
function() {
reset();
updateDisplay(0);
}
function convertToGB() {
let bytesValue = document.getElementById('bytes_input').value;
let gbValue = (bytesValue / (1000 * 1000 * 1000)).toFixed(2);
updateDisplay(gbValue);
}
function reset() {
document.getElementById('bytes_input').value = 0;
updateDisplay(0);
}
function updateDisplay(val) {
document.getElementById('gbValue').innerHTML = val;
}
The steps are as follows.
-
We design an HTML GUI to capture the number of bytes from the user. The GUI has a
Convert
and aReset
button. We bind theConvert
button to theconvertToGB()
function that converts the given byte value to gigabytes. TheReset
button is bound with thereset()
function to reset the values so that the user can try newer values to convert. -
The execution of the code starts with the
window.onload()
function.window.onload()
function is the first one to get executed when the page loads. In this function, we reset the values for the input and the output Gigabyte to 0. -
Once the user enters a byte value and clicks on the
Convert
button, theconvertToGB()
function is triggered. -
In the
convertToGB()
function, we capture the value entered by the user in the byte field by using thedocument.getElementById("bytes_input").value
function of javascript and capture it in thebytesValue
variable. In the next step, we convert that byte value to gigabytes by dividing thebytesValue
by(1000 * 1000 * 1000)
. Remember, dividing the value of the byte by 1000 gives us the kilobyte value dividing it further by thousand returns the megabyte value and dividing the megabytes value gives us the gigabytes representation for the bytes. -
We do these calculations and store the result in the
gbValue
variable. In the next line, we display thegbValue
into the GUI by setting thelabel
value using thedocument.getElementById("gbValue").innerHTML
. And hence the converted value is displayed in the HTML.
Remarks
- In essence, we will require a byte having a value with more than seven digits to get a tangible Gigabyte equivalent for it. The minimum output value is
0.01 GB
, which converts to10000000 Bytes
. That means using the above code, a byte with a value greater than10000000 Bytes
will be shown in the output. The output for values lesser than that will be0 GB
. - There is a limitation of the number of digits that a variable can hold in javascript. JavaScript has a constant
Number.MAX_SAFE_INTEGER
that defines the maximum value a number variable can hold in javascript that is9007199254740991
. Hence, the code will not convert any byte number more than it to the Gigabytes representation. We may lose precision during the conversion. We do not recommend it. - According to the code we saw above, the output value shows values rounded off to two decimal places. We use the
Number.toFixed()
method of JavaScript. As per the line(bytesValue / (1000 * 1000 * 1000)).toFixed(2)
, the output will be 2 decimal places. If we show gigabyte value with better precision, we can tweak the code with.toFixed(3)
or.toFixed(4)
as per the requirement.
Converting to Binary Base
The more commonly understood ways of converting a byte value to a Gigabyte is with the binary base one. Most of us are aware of the 1024
unit value. Unlike in the decimal system, where the division is by 1000, to convert to higher byte units, in the binary number system, we divide by the 1024
factor.
Basis to Conversion With Binary Base
Before looking at the code for the conversion, let us look at the conversion unit value in the binary number system. Here the rationale will be revolving around 1024
(that is 2 to the power 10
or 2^10).
- 1 KiloByte = 1024 Byte
- 1 Megabyte = 1024 KiloByte
- 1 GigaByte = 1024 MegaByte
Hence, in the binary base system, 1 GigaByte = 1024 * 1024 * 1024 Bytes. Converting a byte number value to Gigabyte will require dividing it by 1024 * 1024 * 1024
.
Conversion Code
The following code converts the number of the bytes to a gigabytes representation in the binary system.
<h3><center>Byte to GB</center></h3>
<div>
<center>
<input type="text" id="bytes_input"><label>Bytes</label>
</center>
</div>
<div>
<center>
<button onclick="convertToGB()">Convert</button>
<button onclick="reset()">Reset</button>
</center>
</div>
<center>
<div>
<label>Result: </label> <label id="gbValue">0</label> GB
</div>
</center>
window.onload =
function() {
reset();
updateDisplay(0);
}
function convertToGB() {
let bytesValue = document.getElementById('bytes_input').value;
let gbValue = (bytesValue / (1024 * 1024 * 1024)).toFixed(2);
updateDisplay(gbValue);
}
function reset() {
document.getElementById('bytes_input').value = 0;
updateDisplay(0);
}
function updateDisplay(val) {
document.getElementById('gbValue').innerHTML = val;
}
As compared to the code with the decimal base system conversion, the only difference here is that we use the 1024
factor instead of 1000
that is evident from the line (bytesValue / (1024 * 1024 * 1024)).toFixed(2)
.
Remarks
- The same limitation applies in this method for the maximum number range that can be input. A number greater than
9007199254740991
will not guarantee a successful conversion. - We can adjust the precision with by changing the parameter of the
toFixed()
function in the line(bytesValue / (1024 * 1024 * 1024)).toFixed(2)
. Usually, in most cases, 2 or 3 digits are preferred after the decimal. - The results may differ in both conversions, the decimal base conversion and the binary base conversion. The comparison between the two methods is invalid as it will be like comparing apple and oranges. The results are in the respective number base systems. That means, in the decimal base system of converting bytes to gigabytes, the resultant will be gigabyte representation in the decimal number system and vice-versa for the binary number base system.